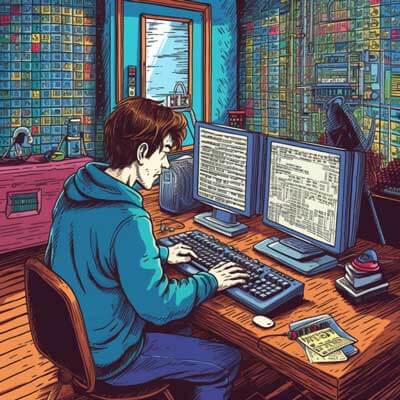
Table of Contents
Overview of Global npm Packages
Global npm packages are tools that can be used across various projects on a system. These packages are installed in a central location on your machine, which allows you to access them from the command line anywhere. This is particularly useful for packages that provide command-line tools, such as npm, gulp, or typescript. Unlike local packages, which are specific to a project, global packages facilitate access to utility scripts and other tools without needing to install them in every single project.
When a package is installed globally, it is stored in a default directory which depends on the operating system. For example, on Unix-based systems, the global packages are typically installed in /usr/local/lib/node_modules
, while on Windows, they are stored in %APPDATA%\npm\node_modules
. This centralized management helps streamline workflows, especially for developers who frequently use the same tools across different projects.
Related Article: How to Use Luxon with npm
Installing Global Packages
To install a global package, the npm command line tool is employed with the -g
or --global
flag. This flag indicates that the package should be installed globally rather than locally within a specific project. The syntax for installing a global package is as follows:
npm install -g <package-name>
For example, to install the typescript package globally, you would run:
npm install -g typescript
After executing this command, the TypeScript compiler will be available for use in any directory on your machine. This alleviates the need to install TypeScript for each project separately.
Listing Global Packages
To view the global packages currently installed on your system, the following command can be used:
npm list -g --depth=0
This command lists all globally installed packages at the top level, meaning it won't delve into their dependencies. The --depth=0
flag ensures that only the main packages are displayed, which simplifies the output. The output will show you the names and versions of the packages, making it easy to keep track of what is installed.
Filtering the Global Package List
Filtering the global package list can be accomplished using various command-line tools in conjunction with the npm list
command. For example, if you want to find a specific package, you can pipe the output to grep:
npm list -g --depth=0 | grep <package-name>
For instance, if you are looking for the typescript package, the command would be:
npm list -g --depth=0 | grep typescript
This command will return only the line containing the TypeScript package, helping you quickly determine if it's installed.
Related Article: How to Fix npm err cb never called
Finding Global Package Locations
Identifying where global packages are installed can be done using the npm root
command with the -g
flag:
npm root -g
This command will return the path to the global node_modules directory. Knowing the location is beneficial for troubleshooting or when you need to manually manipulate files or directories associated with a package.
Checking Global Package Versions
Verifying the version of a globally installed package helps ensure compatibility and functionality. The command for checking a specific package version is as follows:
npm list -g <package-name>
For example, to check the version of the typescript package, the command would be:
npm list -g typescript
This will display the version number of TypeScript if it is installed globally, allowing you to confirm you are using the desired version.
Updating Global Packages
Keeping global packages up to date is crucial for security and functionality. To update a global package, the following command is used:
npm update -g <package-name>
For instance, to update TypeScript to the latest version, the command would be:
npm update -g typescript
To update all globally installed packages at once, you can run:
npm update -g
This command will systematically check for updates to all global packages and apply them as necessary.
Removing Global Packages
When a global package is no longer needed, it can be removed using the uninstall command. The syntax is:
npm uninstall -g <package-name>
For example, to uninstall the typescript package, you would use:
npm uninstall -g typescript
This command will remove TypeScript from the global scope, freeing up space and reducing clutter in your development environment.
Related Article: How to Fix Yarn v4 npm Publish 401 Error
Managing Global Package Permissions
Managing permissions for global packages can become important when facing permission errors during installation or removal. On Unix-based systems, global packages might require elevated privileges for installation. If permission errors occur, prefixing the npm command with sudo
can resolve these issues:
sudo npm install -g <package-name>
However, using sudo
can lead to complications with file permissions. An alternative approach is to configure npm to use a directory in the user's home folder for global installations, avoiding permission issues altogether. This can be done by creating a directory and updating the npm configuration:
mkdir ~/.npm-globalnpm config set prefix '~/.npm-global'
After doing this, you will need to add the following line to your profile file (like .bashrc
or .zshrc
):
export PATH=~/.npm-global/bin:$PATH
This ensures that your shell recognizes the location of globally installed packages.
Global Package Configuration
Configuring global packages can enhance your workflow and tailor the tools to your specific needs. npm allows you to set various configuration options that apply globally. One common configuration is to set the default registry for package installations:
npm config set registry <registry-url>
Additionally, you can view the current configuration settings using:
npm config list
This command will display all the configuration settings, including global package configurations. Adjusting these settings can optimize your development environment, making it more aligned with your requirements.