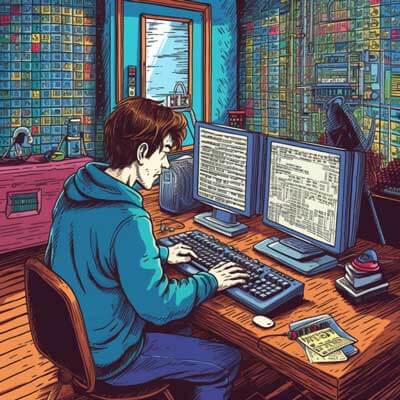
Table of Contents
To get the selected option from a dropdown using jQuery, you can use the val()
function along with the :selected
selector. Here are two possible approaches:
Approach 1: Using the val() function
You can use the val()
function to get the value of the selected option from a dropdown. Here's an example:
// HTML Option 1 Option 2 Option 3 // JavaScript var selectedValue = $('#myDropdown').val(); console.log(selectedValue); // Output: the value of the selected option
In this example, we use the val()
function on the dropdown element with the ID myDropdown
. This will return the value of the selected option.
Related Article: How to Integrate Redux with Next.js
Approach 2: Using the :selected selector
Another approach is to use the :selected
selector along with the val()
function. Here's an example:
// HTML Option 1 Option 2 Option 3 // JavaScript var selectedValue = $('#myDropdown option:selected').val(); console.log(selectedValue); // Output: the value of the selected option
In this example, we use the option:selected
selector to target the selected option within the dropdown. Then, we use the val()
function to get its value.
Additional Suggestions
- If you want to get the text of the selected option instead of the value, you can use the text()
function instead of val()
. For example: var selectedText = $('#myDropdown option:selected').text();
- If you have multiple dropdowns on the same page and want to get the selected option from a specific dropdown, make sure to use a unique ID for each dropdown and target the correct ID in your jQuery selector.
- If you want to perform some action when the selected option changes, you can use the change()
event. Here's an example:
// HTML Option 1 Option 2 Option 3 // JavaScript $('#myDropdown').change(function() { var selectedValue = $(this).val(); console.log(selectedValue); // Output: the value of the newly selected option });
In this example, we attach a change()
event handler to the dropdown element. Whenever the selected option changes, the code inside the event handler will be executed.
Best Practices
- It is recommended to use unique IDs for your dropdowns to avoid any conflicts or unexpected behavior.
- Make sure to include the jQuery library before your JavaScript code to ensure that the jQuery functions are available.
- Use descriptive variable names to improve code readability and maintainability.
- If you're working with a large number of dropdowns or need to perform more complex operations, consider using a JavaScript framework like React or Vue.js to manage state and simplify your code.