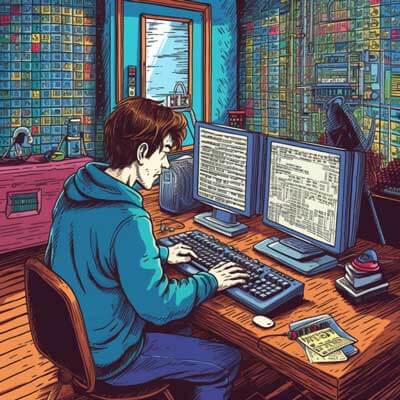
Table of Contents
To get query string values in JavaScript, you can use the URLSearchParams
API or manually parse the URL string. Here are two possible approaches:
Using the URLSearchParams API
The URLSearchParams
API provides a convenient way to work with query strings. It allows you to easily access and manipulate the parameters in a URL's query string.
Here's an example of how you can use the URLSearchParams
API to get query string values:
// Assuming the URL is https://example.com/?name=John&age=30 const urlParams = new URLSearchParams(window.location.search); const name = urlParams.get('name'); const age = urlParams.get('age'); console.log(name); // Output: John console.log(age); // Output: 30
In the example above, we first create a new URLSearchParams
object by passing window.location.search
as the parameter. This retrieves the query string from the current URL.
We then use the get()
method of the URLSearchParams
object to retrieve the value of a specific parameter. In this case, we retrieve the values of the name
and age
parameters.
Related Article: How to Use a Regular Expression to Match a URL in JavaScript
Manually Parsing the URL
If you prefer not to use the URLSearchParams
API, you can manually parse the URL string to extract the query string values. This approach gives you more control over the parsing process.
Here's an example of how you can manually parse the URL to get query string values:
// Assuming the URL is https://example.com/?name=John&age=30 function getQueryParams(url) { const queryString = url.split('?')[1]; const params = {}; if (queryString) { queryString.split('&').forEach((param) => { const [key, value] = param.split('='); params[key] = decodeURIComponent(value); }); } return params; } const queryParams = getQueryParams(window.location.href); console.log(queryParams.name); // Output: John console.log(queryParams.age); // Output: 30
In the example above, we define a function called getQueryParams()
that takes a URL as input.
Inside the function, we first split the URL string at the ?
character to separate the base URL from the query string.
We then split the query string at the &
character to separate individual parameters. For each parameter, we further split it at the =
character to separate the key and value.
Finally, we decode the URL-encoded value using decodeURIComponent()
and store the key-value pairs in an object.
The getQueryParams()
function returns the object containing the query string parameters.
Suggestions and Best Practices
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
- When using the URLSearchParams
API, make sure to check browser compatibility. It is supported in most modern browsers but may not work in older versions.
- If you need to support older browsers or have more complex query string parsing requirements, consider using a third-party library like query-string
or qs
. These libraries provide additional functionality and support for different parsing options.
- To handle query string values with repeated keys (e.g., ?color=red&color=blue
), the URLSearchParams
API provides the getAll()
method to retrieve all values associated with a key.
- When manually parsing the URL, it's important to handle URL encoding properly. Use decodeURIComponent()
to decode URL-encoded values.
- To get query string values from a URL other than the current one (e.g., a stored URL string), pass the URL as a parameter to the respective approach.
- Remember that query string values are always treated as strings. If you need to convert them to other data types (e.g., numbers, booleans), you can use JavaScript's built-in conversion functions like parseInt()
or parseFloat()
.
- Be mindful of security considerations when working with query string values. Validate and sanitize user input to prevent against potential vulnerabilities like SQL injection or cross-site scripting (XSS) attacks.