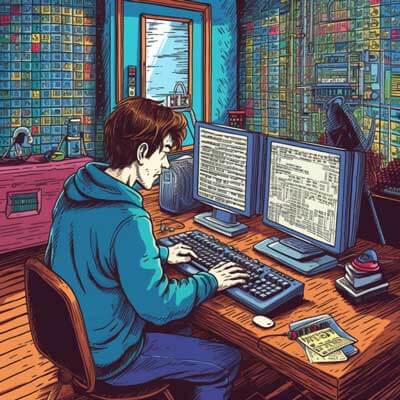
Table of Contents
To generate a GUID (Globally Unique Identifier) or UUID (Universally Unique Identifier) in Python, you can use the built-in uuid
module. This module provides functions for generating, representing, and manipulating UUIDs.
Using the uuid4() function
The simplest way to generate a UUID in Python is by using the uuid4()
function from the uuid
module. This function generates a random UUID using the version 4 UUID algorithm.
Here's an example of how to use the uuid4()
function to generate a UUID:
import uuid guid = uuid.uuid4() print(guid)
Output:
d53eabf6-9a0c-4e2f-87e1-5f2b52c42e6e
In the above example, we import the uuid
module and call the uuid4()
function, which returns a new UUID object. We then print the UUID object, which is automatically converted to its string representation.
Related Article: How to Use Python's isnumeric() Method
Using the uuid1() function
Another option for generating a UUID in Python is by using the uuid1()
function from the uuid
module. This function generates a UUID based on the current time and the MAC address of the computer.
Here's an example of how to use the uuid1()
function to generate a UUID:
import uuid guid = uuid.uuid1() print(guid)
Output:
5e5f9f9e-9a0c-11ec-9f50-5f2b52c42e6e
In the above example, we import the uuid
module and call the uuid1()
function, which returns a new UUID object. We then print the UUID object, which is automatically converted to its string representation.
Best practices for working with UUIDs
When working with UUIDs in Python, it's important to keep the following best practices in mind:
1. Use the uuid
module: Python provides the uuid
module as a standard library, which makes it easy to work with UUIDs. Avoid using external libraries unless you have specific requirements that are not met by the uuid
module.
2. Store UUIDs as strings: UUIDs are typically represented as strings, so it's recommended to store them as strings in your database or other data storage systems.
3. Avoid using UUIDs as primary keys: While it's technically possible to use UUIDs as primary keys in databases, it can have performance implications, especially for large datasets. Consider using sequential integer primary keys instead, and use UUIDs as additional identifiers if needed.
4. Generate UUIDs on the server side: If you need to generate UUIDs for your application, it's generally recommended to generate them on the server side rather than on the client side. This helps ensure uniqueness and prevents potential collisions.
5. Use UUID version 4 for random UUIDs: If you need to generate random UUIDs, use UUID version 4, which is designed for this purpose. UUID version 4 uses random data from the operating system's random number generator to ensure uniqueness.
6. Use UUID version 1 for time-based UUIDs: If you need to generate time-based UUIDs, use UUID version 1. UUID version 1 includes a timestamp component that can be used to determine the time when the UUID was generated.
Alternative ideas
While the uuid
module is the standard way to generate UUIDs in Python, there are also other libraries available that provide additional features or alternative implementations. Some popular alternatives include:
- shortuuid
: This library provides a shorter representation of UUIDs by using a combination of base57 encoding and a timestamp component. It can be useful in situations where you need shorter identifiers or want to obfuscate the underlying UUIDs.
- ulid
: The ULID (Universally Unique Lexicographically Sortable Identifier) specification is a combination of a timestamp and a random component, similar to UUID version 1. The ulid-py
library provides an implementation of ULIDs in Python.
- cuid
: CUID (Collision-resistant Unique Identifier) is a compact alternative to UUIDs that aims to be collision-resistant and human-friendly. The python-cuid
library provides an implementation of CUIDs in Python.
These libraries can be useful if you have specific requirements that are not met by the uuid
module or if you prefer alternative representations or algorithms for generating unique identifiers.