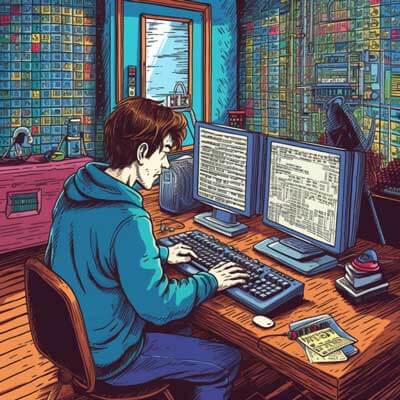
Table of Contents
Overview of Express
Express is a web application framework for Node.js designed to simplify the process of building web applications and APIs. It provides a robust set of features for web and mobile applications, making it a popular choice among developers. Express enables developers to create dynamic web applications with a minimum amount of code, offering a wide range of middleware to handle various tasks such as authentication, logging, and error handling.
One of the key benefits of using Express is its minimalistic architecture, which allows developers to add only the components they need. This flexibility makes it suitable for both small and large applications. Additionally, Express is built on top of Node.js, which means it inherits the non-blocking, event-driven nature of Node.js, allowing for high performance and scalability.
Related Article: How to Use Force and Legacy Peer Deps in Npm
Installing Node.js and npm
Node.js is a JavaScript runtime built on Chrome's V8 JavaScript engine. To use Express, you first need to have Node.js and npm (Node Package Manager) installed on your machine. npm comes bundled with Node.js, so installing Node.js will also install npm.
To install Node.js, visit the official Node.js website at https://nodejs.org and download the installer for your operating system. Follow the installation instructions provided on the website.
To verify the installation, open your terminal or command prompt and run the following commands:
node -v npm -v
These commands will display the installed versions of Node.js and npm, confirming that the installation was successful.
Creating a New Project
After installing Node.js and npm, the next step is to create a new project directory. It is common practice to organize your projects in a dedicated directory. You can create a new project folder using the terminal or command prompt.
Navigate to the location where you want to create your project and run the following command:
mkdir my-express-app cd my-express-app
This command creates a new directory called my-express-app
and navigates into it.
Adding Express to package.json
To manage your project dependencies, you need a package.json
file, which holds essential information about your project and its dependencies. You can create this file by running the following command in your project directory:
npm init -y
The -y
flag automatically answers "yes" to all prompts, creating a package.json
file with default settings. After running this command, open the package.json
file in a text editor. You will see a structure that includes metadata about your project, such as the name, version, and description.
Next, you will add Express as a dependency to this file. You could do this manually, but the next step simplifies the process.
Related Article: How to Install Specific npm Package Versions
Running npm install Command
To install Express, you can run the following command:
npm install express
This command will download the Express package and its dependencies from the npm registry and add it to your package.json
file under the dependencies
section. After the installation completes, your package.json
will look something like this:
{ "name": "my-express-app", "version": "1.0.0", "description": "", "main": "index.js", "dependencies": { "express": "^4.17.1" }, "scripts": { "test": "echo \"Error: no test specified\" && exit 1" }, "author": "", "license": "ISC" }
Verifying Express Installation
To ensure that Express has been installed correctly, you can check the node_modules
directory in your project folder. This directory contains all the installed packages. You can also verify the installation by running the following command:
npm list express
This command will display the installed version of Express. If you see something like express@4.17.1
, it confirms that Express is installed and ready to use.
Using Express Generator
For developers who prefer a scaffolded approach, the Express Generator is a command-line tool that helps create a basic Express application structure. To install the generator globally, run the following command:
npm install -g express-generator
Once installed, you can create a new Express application by running:
express my-express-app
This command creates a new directory called my-express-app
with a basic structure and necessary files. Navigate into the newly created directory:
cd my-express-app
You will notice that a number of files and folders have been created, including app.js
, routes
, and views
.
Setting Up a Basic Express Application
To start developing your Express application, you need to install the dependencies listed in the package.json
file. Run the following command:
npm install
After the installation, you can start the application by adding a simple server setup in the app.js
file. Open app.js
in a text editor and look for the following code:
const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('Hello World!'); }); app.listen(port, () => { console.log(`Example app listening at http://localhost:${port}`); });
This code creates a basic Express server that listens on port 3000 and responds with "Hello World!" when visiting the root URL.
Related Article: How to Use Luxon with npm
Configuring Express Middleware
Middleware functions are essential in Express applications. They are functions that execute during the request-response cycle and can modify the request, response, or end the request-response cycle. To use middleware, you can define it in your app.js
file.
For example, to use the built-in middleware to serve static files, add the following line before your routes:
app.use(express.static('public'));
This line tells Express to serve static files from the public
directory. You can create this directory and add some static assets like images or stylesheets.
Starting the Application with npm start
To run your Express application, you can start it using npm. First, modify the scripts
section in your package.json
to include a start script:
"scripts": { "start": "node app.js" }
Now you can start your application by running:
npm start
You should see a message indicating that your app is listening on port 3000. You can visit http://localhost:3000 in your web browser to see your application in action.
Managing Express as a Dependency
Managing dependencies is crucial for maintaining your application. The package.json
file keeps track of the packages you have installed, including Express. To ensure that other developers can install the same dependencies, you should always include the package.json
file in your version control system.
Whenever you want to update your dependencies, including Express, you can run:
npm update
This command updates all your packages to their latest versions based on the versioning rules defined in your package.json
.
Updating Express Version
To update Express to a specific version, you can specify the version number in the install command. For example, to update to version 4.17.3, run:
npm install express@4.17.3
This command will update Express to the specified version and modify the package.json
file accordingly.
To check for outdated packages, you can run:
npm outdated
This command will list all the outdated packages in your project, including Express, if applicable.
Related Article: How to Fix npm Unsupported Engine Issues
Uninstalling Express Package
If you need to remove Express from your project, you can do so with the following command:
npm uninstall express
This command will remove Express from the node_modules
directory and update your package.json
file to reflect the change. After uninstalling, if you run npm list express
, you should see a message indicating that Express is no longer installed.
Differences Between npm Install Options
When installing packages with npm, there are various options you can use with the npm install
command. The most common options include:
1. npm install <package>
: Installs the latest version of the specified package.
2. npm install <package>@<version>
: Installs a specific version of the package.
3. npm install --save <package>
: Installs the package and adds it as a dependency in the package.json
file.
4. npm install --save-dev <package>
: Installs the package as a development dependency, meaning it is only required during development and not in production.
Understanding these options helps you manage your project's dependencies better.
Global Installation of Express
While Express is typically installed locally within a project, you can also install it globally. This is useful if you want to use the Express Generator or other command-line tools related to Express. To install Express globally, run:
npm install -g express
This command installs Express globally on your machine. You can verify the installation by running:
npm list -g express
It's important to note that installing Express globally does not make it available to your local project. Each project should have its own instance of Express installed locally to ensure compatibility and avoid conflicts with other projects.