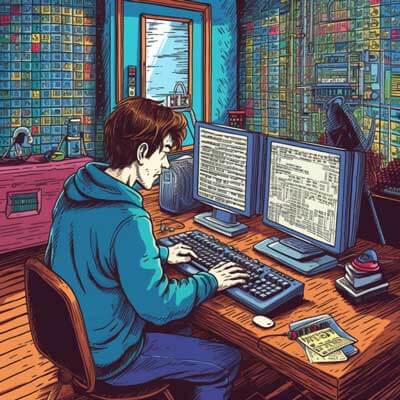
Table of Contents
There are several ways to implement a sleep function in Node.js to introduce a delay in the execution of code. In this answer, we will explore two popular approaches: using the built-in setTimeout function and utilizing the newer async/await syntax.
Using setTimeout
The setTimeout function is a built-in Node.js function that executes a specified function or a piece of code after a specified delay. We can leverage this function to implement a sleep-like behavior in our code.
Here's an example of how to use setTimeout to introduce a delay in Node.js:
function sleep(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async function main() { console.log('Before sleep'); await sleep(2000); // Sleep for 2 seconds console.log('After sleep'); } main();
In the above code, we define a sleep
function that returns a Promise. This Promise is resolved after a specified number of milliseconds using setTimeout
. We then use the await
keyword to asynchronously pause the execution of the code for the specified duration.
When main
is invoked, it logs "Before sleep" to the console, waits for 2 seconds using the sleep
function, and then logs "After sleep" to the console.
This approach is useful when you want to introduce a delay in an asynchronous context, such as waiting for an API response or simulating a time-consuming task.
Related Article: How To Check Node.Js Version On Command Line
Using async/await with a Timer Function
Another way to implement sleep in Node.js JavaScript is by utilizing the newer async/await syntax along with a timer function. This approach allows for a more intuitive and synchronous-looking code structure.
Here's an example of how to implement sleep using async/await and a timer function:
function sleep(ms) { return new Promise(resolve => { const timer = setTimeout(() => { clearTimeout(timer); // Clear the timer resolve(); }, ms); }); } async function main() { console.log('Before sleep'); await sleep(2000); // Sleep for 2 seconds console.log('After sleep'); } main();
In the above code, the sleep
function returns a Promise that resolves after the specified number of milliseconds. Inside the Promise, we create a timer using setTimeout
and clear the timer using clearTimeout
once the timeout has completed.
The main
function uses the await
keyword to pause the execution of the code for 2 seconds using the sleep
function.
This approach is similar to the previous one but provides more control over the timer and allows for customization if needed.
Alternative Ideas
While the above approaches are commonly used to introduce a sleep-like behavior in Node.js, it's worth mentioning that introducing unnecessary delays in your code can impact performance and should be used sparingly. In many cases, there are alternative solutions that can achieve the desired outcome without the need for sleep.
For example, if you're waiting for an API response, it's generally better to use callbacks, Promises, or async/await syntax to handle the asynchronous nature of the operation rather than introducing a sleep delay.
Similarly, if you're writing tests, using testing frameworks like Mocha or Jest provides built-in mechanisms for handling asynchronous code and waiting for assertions to complete.
Best Practices
When implementing a sleep-like behavior in Node.js, consider the following best practices:
1. Use the appropriate approach based on your use case. If you're working with asynchronous code, the setTimeout
approach or async/await with a timer function is suitable. If you're working with synchronous code, consider using other control flow mechanisms like loops or conditionals.
2. Avoid introducing unnecessary delays in your code. Sleep-like behavior should be used judiciously and only when necessary. Consider alternative solutions that align with Node.js's non-blocking nature.
3. Keep the sleep duration reasonable. Longer sleep durations can impact the responsiveness of your application. Consider optimizing your code or utilizing other asynchronous patterns to reduce the need for long delays.
4. Use proper error handling. When using async/await or Promises, make sure to handle any potential errors that may occur during the sleep operation.