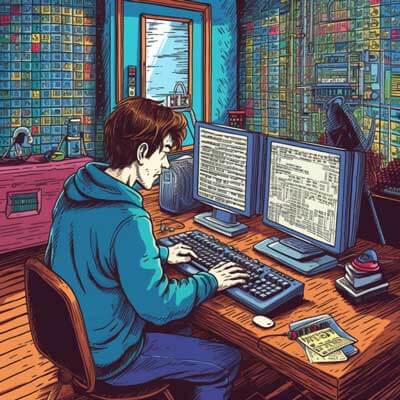
Table of Contents
Overview of npm Warn Messages
npm (Node Package Manager) is a critical tool for managing packages in JavaScript applications. It provides warnings to help developers address potential issues in their projects. npm warn messages act as alerts signaling that something may not be quite right. These warnings can arise from various sources, such as outdated packages, potential vulnerabilities, or deprecated features. The intent behind these warnings is to guide developers toward best practices and ensure the software remains stable and secure.
For instance, you might encounter a warning when trying to install a package that has dependencies requiring different versions. This can lead to conflicts that might break your application. Ignoring these warnings can lead to bigger issues down the line, making it crucial to pay attention to them.
Related Article: How to Use npm Tiny Invariant in Your Project
What Does 'npm Warn Using --Force Recommended Protections Disabled' Mean
This specific warning indicates that you are executing an npm command with the --force
flag, which disables some of npm's built-in protections. By default, npm includes safeguards to prevent certain actions that could lead to instability or security vulnerabilities. When you use the --force
option, you override these protections, allowing npm to proceed with actions that it would typically block.
For example, if you attempt to install a package that has known vulnerabilities or conflicts, npm will usually prevent this action. However, adding the --force
option tells npm to ignore these warnings and proceed anyway. This can be useful in certain situations but should be approached with caution.
When to Use --Force with npm
Using the --force
flag can be appropriate in specific scenarios. For instance, when you are confident that the warnings generated by npm do not apply to your use case, or when you are trying to install a package that fails due to dependency issues but you understand the implications. Here's an example command:
npm install <package-name> --force
In this example, replace <package-name>
with the name of the package you wish to install. This command forces the installation, bypassing any warnings that npm might generate regarding dependencies or vulnerabilities. However, this should generally be reserved for development or testing environments rather than production.
Risks of Using --Force in npm
Using --force
carries inherent risks. By bypassing npm's safeguards, you might inadvertently introduce bugs, security vulnerabilities, or other issues into your application. Some common risks include:
1. Dependency Conflicts: Ignoring version mismatches can lead to runtime errors.
2. Security Vulnerabilities: Installing packages with known vulnerabilities may expose your application to attacks.
3. Instability: Unstable versions of packages may break functionality or lead to crashes.
It is essential to evaluate these risks carefully before deciding to use the --force
flag. The consequences can be significant, especially in a production environment.
Related Article: How to Use npm with Next.js
Avoiding the --Force Warning
To avoid the warning about using --force
, consider resolving the underlying issues that cause npm to flag the command. Some methods to achieve this include:
1. Updating Packages: Ensure all your dependencies are up to date. Use the following command to check for outdated packages:
npm outdated
2. Addressing Vulnerabilities: You can use npm audit to identify vulnerabilities and npm audit fix to attempt automatic fixes.
3. Reviewing Peer Dependencies: If you encounter peer dependency warnings, review the versions specified in your package.json
and adjust accordingly.
Protections Disabled by --Force
When using the --force
flag, npm disables several safeguards designed to protect the integrity of your project. These protections include:
1. Dependency Resolution: npm will not enforce strict dependency resolutions, allowing potentially conflicting versions to be installed.
2. Vulnerability Warnings: Known vulnerabilities will not prevent the installation of packages.
3. Peer Dependency Conflicts: npm ignores peer dependency issues, which could lead to unexpected behavior in your application.
These disabled protections can leave your application in a fragile state, making it crucial to weigh the decision to use --force
carefully.
Ignoring the npm Warn Using --Force Message
Ignoring the warning about using --force
can be tempting, especially when you need to get a package installed quickly. However, doing so can lead to significant long-term issues. If you choose to ignore this warning, ensure you have a plan in place to monitor for any problems that might arise from this action.
In many cases, it may be preferable to take the extra time to resolve the underlying issues rather than risk the stability of your project. If you find yourself repeatedly using --force
, consider revisiting your dependency management practices.
Consequences of Using --Force
The consequences of using --force
can manifest in various ways. You might encounter:
1. Unstable Application Behavior: Conflicting dependencies can lead to unexpected crashes or bugs.
2. Difficult Debugging: When issues arise, it may be challenging to pinpoint the cause if multiple packages are conflicting.
3. Increased Technical Debt: Relying on --force
can lead to a build-up of unresolved issues, making it harder to maintain the codebase over time.
Considering these potential consequences highlights the importance of careful package management and the need to understand the implications of using --force
.
Related Article: How to Track the History of npm Packages
Reverting Changes After --Force Usage
If you decide to use --force
and later encounter issues, reverting changes can be a complex process. Here are some steps to help manage this:
1. Version Control: Always use version control systems like Git. Commit your changes before running commands with --force
, so you can easily revert to a stable state.
git commit -m "Backup before using --force"
2. Reinstall Packages: If you identify a problem, you may need to remove the problematic package and reinstall it without --force
. Use the following commands:
npm uninstall <package-name>npm install <package-name>
Replacing <package-name>
with the name of the package you wish to uninstall.
3. Audit Your Dependencies: After reverting, run npm audit to check for vulnerabilities and ensure that your dependencies are in a healthy state.
Impact of --Force on Package Installations
The impact of using --force
on package installations can be significant. It can lead to:
1. Broken Builds: If incompatible versions are installed, your builds might fail, causing delays in development.
2. Runtime Errors: You might encounter runtime errors that stem from conflicts or unstable versions, which can disrupt user experience.
3. Increased Maintenance Efforts: More time may be required to track down bugs and resolve issues caused by the forced installations.
Being aware of these impacts can help you make more informed decisions when considering the use of --force
.
Recommended Ways to Handle npm Warnings
Handling npm warnings effectively is crucial for maintaining a healthy codebase. Some strategies include:
1. Regularly Update Dependencies: Keeping your packages updated minimizes the chances of conflicts or vulnerabilities.
npm update
2. Conduct Security Audits: Regularly running npm audit helps identify and address vulnerabilities before they become a problem.
3. Carefully Evaluate Warnings: Take the time to understand each warning and what it means for your project. This can save time and effort in the long run.
4. Consult Documentation: Sometimes, the issue may stem from package-specific requirements or changes. Always consult the documentation for the packages you are using.