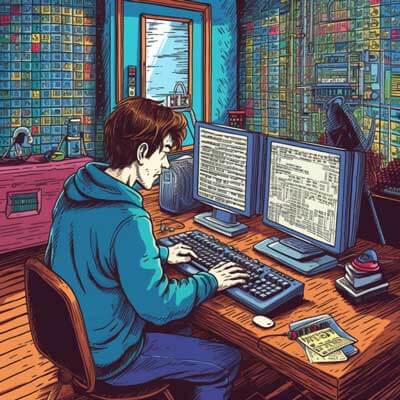
Table of Contents
PyTorch is a popular open-source machine learning library that is widely used for deep learning tasks. In this article, we will walk through the step-by-step process of installing PyTorch on different operating systems, configuring it for GPU usage, and various other installation methods. Additionally, we will explore how to set up a virtual environment, install PyTorch dependencies, and troubleshoot installation issues. Once PyTorch is installed, we will delve into different aspects of using PyTorch, including writing your first PyTorch program, handling data formats, training and validating models, and more. Let's get started!
Installing PyTorch on Windows
To install PyTorch on Windows, follow these steps:
1. Open a web browser and go to the official PyTorch website: https://pytorch.org/
2. Select the appropriate options for your system configuration, such as your Python version and CUDA support.
3. Copy the installation command provided on the website based on your preferences.
4. Open a command prompt or PowerShell window with administrative privileges.
5. Paste the installation command into the command prompt and press Enter to execute it.
6. Wait for the installation process to complete.
Here's an example of the installation command for PyTorch with CUDA support on Python 3.8:
pip install torch==1.7.1+cu110 torchvision==0.8.2+cu110 torchaudio==0.7.2 -f https://download.pytorch.org/whl/torch_stable.html
Related Article: An Introduction to PyTorch
Installing PyTorch on macOS
To install PyTorch on macOS, follow these steps:
1. Open a web browser and go to the official PyTorch website: https://pytorch.org/
2. Select the appropriate options for your system configuration, such as your Python version and CUDA support.
3. Copy the installation command provided on the website based on your preferences.
4. Open a terminal window.
5. Paste the installation command into the terminal and press Enter to execute it.
6. Wait for the installation process to complete.
Here's an example of the installation command for PyTorch on macOS with Python 3.8:
pip3 install torch torchvision torchaudio
Installing PyTorch on Linux
To install PyTorch on Linux, follow these steps:
1. Open a web browser and go to the official PyTorch website: https://pytorch.org/
2. Select the appropriate options for your system configuration, such as your Python version and CUDA support.
3. Copy the installation command provided on the website based on your preferences.
4. Open a terminal.
5. Paste the installation command into the terminal and press Enter to execute it.
6. Wait for the installation process to complete.
Here's an example of the installation command for PyTorch on Linux with Python 3.8:
pip3 install torch torchvision torchaudio
Configuring PyTorch for GPU
To configure PyTorch for GPU usage, follow these steps:
1. Make sure you have a compatible GPU and the necessary drivers installed on your system.
2. Install the CUDA Toolkit, if it is not already installed. You can download it from the NVIDIA website: https://developer.nvidia.com/cuda-toolkit
3. Install the cuDNN library, if it is not already installed. You can download it from the NVIDIA website: https://developer.nvidia.com/cudnn
4. After installing CUDA and cuDNN, PyTorch should automatically detect and utilize your GPU for accelerated computations.
Related Article: Creating Custom Datasets and Dataloaders in PyTorch
Checking PyTorch Installation
To check if PyTorch is installed correctly, open a Python interpreter or a Jupyter Notebook and run the following code:
import torch print(torch.__version__) print(torch.cuda.is_available())
If PyTorch is installed correctly, you should see the version number and whether CUDA is available on your system.
Setting Up a Virtual Environment
Setting up a virtual environment is a good practice to isolate your PyTorch installation and its dependencies from other projects. To set up a virtual environment, follow these steps:
1. Open a terminal or command prompt.
2. Install the virtualenv package, if it is not already installed, by running the following command:
pip install virtualenv
3. Create a new virtual environment by running the following command:
virtualenv myenv
Replace "myenv" with the desired name for your virtual environment.
4. Activate the virtual environment by running the appropriate command for your operating system:
On Windows:
myenv\Scripts\activate
On macOS and Linux:
source myenv/bin/activate
5. Install PyTorch and other dependencies within the virtual environment using the appropriate installation method.
6. When you're done working with PyTorch, deactivate the virtual environment by running the following command:
deactivate
Installing PyTorch Dependencies
PyTorch has a few dependencies that need to be installed before using it. Here are the common dependencies and how to install them:
- NumPy: A useful numerical computing library. Install it using the following command:
pip install numpy
- Matplotlib: A plotting library for visualizing data. Install it using the following command:
pip install matplotlib
- SciPy: A library for scientific and technical computing. Install it using the following command:
pip install scipy
These are just a few examples of PyTorch dependencies. Depending on your specific use case, you may need to install additional libraries.
Using Conda for PyTorch Installation
Conda is a package management system that is commonly used for scientific computing and data science tasks. To install PyTorch using Conda, follow these steps:
1. Install Miniconda or Anaconda, if it is not already installed. You can download it from the official website: https://docs.conda.io/en/latest/miniconda.html
2. Open a terminal or command prompt.
3. Create a new conda environment by running the following command:
conda create --name myenv
Replace "myenv" with the desired name for your environment.
4. Activate the conda environment by running the following command:
conda activate myenv
5. Install PyTorch and other dependencies using the following command:
conda install pytorch torchvision torchaudio -c pytorch
Related Article: Data Loading and Preprocessing in PyTorch
Installing PyTorch with Pip
Pip is the default package manager for Python and can be used to install PyTorch. To install PyTorch with pip, follow these steps:
1. Open a terminal or command prompt.
2. Create a new virtual environment, if desired.
3. Activate the virtual environment, if applicable.
4. Run the following command to install PyTorch:
pip install torch torchvision torchaudio
Installing PyTorch with Anaconda
Anaconda is a popular distribution for Python that includes many scientific computing packages. To install PyTorch with Anaconda, follow these steps:
1. Install Anaconda, if it is not already installed. You can download it from the official website: https://www.anaconda.com/products/individual
2. Open the Anaconda Navigator or Anaconda Prompt.
3. Create a new conda environment, if desired.
4. Activate the conda environment, if applicable.
5. Use the following command to install PyTorch:
conda install pytorch torchvision torchaudio -c pytorch
Installing PyTorch with Docker
Docker provides a convenient way to package and distribute software applications. To install PyTorch with Docker, follow these steps:
1. Install Docker, if it is not already installed. You can download it from the official website: https://www.docker.com/products/docker-desktop
2. Open a terminal or command prompt.
3. Run the following command to pull the PyTorch Docker image:
docker pull pytorch/pytorch
4. Run the following command to start a PyTorch container:
docker run -it --rm pytorch/pytorch
This will start an interactive session inside the PyTorch container.
Troubleshooting PyTorch Installation
If you encounter any issues during the PyTorch installation process, consider the following troubleshooting steps:
1. Make sure you have the latest version of Python installed.
2. Check if you have the necessary system requirements, such as GPU drivers and CUDA.
3. Verify that the installation command is correct and matches your system configuration.
4. Check if there are any conflicting packages or dependencies in your environment.
5. Refer to the official PyTorch documentation and community forums for troubleshooting guides and solutions.
Related Article: Comparing PyTorch and TensorFlow
Uninstalling PyTorch
To uninstall PyTorch, follow these steps:
1. Open a terminal or command prompt.
2. Activate the virtual environment, if applicable.
3. Run the following command to uninstall PyTorch:
pip uninstall torch torchvision torchaudio
Upgrading PyTorch
To upgrade PyTorch to the latest version, follow these steps:
1. Open a terminal or command prompt.
2. Activate the virtual environment, if applicable.
3. Run the following command to upgrade PyTorch:
pip install --upgrade torch torchvision torchaudio
Using PyTorch in Jupyter Notebook
To use PyTorch in Jupyter Notebook, follow these steps:
1. Install Jupyter Notebook, if it is not already installed. You can install it using pip or conda.
2. Open a terminal or command prompt and navigate to the desired directory.
3. Activate the virtual environment, if applicable.
4. Run the following command to start Jupyter Notebook:
jupyter notebook
5. In the Jupyter Notebook interface, create a new notebook and import the PyTorch library to start using it:
import torch
Using PyTorch in Visual Studio Code
To use PyTorch in Visual Studio Code, follow these steps:
1. Install Visual Studio Code, if it is not already installed. You can download it from the official website: https://code.visualstudio.com/
2. Install the Python extension for Visual Studio Code.
3. Open Visual Studio Code and open a Python file or create a new one.
4. Activate the virtual environment, if applicable.
5. Import the PyTorch library and start using it in your Python code:
import torch
Related Article: PyTorch Application in Natural Language Processing
Writing Your First PyTorch Program
To write your first PyTorch program, follow these steps:
1. Create a new Python file or Jupyter Notebook.
2. Import the necessary PyTorch modules:
import torch import torch.nn as nn import torch.optim as optim
3. Define your model architecture using PyTorch's nn.Module class. Here's an example of a simple neural network with one hidden layer:
class Net(nn.Module): def __init__(self): super(Net, self).__init__() self.fc1 = nn.Linear(784, 64) self.fc2 = nn.Linear(64, 10) def forward(self, x): x = x.view(-1, 784) x = torch.relu(self.fc1(x)) x = self.fc2(x) return x
4. Instantiate your model:
model = Net()
5. Define your loss function and optimizer:
criterion = nn.CrossEntropyLoss() optimizer = optim.SGD(model.parameters(), lr=0.001, momentum=0.9)
6. Load your training data and labels.
7. Train your model by iterating over the training data, performing forward and backward passes, and updating the model parameters.
8. Evaluate your model on the test data and calculate performance metrics.
This is just a basic example to get you started. PyTorch offers many more features and capabilities for building and training complex models.
Using PyTorch Models from the Model Zoo
PyTorch provides a Model Zoo, which is a collection of pre-trained models for various computer vision and natural language processing tasks. To use a pre-trained model from the Model Zoo, follow these steps:
1. Import the necessary PyTorch modules:
import torch import torchvision.models as models import torchvision.transforms as transforms
2. Load the pre-trained model:
model = models.resnet18(pretrained=True)
3. Preprocess your input data:
transform = transforms.Compose([ transforms.Resize(256), transforms.CenterCrop(224), transforms.ToTensor(), transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225]) ])
4. Apply the preprocessing transform to your input data:
input_data = transform(input_data)
5. Perform inference using the pre-trained model:
output = model(input_data)
6. Process the output to get the desired predictions or results.
Handling Different Data Formats in PyTorch
PyTorch provides various data loading utilities and classes to handle different data formats. Here are a few examples:
- CSV: You can use the torch.utils.data.Dataset
class to create a custom dataset class that reads data from a CSV file. Implement the __getitem__
method to load and preprocess the data.
- Image: PyTorch provides the torchvision.datasets.ImageFolder
class to load image data from a directory structure. The directory structure should be organized such that each subdirectory represents a class.
- Numpy: You can convert a Numpy array to a PyTorch tensor using the torch.from_numpy
function.
- Text: PyTorch provides the torchtext
library for handling text data. It includes various utilities for tokenizing, preprocessing, and batching text data.
These are just a few examples of how to handle different data formats in PyTorch. Depending on your specific use case, you may need to implement custom data loading and preprocessing logic.
Preprocessing Data for PyTorch
Preprocessing data is an important step in machine learning tasks. PyTorch provides various tools and utilities to preprocess data. Here are a few examples:
- Normalization: You can use the torchvision.transforms.Normalize
class to normalize input data using mean and standard deviation values.
- Data augmentation: PyTorch provides the torchvision.transforms
module, which includes various transformations for data augmentation, such as random cropping, flipping, and rotation.
- Resizing and cropping: PyTorch provides the torchvision.transforms.Resize
and `torchvision.transforms.Center
Related Article: GPU Acceleration Implementation with PyTorch
Additional Resources
- Installing PyTorch with Anaconda