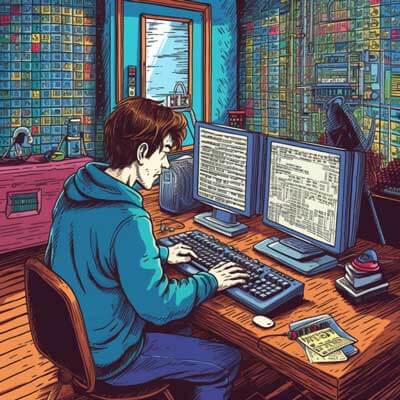
Table of Contents
Overview
The "Command Not Found" error typically occurs when the terminal cannot find the command you are trying to execute. This often happens if the software is not installed or if the command is not accessible in your system's PATH. For instance, if you try to run webpack
in your terminal and see this error, it means that the system cannot find the Webpack binary.
Related Article: How to Use the Fork TS Checker Webpack Plugin
Steps for Correct Installation Using npm
To install Webpack correctly using npm, follow these simple steps:
1. Open your terminal.
2. Navigate to your project directory using the cd
command. For example:
cd my-project
3. Run the following command to install Webpack and Webpack CLI:
npm install --save-dev webpack webpack-cli
This command installs Webpack as a development dependency in your project, ensuring it is available for your project's build processes.
Node modules are packages that contain reusable code. When you install a package using npm, it is stored in the node_modules
directory within your project. Webpack, being a Node.js package, resides in this directory after installation. This modular approach allows you to use various libraries and tools without cluttering your project directory with all their code.
Global Installation Versus Local Installation
Global installation means you install the package system-wide, making it accessible from any project. Local installation, on the other hand, installs the package only within the project’s directory.
To install Webpack globally, use:
npm install -g webpack webpack-cli
Local installation is generally preferred for project-specific dependencies, while global installation is useful when you want to use the tool across multiple projects.
How to Verify if the Tool is Installed
After installation, verifying if Webpack is installed correctly is essential. Run the following command in your terminal:
npx webpack --version
If Webpack is installed, this command will return the version number. If you see an error instead, the installation did not succeed.
Related Article: How To Exclude Test Files In Webpack Builds
Running the Tool from the Command Line
You can run Webpack directly from the command line. If you installed it locally, use:
npx webpack
This command executes Webpack from the node_modules
directory. If you installed it globally, you can simply type:
webpack
This will run Webpack from anywhere in your system.
Configuring the Tool for Your Project
To configure Webpack for your project, create a file named webpack.config.js
in your project root. This file will hold the configuration settings for your Webpack build process. A basic configuration might look like this:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, mode: 'development' };
This example specifies an entry point and an output file for the bundled code.
Adding the Tool to Your Project Setup
To add Webpack to your project setup, ensure that you have the necessary scripts in your package.json
. Add a build script like this:
{ "scripts": { "build": "webpack" } }
This allows you to run Webpack with a simple command.
Fixing Common Errors Encountered
Common errors when using Webpack include misconfigured paths, missing loaders, and syntax errors in your configuration file. If you encounter an error, check the terminal output for clues. Ensure that paths in your webpack.config.js
are correct and that any loaders required for processing file types (like CSS, images, etc.) are installed.
For example, if you want to load CSS files, you need to install the appropriate loader:
npm install --save-dev style-loader css-loader
Then, update your configuration to include these loaders.
Related Article: How to Set Up Webpack Proxy for Development
Setting Up npm Scripts for Convenience
Using npm scripts can make running Webpack easier. In your package.json
, you can set up multiple scripts:
{ "scripts": { "build": "webpack", "watch": "webpack --watch", "dev": "webpack-dev-server" } }
Now, you can run npm run build
to build your project, npm run watch
to watch for changes, and npm run dev
to start a development server.
Using the Development Server
Webpack comes with a development server called webpack-dev-server
. This tool serves your app in a local server environment, providing live reloading as you make changes. To set it up, first, install it:
npm install --save-dev webpack-dev-server
Then, configure it in your webpack.config.js
:
devServer: { contentBase: './dist', },
You can start the server using the npm script you added earlier. This setup allows you to see your changes in real-time without manually refreshing the browser.
Managing Build Processes
Managing build processes involves organizing your build scripts and optimizing the configuration. Use the mode
option in webpack.config.js
to switch between development and production builds:
mode: 'production'
In production mode, Webpack optimizes the output, reducing file size and improving load times. For development, you might want source maps enabled for easier debugging:
devtool: 'source-map'
These options help streamline your build process according to your project's needs.
Installing Without npm as an Option
While npm is the most common package manager for JavaScript, it is possible to install Webpack using Yarn, another package manager. To install Webpack with Yarn, run:
yarn add --dev webpack webpack-cli
This command functions similarly to the npm install command, adding Webpack to your project.
Related Article: How To Fix Unhandled Exception Cannot Find Module Webpack
The Configuration File
The webpack.config.js
file is crucial as it defines how Webpack processes your code. Key properties include:
- entry: The entry point of your application, where Webpack starts building the dependency graph.
- output: This specifies the output file and directory for the bundled code.
- loaders: Use loaders to preprocess files, such as converting Sass to CSS or transpiling ES6 to ES5.
- plugins: These can perform a wider range of tasks, like optimizing the build or cleaning the output directory before building.
A more advanced configuration might look like this:
const path = require('path'); const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader' } }, { test: /\.css$/, use: ['style-loader', 'css-loader'] } ] }, plugins: [ new HtmlWebpackPlugin({ template: './src/index.html' }) ], mode: 'development' };
This example demonstrates how to use loaders and plugins for a more functional build setup.
Locating the Tool in Your System
If you need to locate Webpack after installation, you can use the following command to find its path:
npm list -g webpack
For local installations, check the node_modules/.bin
directory within your project. This directory contains executable files for your installed packages. You can also use:
npx webpack --info
This command provides information about the installed Webpack version and its configuration.
Additional Resources
- Webpack Installation Guide