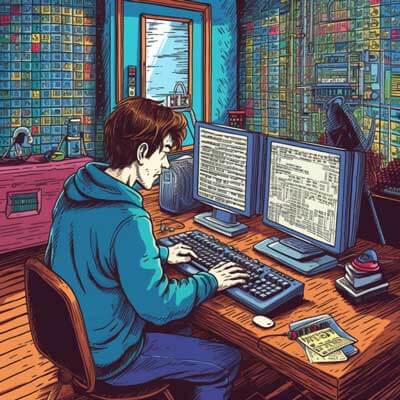
Table of Contents
To get the current date and time in Javascript, you can use the built-in Date
object. The Date
object provides various methods to retrieve different components of the current date and time. Here are two possible ways to get the current date and time in Javascript:
Method 1: Using the toLocaleString
Method
One simple way to get the current date and time in a human-readable format is by using the toLocaleString
method of the Date
object. This method returns a string that represents the current date and time in the user's local time zone.
Here's an example:
const currentDateAndTime = new Date().toLocaleString(); console.log(currentDateAndTime);
This will output the current date and time in a format like "7/1/2022, 10:30:45 AM" depending on the user's local settings.
Related Article: How To Fix the 'React-Scripts' Not Recognized Error
Method 2: Using the Individual Methods
If you need to access individual components of the current date and time separately, you can use the following methods provided by the Date
object:
- getFullYear()
: Returns the current year as a four-digit number.
- getMonth()
: Returns the current month as a zero-based index (0 for January, 1 for February, etc.).
- getDate()
: Returns the current day of the month as a number.
- getHours()
: Returns the current hour (0-23).
- getMinutes()
: Returns the current minute (0-59).
- getSeconds()
: Returns the current second (0-59).
- getMilliseconds()
: Returns the current millisecond (0-999).
Here's an example that demonstrates how to use these methods:
const currentDate = new Date(); const year = currentDate.getFullYear(); const month = currentDate.getMonth() + 1; // Adding 1 to adjust for zero-based index const day = currentDate.getDate(); const hours = currentDate.getHours(); const minutes = currentDate.getMinutes(); const seconds = currentDate.getSeconds(); console.log(`${year}-${month}-${day} ${hours}:${minutes}:${seconds}`);
This will output the current date and time in a format like "2022-07-01 10:30:45".
Alternative Ideas and Best Practices
Related Article: How to Use Javascript Substring, Splice, and Slice
- If you are working with date and time manipulation extensively, consider using a library like Moment.js or Luxon. These libraries provide more advanced functionality and make working with dates and times easier.
- When working with dates and times, be mindful of time zones. The Date
object in Javascript operates based on the user's local time zone. If you need to work with specific time zones, you may need to use third-party libraries or handle the time zone conversions manually.
Overall, getting the current date and time in Javascript is straightforward using the Date
object's methods. Choose the method that best suits your needs based on whether you need a human-readable format or individual components of the date and time.