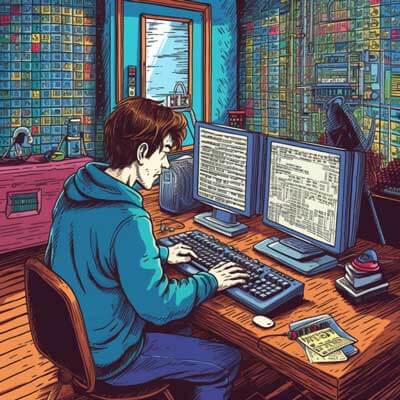
Table of Contents
To implement the HTML select multiple as a dropdown, you can follow these steps:
Step 1: Create a basic HTML structure
Create a basic HTML structure for your dropdown. You can use the element to create a dropdown and add the
multiple
attribute to allow multiple selections.
<!-- options go here -->
Related Article: Comparing Sorting Algorithms (with Java Code Snippets)
Step 2: Add options to the dropdown
Inside the element, add
elements to define the available options in the dropdown. Each
element should have a value attribute to specify its value.
Option 1 Option 2 Option 3
Step 3: Style the dropdown
select[multiple] { height: auto; width: 200px; padding: 5px; }
Step 4: Handle the selected options
To handle the selected options in the dropdown, you can use JavaScript. You can listen for the change
event on the element and retrieve the selected options using the
selectedOptions
property.
const selectElement = document.querySelector('select[multiple]'); selectElement.addEventListener('change', () => { const selectedOptions = Array.from(selectElement.selectedOptions).map(option => option.value); console.log(selectedOptions); });
In the above example, the selected options are logged to the console whenever the selection changes.
Related Article: Tutorial: Supported Query Types in Elasticsearch
Alternative approach using a custom dropdown
If you need more control over the appearance and behavior of the multiple select dropdown, you can also use a custom dropdown library or framework. There are several libraries available that provide more advanced features and customization options. Some popular ones include Select2, Chosen, and Bootstrap Select. These libraries allow you to enhance the default multiple select dropdown with features like search functionality, tagging, and custom styling.
Best Practices
Here are some best practices to consider when implementing an HTML select multiple as a dropdown:
- Provide clear labels for the options in the dropdown to make it easier for users to understand their choices.
- Use the size
attribute to specify the number of visible options in the dropdown. This can help prevent the dropdown from taking up too much space on the page.
- Consider adding a "Select All" option if it makes sense for your use case. This can be useful when users need to select multiple options at once.
- Ensure that the selected options are visually distinguishable from the unselected options to provide clear feedback to the user.
- Test your implementation across different browsers and devices to ensure consistent behavior and appearance.