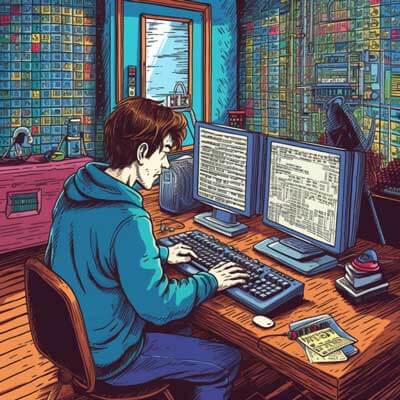
Table of Contents
npm and Node.js
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. It allows developers to run JavaScript on the server side, enabling the building of scalable network applications. npm, or Node Package Manager, comes bundled with Node.js. It is a package manager for JavaScript and helps manage libraries and tools that developers need for their projects. When you install Node.js, npm is installed automatically, allowing you to install, share, and manage dependencies for your JavaScript applications.
Related Article: How to Fix npm Error Could Not Determine Executable
What Does 'npm is Not Recognized' Mean
The error message 'npm is not recognized as an internal or external command' typically indicates that the command line interface (CLI) cannot find the npm executable. This situation usually arises due to issues with the installation or configuration of Node.js and npm. When the system does not know where to look for npm, it cannot execute the commands you enter. This can occur on various operating systems, including Windows, macOS, and Linux.
Checking npm Installation
To determine whether npm is installed, you can run a simple command in your command line interface. Open your terminal or command prompt and type:
npm -v
This command checks the version of npm installed. If npm is installed correctly, it will return a version number. If you see the 'not recognized' error, it indicates that npm is either not installed or not properly configured in your system.
Installing Node.js and npm
If npm is not installed, the next step is to install Node.js, which will include npm. Visit the official Node.js website at https://nodejs.org. You will find two versions available: LTS (Long Term Support) and Current. For most users, the LTS version is recommended due to its stability. Download the installer for your operating system and follow the installation instructions.
During the installation process, ensure that you select the option to install npm alongside Node.js. This is usually checked by default.
Related Article: How To Set Npm Registry Configuration
Verifying Command Line Access
After installation, it is essential to verify that both Node.js and npm are accessible from the command line. You can do this by running the following commands:
node -vnpm -v
Both commands should return version numbers. If you receive the 'not recognized' error for either command, there may be an issue with your installation or your system's PATH configuration.
Setting Up Environment Variables
Environment variables are system-wide parameters that help applications locate necessary resources. For npm to be recognized in your command line, it must be included in the system's PATH environment variable. The PATH variable tells the operating system where to look for executable files.
To check your PATH variable on Windows, open the command prompt and enter:
echo %PATH%
This command displays the PATH variable's contents. You should see a path that leads to the npm directory, typically something like C:\Program Files\nodejs\
.
Modifying the PATH Variable
If the npm path is not present in your PATH variable, you will need to add it manually. Here is how to do this on Windows:
1. Right-click on 'This PC' or 'My Computer' and select 'Properties.'
2. Click on 'Advanced system settings.'
3. In the System Properties window, click on the 'Environment Variables' button.
4. In the Environment Variables window, find the 'Path' variable in the 'System variables' section and select it. Click 'Edit.'
5. In the Edit Environment Variable window, click 'New' and add the path to the Node.js installation directory, typically C:\Program Files\nodejs\
.
6. Click 'OK' to close all windows.
After modifying the PATH variable, restart your command prompt and check if npm is now recognized.
Using npm in Command Prompt
Once npm is set up correctly, you can use it directly in the command prompt. This allows you to install packages globally or locally within your project. Here is an example of installing a package globally:
npm install -g <package-name>
Replace <package-name>
with the name of the package you wish to install. For instance, to install the popular package express, you would run:
npm install -g express
This command installs the express package globally, making it accessible from any project on your machine.
Related Article: How to Fix npm Self Signed Certificate Error
Using npm in PowerShell
PowerShell can also be used to run npm commands. The process is similar to using the command prompt. Open PowerShell and enter your npm commands. If you have followed the previous steps, npm should work seamlessly. For example, you can check the version of npm in PowerShell by running:
npm -v
If npm commands do not work in PowerShell, ensure that the PATH variable is correctly set and that PowerShell is restarted after making any changes.
Global Installation of npm Packages
Installing npm packages globally allows you to use tools and libraries from anywhere on your system. Some commonly used global packages include npm, nodemon, and typescript. To install a package globally, use the -g
flag as shown previously.
For example, to install nodemon, which is a tool that automatically restarts your Node.js application when file changes are detected, run:
npm install -g nodemon
This will allow you to run nodemon from any directory within your command line interface.
Common npm Errors After Installation
Even after installation, users may encounter various npm-related errors. Common issues include permission errors, especially on Unix-based systems, where you might need to use sudo
for global installations:
sudo npm install -g <package-name>
Another frequent error is the EACCES
permission denied issue. This can often be resolved by changing the ownership of the npm directory. You can change the owner of the npm directory with the following command:
sudo chown -R $(whoami) $(npm config get prefix)/{lib/node_modules,bin,share}
This command allows your user to have the necessary permissions to install packages globally.
Reinstalling Node.js and npm
In some cases, reinstalling Node.js and npm may be the simplest solution to persistent issues. First, uninstall Node.js from your system through the Control Panel on Windows or using a package manager on macOS or Linux. After uninstalling, download the latest version from the Node.js website and run the installer, ensuring that npm is included.
After reinstallation, verify the installation again with:
node -vnpm -v
Both commands should return version numbers without errors.
Related Article: How To Get Module Version In Npm
Where to Install npm on Your System
The default installation path for Node.js and npm varies by operating system. On Windows, they are usually installed in C:\Program Files\nodejs\
. On macOS, they may be installed in /usr/local/bin
. For Linux, the installation path can vary depending on the package manager used, but it is often found in /usr/bin/
.
When installing packages, npm will install them in a node_modules
directory within your project folder by default. If you install packages globally, they will be placed in the global node_modules
directory, which is determined by the npm configuration.
Troubleshooting npm PATH Issues
If you continue to experience issues with npm not being recognized, consider the following troubleshooting steps:
1. Ensure Node.js and npm are installed by checking their versions.
2. Verify that the installation path is included in the system's PATH variable.
3. Restart your command prompt or PowerShell after making any changes to the PATH variable.
4. Run the command prompt or PowerShell as an administrator to avoid permission issues.
5. Check for conflicts with other software that may also use npm or Node.js.