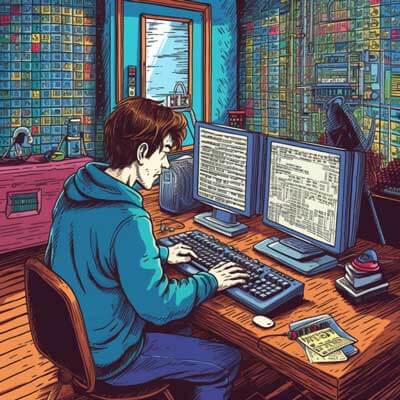
Table of Contents
The "Unhandled Exception Cannot Find Module" error occurs in Webpack when it attempts to require or import a module that does not exist or cannot be found. This can disrupt the build process, leading to failures in running the application. The error message typically looks like this:
Error: Cannot find module 'module-name'
This indicates that Webpack was unable to locate the specified module, which could be due to various reasons, including incorrect paths, missing files, or misconfigured settings. Understanding the root causes and how to resolve them is crucial for maintaining a smooth development workflow.
What is a Module in a Build Tool
Modules are self-contained units of code that can be reused throughout an application. In the context of build tools like Webpack, a module can represent a JavaScript file, a CSS file, an image, or any other resource that can be imported into the application. Webpack treats each file as a module and bundles them together, optimizing the output for performance.
Modules allow for better organization of code, making it easier to manage dependencies and maintain the application. For instance, if you have a file named utils.js
that contains utility functions, you can import it into other files as follows:
// example.jsimport { myFunction } from './utils';
This modular approach helps in keeping code clean and manageable.
Related Article: How to Use Webpack Tree Shaking for Smaller Bundles
Common Causes of Cannot Find Module Error
Several factors contribute to the "Cannot Find Module" error. Some of the most frequent causes include:
- Incorrect File Paths: If the path specified in the import statement is incorrect or misspelled, Webpack will not be able to find the module.
- Missing Modules: If a module has not been installed or is not listed in the package.json
, Webpack will raise this error.
- Case Sensitivity: File systems, especially on Linux, are case-sensitive. An import that uses the wrong casing will lead to this error.
- Incorrect Configuration: Improper Webpack configuration, especially related to module resolution, can also result in this error.
- Version Mismatches: If using different versions of a module, compatibility issues may arise that prevent modules from being found.
Recognizing these common causes can help in troubleshooting the issue more efficiently.
How to Fix the Cannot Find Module Error
Resolving the "Cannot Find Module" error involves a systematic approach to identify and address the underlying issues. Here are the steps to take:
1. Check Import Statements: Ensure that all import statements use the correct file paths. Look for typos or incorrect casing.
// Incorrect import import { myFunction } from './utils'; // Ensure path is correct
2. Verify Module Installation: Confirm that the required module is installed. You can do this by checking the node_modules
directory or using the npm list
command.
npm list module-name
3. Inspect package.json
: Make sure the module is listed under dependencies or devDependencies in your package.json
. If it’s missing, you need to install it.
4. Check Webpack Configuration: Review your Webpack configuration file (usually named webpack.config.js
) for any misconfigurations regarding module resolution.
5. Use Correct File Extensions: Make sure to include the correct file extensions in import statements if your configuration does not automatically resolve them.
6. Reinstall Node Modules: Sometimes, reinstalling all node modules can help resolve issues. You can do this by deleting the node_modules
folder and running:
npm install
Following these steps will often resolve the error.
Configuring Module Resolution
Configuring module resolution in Webpack allows you to specify how modules are found and imported into your application. You can define certain settings in your webpack.config.js
file to make this process smoother.
// webpack.config.jsconst path = require('path');module.exports = { resolve: { extensions: ['.js', '.jsx', '.json'], // Automatically resolve these extensions alias: { Utils: path.resolve(__dirname, 'src/utils/'), // Create an alias for the utils directory }, },};
In this configuration:
- The extensions
array tells Webpack to automatically resolve modules with these extensions, so you can import them without specifying the file extension.
- The alias
option allows you to create shortcuts for paths, which can simplify imports throughout your codebase.
Correctly configuring module resolution can prevent many "Cannot Find Module" errors.
Related Article: How to Use webpack -d for Development Mode
package.json in Dependency Management
The package.json
file is central to dependency management in Node.js projects, including those using Webpack. It lists all the dependencies required for the application to run, along with their versions.
When you install a package using npm, it updates the package.json
file to include the new dependency. Here is an example of how a package.json
file might look:
{ "name": "my-app", "version": "1.0.0", "dependencies": { "react": "^17.0.2", "webpack": "^5.0.0" }, "devDependencies": { "webpack-cli": "^4.0.0" }}
In this example:
- dependencies
are packages needed for the application to run.
- devDependencies
are packages needed only during development, such as testing libraries or build tools.
To install a new dependency, you can run:
npm install module-name
If you want to save it as a development dependency, use:
npm install --save-dev module-name
Managing dependencies through package.json
helps ensure that your project remains organized and that others can easily reproduce your development environment.
Checking Installed Modules
To check which modules are installed in your project, you can use the following command:
npm list --depth=0
This command lists all top-level dependencies in your project without displaying their sub-dependencies. The output will look something like this:
my-app@1.0.0 /path/to/my-app├── react@17.0.2├── webpack@5.0.0└── webpack-cli@4.0.0
If you are looking for a specific module, you can specify its name:
npm list module-name
This will confirm whether the module is installed and what version is in use.
Finding the Version of the Build Tool in Use
To find out which version of Webpack is installed in your project, you can use the following command:
npx webpack --version
This will output the installed version of Webpack. If you need to check the version of other packages, you can also look directly in the package.json
file or use the following command:
npm list webpack
This will show the version of Webpack being used along with its path in the node_modules
directory.
Installing Missing Modules
If a required module is missing, you can install it using npm. The command to do so is:
npm install module-name
For instance, if you need to install the lodash
library, you would run:
npm install lodash
This adds lodash
to your node_modules
and updates the package.json
file accordingly. If you want to install it as a development dependency, use:
npm install --save-dev module-name
This is useful for modules that are only necessary during development, such as linters or testing libraries.
Related Article: How to Configure SVGR with Webpack
Difference Between ES Modules and CommonJS
ES Modules (ECMAScript Modules) and CommonJS are two module systems in JavaScript.
- CommonJS is primarily used in Node.js. It uses require()
to import modules and module.exports
to export them. Here's an example:
// CommonJS exampleconst myFunction = require('./myFunction');module.exports = myFunction;
- ES Modules use the import
and export
syntax. They are part of the ECMAScript specification and are now widely supported in modern browsers and Node.js. Example:
// ES Module exampleexport const myFunction = () => { /* ... */ };import { myFunction } from './myFunction.js';
The choice between them often depends on the project setup and whether you are targeting a Node.js environment or the browser. Webpack supports both systems, allowing developers to adopt the module format that best fits their needs.
Troubleshooting Build Issues
When encountering build issues in Webpack, here are steps to diagnose and resolve them:
1. Check the Console Output: Webpack provides detailed error messages in the terminal. Carefully read the output to identify the source of the problem.
2. Run Webpack in Watch Mode: Running Webpack in watch mode can help you see changes in real-time and quickly identify what breaks the build.
npx webpack --watch
3. Use Source Maps: Enabling source maps can make debugging easier by mapping the minified code back to the original source.
// webpack.config.js module.exports = { devtool: 'source-map', };
4. Check Configuration Files: Review webpack.config.js
for any misconfigurations. Ensure all loaders and plugins are correctly set up.
5. Clear Cache: Sometimes, clearing the Webpack cache can resolve unexplained issues. You can delete the node_modules/.cache
directory.
6. Search for Similar Issues: Look for similar issues on platforms like GitHub, StackOverflow, or the Webpack documentation. Often, other developers have encountered and resolved similar problems.
Systematic troubleshooting can help pinpoint and address build issues efficiently.
Error Handling Techniques in Node.js
Error handling is critical in Node.js applications. Common techniques include:
- Try-Catch Blocks: Wrap code that may throw an error in a try-catch block to handle exceptions gracefully.
try { // Code that may throw an error} catch (error) { console.error('An error occurred:', error);}
- Promise Rejection Handling: Always handle promise rejections to prevent unhandled promise rejections.
myPromise .then(result => { /* handle success */ }) .catch(error => { console.error('Promise rejected:', error); });
- Using Async/Await: With async/await, you can use try-catch to handle errors in asynchronous code.
async function myAsyncFunction() { try { const result = await someAsyncCall(); } catch (error) { console.error('Error in async function:', error); }}
- Global Error Handlers: For catching unhandled errors globally, you can use process-level event handlers.
process.on('uncaughtException', (error) => { console.error('Unhandled Exception:', error);});
Implementing robust error handling techniques ensures that your application can handle unexpected scenarios and improve stability.
Setting Up a Development Server
Setting up a development server in Webpack allows for live reloading and easier debugging. Webpack Dev Server is commonly used for this purpose.
To set it up, install it using npm:
npm install --save-dev webpack-dev-server
Next, add a script to your package.json
to start the server:
"scripts": { "start": "webpack serve --open"}
Now, create a minimal webpack.config.js
for the development server:
const path = require('path');module.exports = { mode: 'development', entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, },};
With this configuration, you can start the server by running:
npm start
The server will serve your application at http://localhost:9000
, and any changes made to your source files will trigger a reload in the browser. This setup enhances the development experience, making it easier to test and debug your application in real-time.
Related Article: How to generate source maps for ESM in Webpack
Additional Resources
- Webpack Module Documentation