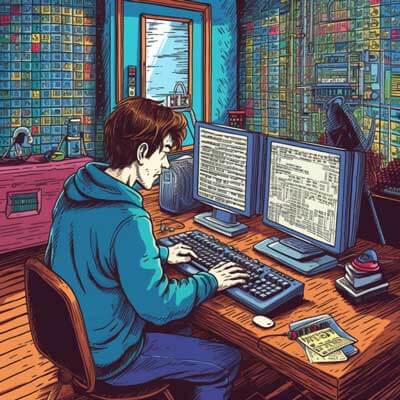
Table of Contents
To get the current URL with JavaScript, you can use the window.location
object. This object provides information about the current URL of the page and allows you to access different parts of the URL, such as the protocol, host, path, and query parameters.
Here are two possible ways to get the current URL using JavaScript:
Method 1: Using window.location.href
One simple way to get the current URL is by using the window.location.href
property. This property returns the complete URL of the current page as a string.
const currentUrl = window.location.href; console.log(currentUrl);
This will log the current URL to the console. You can also use the currentUrl
variable to perform further operations, such as extracting specific parts of the URL.
Related Article: How to Use Ngif Else in AngularJS
Method 2: Using window.location
object properties
Alternatively, you can use the properties of the window.location
object to access specific parts of the URL individually. Here are some of the commonly used properties:
- window.location.protocol
: Returns the protocol (e.g., "http:" or "https:").
- window.location.host
: Returns the hostname and port number.
- window.location.hostname
: Returns the hostname without the port number.
- window.location.port
: Returns the port number.
- window.location.pathname
: Returns the path of the URL.
- window.location.search
: Returns the query parameters as a string.
- window.location.hash
: Returns the URL fragment identifier (e.g., "#section1").
const protocol = window.location.protocol; const host = window.location.host; const pathname = window.location.pathname; const search = window.location.search; const hash = window.location.hash; console.log("Protocol:", protocol); console.log("Host:", host); console.log("Pathname:", pathname); console.log("Query parameters:", search); console.log("Fragment identifier:", hash);
This will log each part of the URL individually. You can customize the code snippet based on your specific requirements.
Best Practices and Suggestions
Related Article: How to Build Interactive Elements with JavaScript
- When working with the current URL, it is important to consider URL encoding and decoding. JavaScript provides functions like encodeURIComponent()
and decodeURIComponent()
to handle URL encoding and decoding, respectively. These functions are useful when dealing with query parameters or dynamically constructing URLs.
- If you are using JavaScript in a browser environment, make sure to test your code across different browsers to ensure compatibility. Browser inconsistencies might exist in certain scenarios, especially when dealing with special characters or non-standard URL formats.
- When manipulating the URL or extracting specific parts of it, always validate and sanitize user input to prevent security vulnerabilities, such as cross-site scripting (XSS) attacks or path traversal attacks.
- If you are working with URLs in a Node.js environment, consider using the url
module that comes with Node.js. This module provides a more structured API for parsing and manipulating URLs.
- If you need to modify the URL and navigate to a different page, you can use the window.location.href
property to set the new URL. For example, window.location.href = "https://example.com/new-page"
will redirect the browser to the specified URL.
These are some best practices and suggestions to consider when working with the current URL in JavaScript.