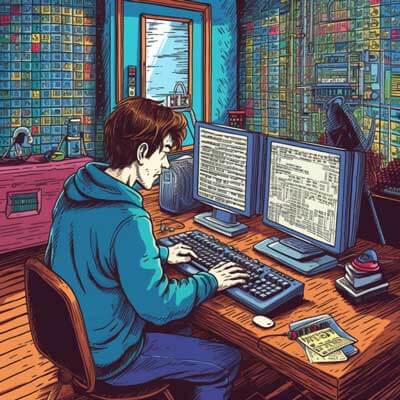
Table of Contents
Overview of npm Start Issues
npm is a package manager for JavaScript that allows developers to share and manage code efficiently. One common command developers use is npm start
, which is intended to run the start script defined in the package.json
file. However, issues can arise when executing this command. Problems can range from misconfiguration in the package.json
file to environmental conflicts. Understanding these issues is crucial for troubleshooting and ensuring a smooth development experience.
Related Article: How to Fix Communication with the API in NPM
What npm Start Does
The npm start
command serves as a shortcut to execute the start script in your package.json
file. If no start script is defined, npm will attempt to run node server.js
by default. The primary purpose of this command is to initiate the application, whether it be a web server, a build process, or any other task defined within the script. The start script can be customized to execute any command, thereby providing flexibility in how an application is launched.
For example, a typical package.json
might look like this:
{ "name": "my-app", "version": "1.0.0", "scripts": { "start": "node app.js" } }
In this case, running npm start
will execute node app.js
, starting the application as intended.
Common Errors with npm Start
Errors when running npm start
can manifest in various forms. Common issues include missing scripts, incorrect paths, and runtime errors in the application code. Users might encounter messages like:
- npm ERR! missing script: start
- Error: Cannot find module 'express'
- Error: listen EADDRINUSE: address already in use
Each of these errors points to a specific problem that requires a different approach to resolve. Addressing these errors usually involves checking the package.json
file, ensuring all dependencies are installed, and verifying that the application code is functioning correctly.
Checking Your npm Installation
Before troubleshooting npm start
issues, it's essential to ensure that npm is correctly installed and functioning. To check the npm installation, execute the following commands in your terminal:
npm -v node -v
These commands will return the versions of npm and Node.js installed on your system. If you encounter errors, the installation may be corrupted, or the paths may not be set correctly. Reinstalling Node.js usually resolves these issues since npm comes bundled with it.
Related Article: How to Use npm for Package Management
Troubleshooting npm Start Problems
When faced with problems running npm start
, a systematic approach to troubleshooting is typically the most effective. Start by reviewing the command output for any specific error messages. These messages often provide clues about what went wrong. After this, the following steps can be taken:
1. Check Your Package.json: Ensure that the start script is defined correctly.
2. Run npm install: This command ensures all dependencies are installed as specified in package.json
.
3. Check Node.js and npm Versions: Compatibility issues can arise if you are using outdated versions.
If the problem persists, consider running the application directly without npm to see if the issue lies within npm itself or the application code.
Checking package.json for Errors
Errors in the package.json
file can lead to the failure of npm start
. Open the package.json
and ensure that the structure is valid JSON. Look for common syntax errors, such as missing commas or quotation marks. Additionally, verify that the start script is properly defined. For example, a faulty package.json
may look like this:
{ "name": "my-app", "version": "1.0.0", "scripts": { "start": "node app.js" "test": "jest" } }
In this case, a comma is missing after the start script, which would cause npm to fail. Correcting it to:
{ "name": "my-app", "version": "1.0.0", "scripts": { "start": "node app.js", "test": "jest" } }
will resolve the issue.
Missing Dependencies and npm Start
Missing dependencies can prevent npm start
from running successfully. If the application relies on external packages, they must be installed for the application to function. To check for missing dependencies, look for error messages that indicate a module could not be found. For instance:
Error: Cannot find module 'express'
This error indicates that the application is trying to use the Express package, which is not installed. To resolve this, run:
npm install express
This command will install the missing package. After ensuring all dependencies are installed, rerun npm start
to see if the issue is resolved.
Node Version Compatibility
Node.js versions can affect how applications behave and whether they start successfully. Certain packages may require specific Node.js versions. To check your currently installed Node.js version, use:
node -v
If a specific version is required, consider using a version manager such as nvm (Node Version Manager) to switch between Node.js versions. To install a specific version using nvm, run:
nvm install 14 nvm use 14
Replace 14
with the desired version number. After switching versions, check if npm start
works properly.
Related Article: How To Run Tests For A Specific File With Npm
Environment Variables and npm Start
Environment variables can influence how npm start
executes. Applications often rely on environment variables for configuration settings such as API keys, database connections, and other sensitive information. If these variables are not set correctly, it can lead to application failures.
To check environment variables in your terminal, use:
printenv
or on Windows:
set
Ensure that any required environment variables are set before running npm start
. If the application uses a .env file for configuration, ensure it is present in the root directory and properly formatted.
Resolving Installation Issues
If issues with npm start
persist, installation problems may be the cause. This can occur due to corrupted node_modules
or a faulty package-lock.json
file. To resolve this, delete the node_modules
directory and the package-lock.json
file:
rm -rf node_modules package-lock.json
After removing these files, reinstall all dependencies by running:
npm install
This action will create a fresh node_modules
directory and regenerate the package-lock.json
file. After this process is complete, try running npm start
again.
Handling Unresponsive npm Start
Sometimes, the npm start
command may execute without errors but not respond as expected. This situation might occur if the application is running but not producing any visible output or is crashing silently. To diagnose this, consider adding console logs throughout the application code to identify where it may be failing.
Using tools like nodemon can also help. Nodemon automatically restarts the application when file changes are detected, providing immediate feedback. To install nodemon, run:
npm install -g nodemon
Then, modify the start script in package.json
:
"scripts": { "start": "nodemon app.js" }
This setup will help in identifying issues as they occur.