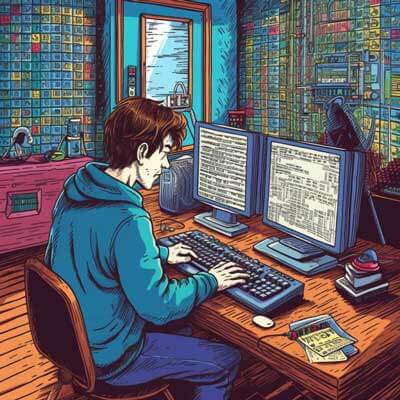
How to Fix Node.js getaddrinfo ENOTFOUND Error
If you encounter the “getaddrinfo ENOTFOUND” error in Node.js, it means that the DNS resolution failed for the given hostname. This error usually occurs when the hostname or domain name you are trying to access is invalid or cannot be resolved. Here are a few steps you can follow to fix this error:
Related Article: How To Update Node.Js
1. Check the Hostname or Domain Name
Make sure that the hostname or domain name you are trying to access is correct and valid. Double-check for any typos or misspellings in the URL or hostname. If you are using a variable to store the hostname, ensure that it is assigned the correct value.
2. Verify the Network Connectivity
Ensure that your machine has a stable internet connection and can access the network. Test your internet connection by pinging a well-known website or using other network-related tools. If you are on a restricted network or behind a firewall, make sure that you have the necessary permissions to access the desired hostname or domain.
3. Check DNS Configuration
The “getaddrinfo ENOTFOUND” error can also occur if there is an issue with your DNS (Domain Name System) configuration. DNS is responsible for resolving domain names to IP addresses. If your DNS settings are incorrect or if the DNS server is experiencing problems, it can result in this error.
To fix this issue, try the following steps:
– Restart your router or modem: Sometimes, restarting your networking equipment can resolve DNS-related issues.
– Flush DNS cache: On Linux or macOS, open a terminal window and run the following command to flush the DNS cache:
sudo /etc/init.d/nscd restart
On Windows, open the Command Prompt as an administrator and run the following command:
ipconfig /flushdns
– Change DNS server: You can try changing your DNS server to a different one. Open your network settings and manually configure your DNS server to use a public DNS service like Google DNS (8.8.8.8) or Cloudflare DNS (1.1.1.1).
Related Article: How To Check Node.Js Version On Command Line
4. Use IP Address Instead of Hostname
If you are still experiencing the “getaddrinfo ENOTFOUND” error, you can try using the IP address instead of the hostname. This bypasses the DNS resolution process and directly connects to the server using its IP address. However, keep in mind that using IP addresses may not be a long-term solution, as IP addresses can change over time.
To use the IP address instead of the hostname, replace the hostname in your code with the IP address of the server you are trying to connect to. You can usually find the IP address by pinging the hostname from your command line:
ping hostname
The IP address will be displayed in the output of the ping command. Replace the hostname in your code with this IP address.
5. Handle Errors Gracefully
When working with network requests in Node.js, it’s important to handle errors gracefully. Instead of letting the “getaddrinfo ENOTFOUND” error crash your application, you can catch the error and handle it appropriately.
Here’s an example of how you can handle the error in a Node.js application:
const http = require('http'); const options = { hostname: 'example.com', port: 80, path: '/', method: 'GET', }; const req = http.request(options, (res) => { // Handle successful response }); req.on('error', (error) => { if (error.code === 'ENOTFOUND') { console.error('Hostname not found'); // Handle the error or retry the request } else { console.error('An error occurred:', error.message); // Handle other errors } }); req.end();
In the above example, the req.on('error')
event handler catches the “getaddrinfo ENOTFOUND” error and provides a custom error message. You can handle the error according to your application’s requirements, whether it’s displaying an error message to the user, retrying the request, or logging the error for further investigation.
6. Use a Library or Module
If you frequently encounter DNS-related errors in your Node.js application, you may consider using a library or module that abstracts the network requests and provides error handling mechanisms. Libraries like axios
, request
, or got
can simplify the process of making HTTP requests and handle errors more effectively.
Here’s an example using the axios
library:
const axios = require('axios'); axios.get('http://example.com') .then((response) => { // Handle successful response }) .catch((error) => { if (error.code === 'ENOTFOUND') { console.error('Hostname not found'); // Handle the error or retry the request } else { console.error('An error occurred:', error.message); // Handle other errors } });
Related Article: How To Upgrade Node.js To The Latest Version
7. Consult the Node.js Documentation and Community
If none of the above steps resolve the “getaddrinfo ENOTFOUND” error, it can be helpful to consult the official Node.js documentation or seek assistance from the Node.js community. The Node.js documentation provides detailed information about network-related modules and functions, which can help you understand and troubleshoot the error.
You can also search for similar issues on popular developer forums, Q&A websites, or the Node.js GitHub repository. Often, other developers have encountered similar problems and may have shared their solutions or workarounds.