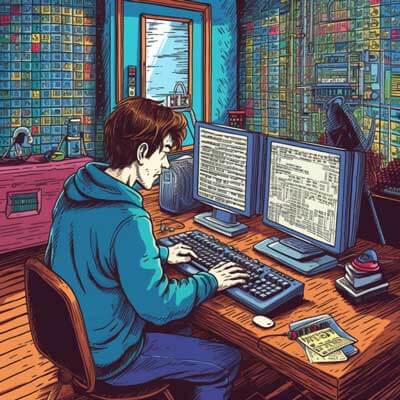
Table of Contents
To get the ID of an element using jQuery, you can use the .attr()
method or the .prop()
method. Both methods allow you to access and modify attributes of HTML elements, including the ID attribute.
Using the .attr() Method:
The .attr()
method in jQuery is used to get or set the value of an attribute for the selected elements. To get the ID of an element, you can use the following syntax:
var elementID = $("selector").attr("id");
Replace "selector" with the appropriate selector for the element you want to target. This could be a class, an ID, or any other valid CSS selector. The elementID
variable will then contain the ID of the selected element.
Here's an example:
<div id="myElement">Hello, World!</div>
var elementID = $("#myElement").attr("id"); console.log(elementID); // Output: myElement
In this example, the .attr()
method is used to get the ID of the <div>
element with the ID "myElement". The ID is then logged to the console.
Related Article: How to Fetch Post JSON Data in Javascript
Using the .prop() Method:
The .prop()
method in jQuery is similar to the .attr()
method but is primarily used to get or set properties of elements, such as the checked state of a checkbox. However, it can also be used to get the ID of an element.
To get the ID of an element using the .prop()
method, you can use the following syntax:
var elementID = $("selector").prop("id");
Replace "selector" with the appropriate selector for the element you want to target. The elementID
variable will then contain the ID of the selected element.
Here's an example:
<div id="myElement">Hello, World!</div>
var elementID = $("#myElement").prop("id"); console.log(elementID); // Output: myElement
In this example, the .prop()
method is used to get the ID of the <div>
element with the ID "myElement". The ID is then logged to the console.
Best Practices:
Related Article: How to Retrieve Values From Get Parameters in Javascript
When using jQuery to get the ID of an element, it's important to keep in mind some best practices:
1. Use specific selectors: To ensure you're targeting the correct element, use specific selectors like class names or IDs. This helps avoid conflicts and improves performance.
2. Avoid using reserved keywords: Be cautious when using reserved keywords as IDs, as they might cause issues or conflicts with JavaScript code.
3. Cache your selectors: If you need to access the same element multiple times, consider storing it in a variable for better performance. This avoids re-querying the DOM every time you need to access the element.
4. Use the .prop()
method for getting properties: While the .attr()
method can be used to get the ID, it is generally recommended to use the .prop()
method for accessing properties like the ID. The .prop()
method is more efficient and performs better in most cases.
Overall, getting the ID of an element using jQuery is straightforward and can be done easily using either the .attr()
or .prop()
method. By following best practices, you can ensure efficient and reliable code when working with element IDs in jQuery.