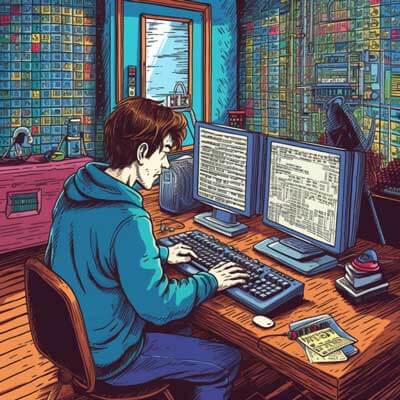
Table of Contents
Overview of Unsupported Engine Warnings
Unsupported engine warnings are common in the Node.js ecosystem when using npm (Node Package Manager). These warnings occur when the specified version of Node.js in your environment does not match the version required by a package you are trying to install or run. Each package can define its compatibility through the engines
field in its package.json
file, which specifies the versions of Node.js that are supported. When npm detects a mismatch, it raises a warning to alert users of potential compatibility issues.
The purpose of these warnings is to inform developers that the package may not work as intended due to the version of Node.js they are using. Addressing these warnings is crucial for maintaining stability and functionality in applications.
Related Article: How to Fix Jupyter Not a Valid NPM Package Error
What Does the npm Warn Ebadengine Message Mean
The npm warn Ebadengine
message is encountered when npm determines that the engine specified in a package's package.json
file does not match the current version of Node.js installed on your machine. This warning typically indicates that the package may not function correctly or may have unexpected behavior due to the version discrepancy.
For instance, if a package specifies it requires Node.js version 14.x, but your environment is running Node.js version 12.x, you will receive this warning. It's important to note that while npm allows you to install the package despite the warning, running it might lead to unforeseen issues down the line.
Causes of the Unsupported Engine Warning
Several factors can lead to the Ebadengine
warning. One primary cause is when the package's package.json
explicitly defines an engine version that your current Node.js installation does not meet.
Another cause may stem from using libraries that depend on specific Node.js features or APIs available only in certain versions. For example, if a package uses ECMAScript features introduced in Node.js 14 but is run on an older version, it will likely generate compatibility issues.
Additionally, if the package has not been updated to support newer Node.js versions, it may still declare compatibility with older versions, leading to confusion and warnings.
Specifying Engine Version in package.json
To avoid the Ebadengine
warning, packages can specify compatible Node.js versions in their package.json
file using the engines
field. This field can take a version range or a specific version.
Here is an example of how to add this to a package.json
file:
{ "name": "example-package", "version": "1.0.0", "engines": { "node": ">=14.0.0 <15.0.0" }}
In this example, the package is declaring that it is compatible with Node.js versions 14.x but not with any version 15.x or above. When using this feature, it is essential to keep the declared version ranges up to date to avoid compatibility issues for users.
Related Article: How to Fix npm Start Not Working Issue
Checking Current Node.js Version
To resolve the Ebadengine
warning, first check the Node.js version currently installed on your system. This can be done using the command line with the following command:
node -v
This command will output the version of Node.js you have installed. For example, if it returns v12.18.3
, then you are running Node.js version 12.18.3. Knowing your current version helps you determine if you need to update Node.js to meet the requirements of the packages you are working with.
Supported Node.js Versions for Packages
Each package may declare different supported Node.js versions. It is essential to check the engines
field in the package.json
of the package you are trying to use. Many popular packages maintain a compatibility table in their documentation, which outlines the supported versions.
For example, if a package documentation states that it supports Node.js versions 14.x and 16.x, but you are running version 12.x, you will need to update your Node.js installation to ensure proper functionality.
Resolving the Unsupported Engine Warning
To address the Ebadengine
warning, you have a few options. The most straightforward solution is to update your Node.js installation to a version that aligns with the requirements specified in the package’s engines
field.
Updating Node.js can be done using Node Version Manager (nvm) or directly downloading from the official Node.js website. If using nvm, the following commands can be utilized:
nvm install 14nvm use 14
This installs and switches to Node.js version 14, addressing the warning if that version is compatible with the packages you are using.
If you cannot update your Node.js version, consider finding an alternative package that is compatible with your current version or reach out to the package maintainers for support.
Handling Peer Dependencies
Peer dependencies can also contribute to engine warnings. These are packages that are required by a dependency but are expected to be installed in the parent project. If these peer dependencies specify an incompatible version of Node.js, you may encounter the Ebadengine
warning.
To handle peer dependencies effectively, check the peer dependency requirements when installing packages. You can view the peer dependencies of a package by running:
npm info <package-name> peerDependencies
Replace <package-name>
with the name of the package you are inspecting. This command will return the required versions of peer dependencies, allowing you to ensure that your project meets all necessary requirements.
Related Article: How To Detect Programming Language In Npm Code
Ignoring the Ebadengine Warning
In some cases, you may choose to ignore the Ebadengine
warning if you believe the package will still function correctly despite the version mismatch. However, it is crucial to proceed with caution, as ignoring these warnings can lead to unexpected behavior in your application.
If you decide to ignore the warning, ensure that you thoroughly test your application to confirm that everything works as expected. This includes running unit tests, integration tests, and manual testing to identify any issues that may arise from the version incompatibility.
Suppressing npm Warnings
Sometimes, suppressing warnings can be necessary, especially in continuous integration environments where you want clean logs. To suppress specific npm warnings, you can set the loglevel
to silent
or error
in your npm command.
For example, you can run:
npm install --loglevel=error
This command will prevent warnings from appearing in your terminal output. Although this does not resolve the underlying issues, it can help maintain cleaner output in automated processes.
Implications of Ebadengine Warnings
Ignoring or suppressing Ebadengine
warnings can have significant implications for your project. If the warning is not addressed, you might encounter runtime errors, unexpected behavior, or even security vulnerabilities if the incompatible package relies on deprecated features.
It is essential to consider the potential risks before deciding to ignore these warnings. Always ensure that the core functionality of your application is not compromised and that you are aware of any limitations that may arise from using packages with unsupported engine warnings.
Addressing Node.js Version Mismatches
To address Node.js version mismatches effectively, start by reviewing the engines
field in the package.json
files of your dependencies. This will provide insight into what versions are compatible. If necessary, update your Node.js installation to the required version or consider using nvm to manage multiple versions of Node.js easily.
Additionally, regularly check for updates to both your Node.js installation and your project dependencies. Keeping everything up to date minimizes compatibility issues and ensures that you benefit from the latest features and security patches.