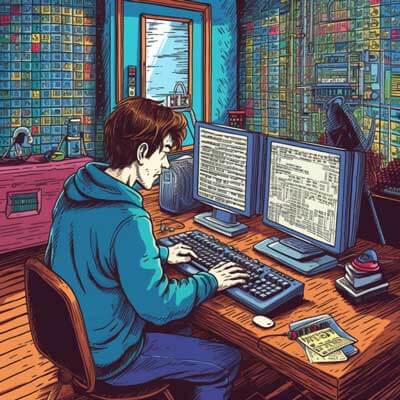
Table of Contents
Overview of Joining Sequences
Joining sequences is the process of combining multiple sequences into a single sequence. Sequences can be lists, tuples, or strings, and joining them allows us to manipulate and work with the data more efficiently. This practical guide will explore different methods for joining sequences in Python, including concatenation, merging, and combining.
Related Article: How to Use Class And Instance Variables in Python
Concatenating Sequences in Python
Concatenation is the process of combining two or more sequences together to create a new sequence. In Python, we can concatenate sequences using the +
operator. Let's take a look at an example:
list1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = list1 + list2 print(concatenated_list)
Output:
[1, 2, 3, 4, 5, 6]
In this example, we concatenate two lists list1
and list2
using the +
operator and store the result in concatenated_list
. The resulting list contains all the elements from both lists in the order they were concatenated.
Merging Sequences
Merging sequences involves combining multiple sequences while preserving the order of the elements. In Python, we can achieve this using the zip()
function. The zip()
function takes two or more sequences as input and returns an iterator that generates tuples containing elements from each sequence. Let's see an example:
list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list(zip(list1, list2)) print(merged_list)
Output:
[(1, 4), (2, 5), (3, 6)]
In this example, we merge list1
and list2
using the zip()
function. The resulting list contains tuples where each tuple contains corresponding elements from both lists.
Combining Sequences
Combining sequences involves creating a new sequence by combining elements from multiple sequences in a specific pattern or order. Python provides several methods for combining sequences, such as using list comprehensions or generator expressions. Let's explore an example using list comprehension:
list1 = [1, 2, 3] list2 = [4, 5, 6] combined_list = [a + b for a, b in zip(list1, list2)] print(combined_list)
Output:
[5, 7, 9]
In this example, we combine list1
and list2
by adding corresponding elements using list comprehension. The resulting list contains the sum of elements at each corresponding index.
Related Article: How To Use If-Else In a Python List Comprehension
Code Snippet: Joining Lists in Python
list1 = [1, 2, 3] list2 = [4, 5, 6] joined_list = list1 + list2 print(joined_list)
Output:
[1, 2, 3, 4, 5, 6]
Code Snippet: Concatenating Sequences
list1 = [1, 2, 3] list2 = [4, 5, 6] concatenated_list = list1 + list2 print(concatenated_list)
Output:
[1, 2, 3, 4, 5, 6]
Code Snippet: Merging Multiple Sequences
list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list(zip(list1, list2)) print(merged_list)
Output:
[(1, 4), (2, 5), (3, 6)]
Code Snippet: Combining Lists in Python
list1 = [1, 2, 3] list2 = [4, 5, 6] combined_list = [a + b for a, b in zip(list1, list2)] print(combined_list)
Output:
[5, 7, 9]
Related Article: Working with Numpy Concatenate
Additional Resources
- Python Sequence Operations