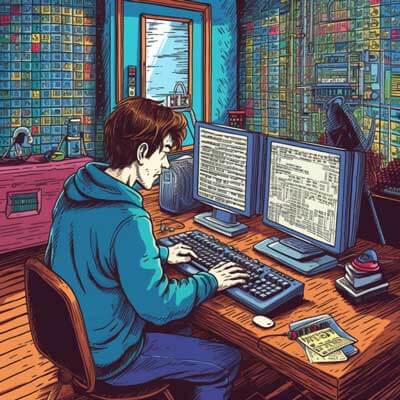
Table of Contents
How to Fix Error ECONNRESET in Node.js
Related Article: How to Fix “Npm Err! Code Elifecycle” in Node.js
Overview
The ECONNRESET error in Node.js occurs when a connection is forcibly closed by the other end without sending a proper response. This can happen due to various reasons, such as network issues, server timeouts, or misconfigured servers. This error can be frustrating to debug, but there are several steps you can take to fix it.
Possible Solutions
Related Article: How to Differentiate Between Tilde and Caret in Package.json
1. Check Network Connectivity
First, ensure that your network connection is stable and functioning properly. ECONNRESET errors can occur when there are network disruptions or intermittent connectivity issues. You can try the following steps:
- Check your internet connection and ensure that it is stable.
- Restart your router or modem to refresh the network connection.
- Temporarily disable any firewall or antivirus software that could be interfering with the network connection.
2. Retry the Connection
In some cases, the ECONNRESET error may be a temporary issue, and retrying the connection can resolve it. You can implement retry logic in your Node.js code to automatically retry failed connections. Here's an example using the axios
library:
const axios = require('axios'); async function makeRequestWithRetry(url, maxRetries = 3) { let retries = 0; while (retries < maxRetries) { try { const response = await axios.get(url); return response.data; } catch (error) { if (error.code === 'ECONNRESET') { console.log('Connection reset, retrying...'); retries++; } else { throw error; } } } throw new Error(`Failed to establish connection after ${maxRetries} retries.`); } // Usage example makeRequestWithRetry('https://api.example.com/data') .then(data => { console.log('Data:', data); }) .catch(error => { console.error('Error:', error); });
In this example, the makeRequestWithRetry
function attempts to make a GET request to the specified URL and retries the connection up to a maximum number of times (maxRetries
). If an ECONNRESET error occurs, it logs a message and retries the connection. If the maximum number of retries is exceeded, it throws an error.
3. Adjust Server Timeout Settings
If you are running a Node.js server and encountering ECONNRESET errors on the server side, adjusting the server's timeout settings may help. By default, Node.js has a server timeout of 2 minutes. You can increase or decrease this value based on your requirements. Here's an example using the Express framework:
const express = require('express'); const app = express(); // Increase server timeout to 5 minutes (300000 milliseconds) app.timeout = 300000; // Rest of your server setup and routes... app.listen(3000, () => { console.log('Server started on port 3000'); });
In this example, the server's timeout is increased to 5 minutes (300000 milliseconds) using the app.timeout
property.
4. Check Server Configuration
If you are experiencing ECONNRESET errors specifically when connecting to a certain server or service, it's possible that the server is misconfigured or has certain limitations. Check the server's documentation or contact the server administrator to ensure that the server is properly configured and can handle the expected traffic.
5. Use a HTTP Keep-Alive Mechanism
Enabling HTTP keep-alive can help prevent ECONNRESET errors by allowing persistent connections to be reused instead of establishing a new connection for each request. Most modern HTTP client libraries, including the built-in http
module in Node.js, support keep-alive by default. However, if you're using a custom HTTP client or making raw HTTP requests, you may need to manually enable keep-alive. Here's an example using the http
module:
const http = require('http'); const options = { hostname: 'api.example.com', port: 80, path: '/data', method: 'GET', headers: { Connection: 'keep-alive' } }; const req = http.request(options, res => { // Handle response... }); req.on('error', error => { console.error('Error:', error); }); req.end();
In this example, the Connection
header is set to 'keep-alive'
to enable persistent connections.