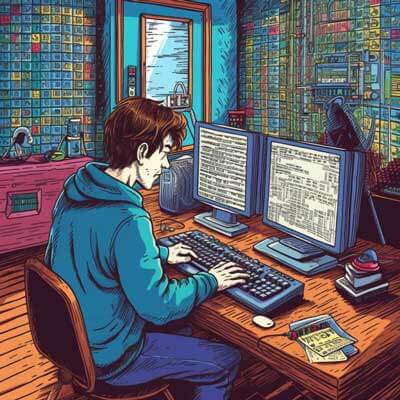
- Overview of GraphQL
- How GraphQL Works
- Main Components of GraphQL
- Defining a GraphQL Schema
- GraphQL Queries
- Queries and Mutations
- Setting Up a GraphQL Server
- Resolvers in GraphQL
- Using Variables in Queries
- Using Fragments in Queries
- Introspection in GraphQL
- Working with Subscriptions
- Creating GraphQL Endpoints
- Types and Fields in GraphQL
Overview of GraphQL
GraphQL is a query language for APIs that provides a more efficient and flexible alternative to the traditional REST API. It allows clients to request only the data they need, which can significantly reduce the amount of data transferred over the network. The core idea is that instead of having multiple endpoints for different resources, GraphQL exposes a single endpoint that can handle all types of requests. This results in a more streamlined approach to data fetching and manipulation.
When using GraphQL, clients specify their data requirements in a structured way, which leads to a clear and predictable API. This simplicity aids in the development of client applications as they can query for exactly what they need without over-fetching or under-fetching data.
Related Article: How to Ignore But Handle GraphQL Errors
How GraphQL Works
At its core, GraphQL organizes data into a graph structure. This means that every item of data can be thought of as a node in a graph, and the relationships between data items are represented as edges. The client sends a query to the server, specifying the structure of the data it requires. The server then responds with a JSON object that matches the structure defined in the query.
Clients can interact with the server using three types of operations: queries, mutations, and subscriptions. Queries are used to fetch data, mutations are for modifying data, and subscriptions allow clients to listen for real-time updates to data. This framework allows for dynamic interactions between the client and server, fostering a more interactive user experience.
Main Components of GraphQL
The main components of GraphQL include the schema, queries, mutations, and resolvers. The schema defines the types of data that can be queried or mutated, including objects, scalar types, and enums. Queries allow the client to request data, while mutations enable data modifications. Resolvers are functions that handle the logic for fetching or modifying data as specified by the queries or mutations.
Understanding these components is critical for designing a well-structured GraphQL API. The schema serves as the contract between the client and server, ensuring that both sides understand the types of data being exchanged.
Defining a GraphQL Schema
Creating a GraphQL schema involves defining types and their relationships. Types can be scalars, like strings and integers, or complex objects that can have fields of their own. A typical schema is defined using the Schema Definition Language (SDL).
For example, a simple schema for a blog might look like this:
type Post { id: ID! title: String! content: String! author: User! } type User { id: ID! name: String! posts: [Post] } type Query { posts: [Post] users: [User] }
In this schema, the Post
type has fields for id
, title
, content
, and an author
, which links to the User
type. The Query
type defines the entry points for fetching data.
Related Article: How to Query Data in GraphQL
GraphQL Queries
Queries are the heart of GraphQL. They allow clients to request specific data from the server. A query is written in a syntax that closely resembles JSON.
Here is an example query to fetch posts:
query { posts { id title content } }
This query asks for the id
, title
, and content
of all posts. The response will return a JSON object that mirrors the structure of the query, ensuring clarity and predictability in the data returned.
Queries and Mutations
While queries retrieve data, mutations are used to change it. Mutations follow a similar syntax to queries but often include input parameters to specify the data being modified.
For example, to create a new post, a mutation might look like this:
mutation { createPost(title: "New Post", content: "This is the content of the new post") { id title content } }
This mutation calls the createPost
function, passing in the title and content for the new post. The server will then return the new post’s id
, title
, and content
as part of the response.
Setting Up a GraphQL Server
Setting up a GraphQL server typically involves choosing a server framework and defining the schema and resolvers. Popular choices include Apollo Server and Express with Apollo Server middleware.
For example, using Apollo Server with Node.js, the setup looks like this:
const { ApolloServer, gql } = require('apollo-server'); const typeDefs = gql` type Post { id: ID! title: String! content: String! } type Query { posts: [Post] } `; const resolvers = { Query: { posts: () => [ { id: "1", title: "Post One", content: "Content of Post One" }, { id: "2", title: "Post Two", content: "Content of Post Two" }, ], }, }; const server = new ApolloServer({ typeDefs, resolvers }); server.listen().then(({ url }) => { console.log(`Server ready at ${url}`); });
This code defines a simple server that exposes a posts
query. When the server is run, it listens for incoming requests and responds with the hardcoded posts.
Related Article: Exploring Directus GraphQL
Resolvers in GraphQL
Resolvers are functions that provide the instructions for turning a GraphQL operation into data. Each field in a schema can have a corresponding resolver function that defines how to fetch or compute the value for that field.
For example, if a Post
has an author
field, the resolver for that field might look like this:
const resolvers = { Post: { author: (post) => { // Logic to retrieve author data based on post.id return getUserByPostId(post.id); }, }, };
In this example, the resolver accesses the post
object and retrieves the author information based on the post’s ID. This approach decouples the data fetching logic from the schema definition, promoting better organization and maintainability.
Using Variables in Queries
GraphQL supports the use of variables in queries, which allows for more dynamic and reusable queries. Variables can be defined in the query and passed at runtime, making it easier to work with user input or other changing data.
An example query with variables could look like this:
query GetPost($postId: ID!) { post(id: $postId) { title content } }
In this case, $postId
is a variable that will be provided when the query is executed. This method minimizes hardcoded values in queries, allowing for more adaptable code.
Using Fragments in Queries
Fragments allow for code reuse in queries by enabling the definition of reusable units of query logic. This is particularly useful when multiple queries or mutations require the same fields.
Here is an example of a fragment:
fragment PostFields on Post { id title content } query { posts { ...PostFields } }
Using the fragment PostFields
, the query retrieves the same set of fields for each post without repeating the field definitions. This makes queries cleaner and easier to maintain.
Related Article: Step by Step Process: Passing Enum in GraphQL Query
Introspection in GraphQL
Introspection is a feature that allows clients to query the schema itself. This means clients can find out the types, fields, and operations available in the GraphQL API, which aids in development and debugging.
A simple introspection query might look like this:
{ __schema { types { name } } }
This query returns a list of all types defined in the schema. Most GraphQL tools and libraries leverage introspection to provide features like auto-completion and documentation generation.
Working with Subscriptions
Subscriptions provide a way to maintain a real-time connection to the server. They allow clients to listen for events and receive updates when data changes. This is particularly useful for applications like chat apps or live dashboards.
A typical subscription might look like this:
subscription { postCreated { id title content } }
When a new post is created, clients subscribed to this event will receive the new post’s details automatically without having to refresh or re-query the data.
Creating GraphQL Endpoints
Creating GraphQL endpoints involves setting up a server that can handle GraphQL requests. Generally, a single endpoint is created to handle all queries, mutations, and subscriptions.
For instance, in an Express application, you might use the following code:
const express = require('express'); const { ApolloServer } = require('apollo-server-express'); const app = express(); const server = new ApolloServer({ typeDefs, resolvers }); server.applyMiddleware({ app }); app.listen({ port: 4000 }, () => console.log(`Server ready at http://localhost:4000${server.graphqlPath}`) );
This code integrates Apollo Server with an Express application, allowing for a GraphQL API to be served at a specific path.
Related Article: Achieving Production-Ready GraphQL
Types and Fields in GraphQL
Types and fields form the backbone of a GraphQL schema. Every type can have fields, which can themselves be other types or scalars. Scalars are the basic data types provided by GraphQL, including String
, Int
, Float
, Boolean
, and ID
.
For example, a User
type might have fields like this:
type User { id: ID! name: String! email: String! posts: [Post] }
In this example, the User
type has three scalar fields and a field posts
, which is an array of Post
objects. This structure allows for rich, nested data relationships, making it easier to model complex data.