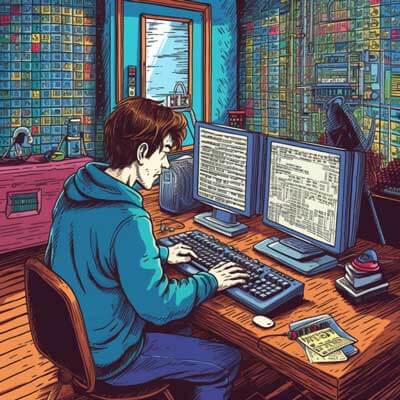
Table of Contents
Overview
The "webpack not recognized as a command" error occurs when the system cannot locate the webpack executable in your command line interface. This can halt your development workflow, as Webpack is a popular module bundler used to compile JavaScript applications. Without proper recognition of the command, you cannot leverage Webpack's functionalities for tasks like optimizing assets, transforming code, or managing dependencies.
There are two primary methods for installing Webpack: local and global. A local installation means adding Webpack to a specific project, allowing for project-specific configurations and dependencies. In contrast, a global installation makes the command available system-wide, enabling usage across multiple projects without needing to install it each time.
Related Article: How to generate source maps for ESM in Webpack
Step-by-step guide to global installation using npm
To install Webpack globally using npm, follow these steps:
1. Open your command line interface (CLI).
2. Run the following command:
npm install -g webpack webpack-cli
This command installs both Webpack and its command-line interface globally. The -g
flag indicates that the package should be installed globally.
Instructions for local installation within a project
To install Webpack locally within a project, execute the following steps:
1. Navigate to your project directory using the command line:
cd your-project-directory
2. Run the local installation command:
npm install --save-dev webpack webpack-cli
The --save-dev
flag indicates that these packages are development dependencies, which means they are only needed during development and not in production.
Common reasons for the command not being recognized
Several factors can cause the "not recognized" error:
1. Webpack not installed: The command will not work if Webpack is not installed globally or locally in your project.
2. Incorrect PATH: The system PATH variable may not include the directory where Webpack is installed.
3. Terminal or shell issues: Sometimes, terminal configurations or issues can prevent commands from being recognized.
4. Version conflicts: If there are conflicting versions of Webpack installed, it may lead to command recognition problems.
Related Article: How to Fix Angular Webpack Plugin Initialization Error
Verifying correct installation and setup
To verify if Webpack is correctly installed, run the following command:
webpack --version
If installed correctly, this command will return the installed version of Webpack. If not, it will indicate that the command is still not recognized.
Checking the version of the module in use
After installation, checking the version of Webpack is essential for ensuring compatibility with your project. Use the following command:
npm list webpack
This command displays the version of Webpack that is currently installed in your project. If you want to check the global version, use:
npm list -g webpack
Setting up the PATH variable for command line access
In cases where Webpack is installed but not recognized, the PATH variable may need adjustment. The PATH variable tells the operating system where to look for executables. To set it up:
1. Determine where npm installs global packages. You can find this by running:
npm config get prefix
2. Add the bin
directory to your PATH. For example, if the output is /usr/local
, add /usr/local/bin
to your PATH.
On Windows, you can set the PATH variable by:
- Right-clicking on "This PC" or "My Computer" and selecting "Properties".
- Clicking on "Advanced system settings".
- Going to the "Environment Variables" section.
- Under "System variables", find the "Path" variable, select it, and click "Edit".
- Add the path to the npm global bin directory.
How environment variables influence command recognition
Environment variables play a crucial role in how commands are recognized by the command line. If the directory containing Webpack is not included in the PATH variable, the command line will not be able to find it, resulting in the error. Adjusting the PATH allows the command line to locate the Webpack executable when you try to run it.
Related Article: How to Configure Electron with Webpack
Adding the necessary module to your project
To ensure that Webpack functions correctly in your project, you need to include necessary configurations and modules. After installing Webpack locally, create a webpack.config.js
file in your project root with the following basic setup:
// webpack.config.js const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') } };
This configuration specifies that Webpack should take src/index.js
as the entry point and output bundle.js
in the dist
directory.
Troubleshooting terminal issues related to command not found
If the command is still not recognized after installation, check the following:
1. Ensure that you are in the correct terminal. Sometimes, using different terminals (e.g., PowerShell, Command Prompt, or Terminal on macOS) can yield different results.
2. Restart the terminal after making changes to the PATH variable.
3. Check if there are any aliases or functions defined in your shell configuration files (like .bashrc
, .bash_profile
, or .zshrc
) that might conflict with the Webpack command.
Using alternatives to npm for installation
While npm is the most common package manager for Node.js, alternatives like Yarn and pnpm can also be used for installing Webpack. For example, to install Webpack using Yarn, run:
yarn global add webpack webpack-cli
For a local installation with Yarn, use:
yarn add --dev webpack webpack-cli
Similarly, pnpm can be used in the following way for global installation:
pnpm add -g webpack webpack-cli
Local installation with pnpm would look like this:
pnpm add --save-dev webpack webpack-cli
Updating dependencies for optimal functionality
Keeping Webpack and its dependencies up to date is vital for ensuring optimal performance and security. To update Webpack to its latest version, run:
npm update webpack webpack-cli
For Yarn, use:
yarn upgrade webpack webpack-cli
For pnpm, the command is similar:
pnpm update webpack webpack-cli
Regularly updating helps prevent issues related to outdated packages and ensures access to the latest features and bug fixes.
Related Article: How to Choose Between Gulp and Webpack
Additional Resources
- How to Install Webpack Globally