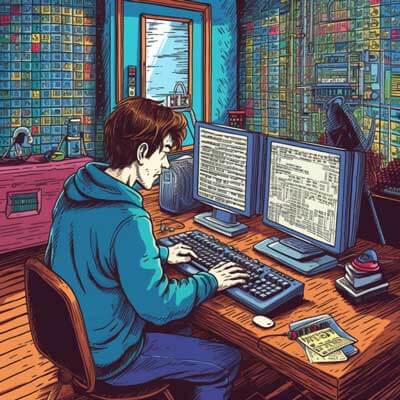
Table of Contents
To get the selected value in a dropdown list using JavaScript, you can use the following approaches:
1. Using the value property of the selected option
You can access the selected value of a dropdown list by using the value property of the selected option. Here's an example:
// HTML Option 1 Option 2 Option 3 // JavaScript const dropdown = document.getElementById("myDropdown"); const selectedValue = dropdown.value; console.log(selectedValue); // Output: "option2"
In the above example, we first obtain a reference to the dropdown element using its ID. Then, we access the value property of the dropdown element to retrieve the selected option's value. Finally, we log the selected value to the console.
Related Article: How to Use Angular Reactive Forms
2. Using the selectedIndex property and options collection
Another way to get the selected value in a dropdown list is by using the selectedIndex property and the options collection. Here's an example:
// HTML Option 1 Option 2 Option 3 // JavaScript const dropdown = document.getElementById("myDropdown"); const selectedOption = dropdown.options[dropdown.selectedIndex]; const selectedValue = selectedOption.value; console.log(selectedValue); // Output: "option2"
In this example, we first obtain a reference to the dropdown element using its ID. Then, we use the selectedIndex property to get the index of the selected option. We can then access the selected option using the options collection and the obtained index. Finally, we retrieve the value property of the selected option and log it to the console.
Best Practices
Related Article: How To Use the Javascript Void(0) Operator
- It's important to ensure that the JavaScript code is executed after the DOM has finished loading. You can achieve this by placing your code inside a DOMContentLoaded event listener or by placing the script tag at the end of the HTML body.
- When selecting elements by ID, make sure the ID is unique within the document. Otherwise, the code may not select the intended element.
- To improve code readability and maintainability, consider assigning the dropdown element to a variable with a meaningful name.
- When accessing the selected value multiple times, it's more efficient to store it in a variable rather than repeatedly accessing the DOM.
These are the main ways to get the selected value in a dropdown list using JavaScript. Choose the approach that best suits your needs and coding style.