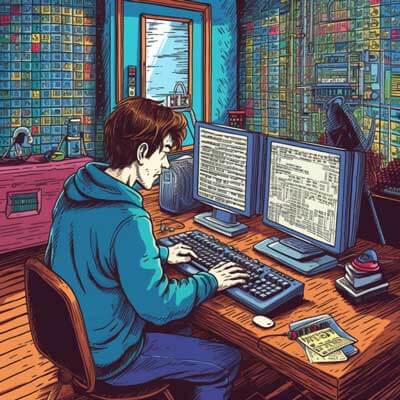
Table of Contents
Overview of Generating Source Maps for ESM
Source maps are essential for debugging in modern web applications. They link the minified or compiled code back to the original source code, making it easier to trace errors. When working with ECMAScript Modules (ESM) in Webpack, generating source maps allows developers to see their original JavaScript code during debugging, even after it has been bundled. This is particularly useful as ESM is becoming the standard for modular JavaScript, offering benefits like improved scoping and better dependency management.
Related Article: How To Fix Unhandled Exception Cannot Find Module Webpack
ESM and CommonJS Differences
ESM and CommonJS are two different module systems in JavaScript. CommonJS is primarily used in Node.js and employs a synchronous loading mechanism, using require
to import modules. ESM, on the other hand, is asynchronous and uses the import
statement, allowing for better optimization in modern browsers.
A key difference lies in how they handle exports. CommonJS uses module.exports
to export values, while ESM uses the export
statement. This distinction affects how source maps are generated and interpreted, as ESM's static structure allows better tree-shaking and optimizations in bundlers like Webpack.
// CommonJS example const myModule = require('./myModule'); module.exports = myModule; // ESM example import myModule from './myModule.js'; export default myModule;
Setting Up Source Maps in Development Mode
To enable source maps in development mode, you need to adjust your Webpack configuration. The devtool
property in the Webpack configuration file determines how source maps are generated. For development, a popular option is eval-source-map
, which provides high-quality source maps.
Example configuration:
// webpack.config.js module.exports = { mode: 'development', devtool: 'eval-source-map', entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist', }, // Additional configurations... };
This setting allows for fast rebuilds and easy debugging, as changes in source files will be reflected in the source maps without needing a full reload.
Configuring for Production Builds
For production builds, you want to balance between the quality of source maps and file size. A common choice is source-map
, which generates complete source maps but keeps them separate from the bundle. This is useful for detailed debugging without exposing the source code in the production environment.
Example configuration:
// webpack.config.js module.exports = { mode: 'production', devtool: 'source-map', entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist', }, // Additional configurations... };
This setup allows you to retain the benefits of source maps while keeping your production builds optimized.
Related Article: How to Use Django Webpack Loader with Webpack
Customizing Settings
Webpack allows customization of source map settings based on specific needs. The devtool
option can take several values, each providing different levels of source map detail and performance. Options include:
- cheap-module-source-map
: Generates source maps without column mappings, providing faster builds and smaller files.
- inline-source-map
: Embeds the source map directly in the bundle, which can be useful for debugging during development.
Example of using cheap-module-source-map
:
// webpack.config.js module.exports = { mode: 'development', devtool: 'cheap-module-source-map', entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist', }, // Additional configurations... };
Experimenting with these options helps find the right balance for your project.
How Source Maps Enhance Debugging
Source maps greatly enhance debugging by providing a clear link between the minified code and the original source code. This means when an error occurs, the developer can see the exact line in their original files rather than in the generated bundle. For example, if an error occurs in the bundled file at a particular line, the source map will point back to the exact line in the original file.
In Chrome Developer Tools, for instance, you can see your original source files, set breakpoints, and inspect variables directly in the context of your original code. This significantly reduces the time spent figuring out where an issue lies.
Performance Impact
Using source maps can impact performance, particularly in production environments. While they provide valuable debugging information, they can also increase the size of the assets served by the web server. When source maps are included in production builds, they can expose the source code, which might not be desirable.
To mitigate performance impacts, consider the following:
- Use source-map
in production to keep source files separate from the main bundle.
- Avoid including source maps in production unless necessary for debugging purposes.
- Use tools like webpack-bundle-analyzer
to analyze and optimize your bundle sizes.
Generating Source Maps for Different File Types
Webpack supports various file types, including JavaScript, CSS, and images. Source maps can be generated for all these types, allowing for comprehensive debugging.
For CSS, for example, you can enable source maps in the css-loader
configuration:
// webpack.config.js module.exports = { module: { rules: [ { test: /\.css$/, use: [ 'style-loader', { loader: 'css-loader', options: { sourceMap: true, }, }, ], }, ], }, // Additional configurations... };
This configuration generates source maps for your CSS files, allowing you to debug styles accurately.
Related Article: How to Use the Compression Plugin in Webpack
Using External Source Maps
External source maps are beneficial for keeping the source maps separate from the bundled code. This can help reduce the size of your main files and keep your source code hidden from users. To enable external source maps, you can configure the devtool
option to source-map
and ensure that the source maps are outputted as separate files.
Example configuration:
// webpack.config.js module.exports = { mode: 'production', devtool: 'source-map', entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist', sourceMapFilename: '[file].map', // Generates a separate .map file }, // Additional configurations... };
This setup ensures that the source maps are available without cluttering your main JavaScript bundle.
Troubleshooting
Source map issues can arise due to various reasons, such as incorrect paths or misconfigurations. Common troubleshooting steps include:
1. Verify the devtool
setting: Ensure it is correctly set based on your environment (development or production).
2. Check file paths: Ensure the paths in your source maps point to the correct files.
3. Review console errors: Browsers often provide warnings or errors related to source maps in the console, which can guide troubleshooting.
Example of a console error related to source maps:
DevTools failed to load source map: Could not load content for http://localhost:3000/dist/bundle.js.map: 404 (Not Found)
This indicates the source map file is not found, which may require checking the output configuration in Webpack.
Code Splitting
Code splitting is a technique used to divide your code into smaller chunks, which can be loaded on demand. This improves performance, especially for large applications. When using code splitting with Webpack, source maps are generated for each chunk, allowing debugging across different parts of your application.
To enable code splitting, you can use dynamic imports in your code:
// src/index.js import('./moduleA').then(module => { module.doSomething(); });
Webpack will automatically generate separate bundles for each module, and source maps will be created for each chunk, helping maintain a clear debugging experience.
Additional Resources
- Enabling Source Maps in Webpack