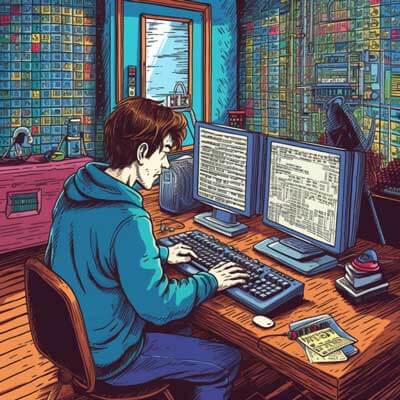
Table of Contents
Inline styles in React JS allow you to apply styles directly to individual components. This can be useful for adding dynamic styles or overriding default styles. However, it's important to follow best practices when using inline styles to ensure maintainability and readability of your code. Here are some steps to implement inline style best practices in React JS:
1. Use JavaScript objects for inline styles
In React JS, inline styles are defined using JavaScript objects. This allows you to dynamically generate styles based on component props or state. To define inline styles, create a JavaScript object with key-value pairs where the keys are CSS property names in camel case and the values are the corresponding property values. For example:
const styles = { container: { backgroundColor: 'red', padding: '10px', }, text: { color: 'white', fontSize: '16px', }, }; function MyComponent() { return ( <div> <p>Hello, world!</p> </div> ); }
Related Article: How To Replace All Occurrences Of A String In Javascript
2. Separate styles from component logic
To improve code maintainability and readability, it's best to separate the styles from the component logic. You can define the styles in a separate file or at the top of your component file. By doing this, you can easily locate and modify the styles without cluttering the component logic. For example:
// styles.js export const container = { backgroundColor: 'red', padding: '10px', }; export const text = { color: 'white', fontSize: '16px', }; // MyComponent.js import React from 'react'; import { container, text } from './styles'; function MyComponent() { return ( <div> <p>Hello, world!</p> </div> ); }
3. Use variables for reusable styles
If you have styles that are reused across multiple components, consider defining them as variables and importing them where needed. This promotes consistency and makes it easier to update styles across the application. For example:
// styles.js export const primaryButton = { backgroundColor: 'blue', color: 'white', padding: '10px', }; // Button.js import React from 'react'; import { primaryButton } from './styles'; function Button({ children }) { return <button>{children}</button>; }
4. Avoid using inline styles for complex styles
While inline styles can be useful for simple styles, they can quickly become hard to manage for complex styles. In such cases, it's better to use CSS files or CSS-in-JS libraries like styled-components or CSS Modules. These approaches provide better separation of concerns and allow you to write CSS styles in a more familiar syntax. For example:
// Button.js import React from 'react'; import styled from 'styled-components'; const StyledButton = styled.button` background-color: blue; color: white; padding: 10px; `; function Button({ children }) { return {children}; }
Related Article: How to Fetch a Parameter Value From a Query String in React
5. Consider using CSS preprocessors
If you prefer using CSS preprocessors like Sass or Less, you can still use them with inline styles in React JS. Preprocessors offer additional features like variables, mixins, and nesting, which can make your styles more efficient and maintainable. You can compile your preprocessed styles to JavaScript objects and use them as inline styles. For example:
// styles.scss $primary-color: blue; .container { background-color: $primary-color; padding: 10px; } .text { color: white; font-size: 16px; }
// MyComponent.js import React from 'react'; import styles from './styles.scss'; function MyComponent() { return ( <div> <p>Hello, world!</p> </div> ); }