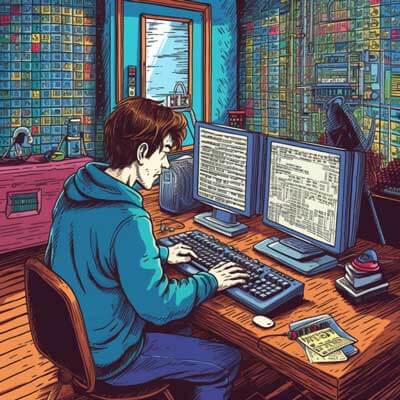
Table of Contents
Overview of PrimeVue Components
PrimeVue is a popular UI component library for Vue.js applications. It provides a wide range of rich and customizable components that help developers build user interfaces more quickly. Each component is designed to be easy to use and can be styled to match the desired look of the application. Components include buttons, data tables, charts, dialogs, and much more. The library is built with accessibility in mind, ensuring that applications are usable by everyone.
When working with PrimeVue, it is important to understand the structure of its components. Each component can be imported individually, allowing developers to include only what is necessary for their application. This modular approach helps to keep the bundle size smaller, which can improve load times and performance.
Related Article: How to Fix Communication with the API in NPM
Prerequisites for Installation
Before proceeding with the installation of PrimeVue components, certain prerequisites must be fulfilled. First, ensure that Node.js and npm (Node Package Manager) are installed on your system. These tools allow you to manage dependencies in your JavaScript applications.
To check if Node.js and npm are installed, you can run the following commands in your terminal:
node -v npm -v
If both commands return version numbers, you are ready to proceed. If not, download and install Node.js from the official website, which will also install npm.
Additionally, a Vue.js project should be set up. You can create a new Vue project using Vue CLI. If Vue CLI is not installed, you can install it with the following command:
npm install -g @vue/cli
To create a new Vue project, run:
vue create my-project
After the project is created, navigate into the project directory:
cd my-project
With these prerequisites in place, the installation of PrimeVue components can begin.
Finding PrimeVue Components
PrimeVue offers a variety of components, and each component has its own documentation page. To find the components, visit the official PrimeVue website. The documentation includes a list of all available components along with examples of how to use them.
When selecting components, consider the needs of your application. For instance, if you require a datatable, you can look for the DataTable component. If you need a modal dialog, explore the Dialog component. Each component's documentation provides additional information on props, events, and methods, which are crucial for proper implementation.
Configuring package.json for PrimeVue
Configuring your package.json
file is essential for managing dependencies. When you install PrimeVue, it will automatically update this file to include PrimeVue and its dependencies. The package.json
file contains metadata about the project and its dependencies.
To manually add PrimeVue, you can modify the package.json
file directly. Open the package.json
file in a text editor and add PrimeVue under the dependencies
section:
"dependencies": { "vue": "^3.0.0", "primevue": "^3.0.0", "primeicons": "^4.0.0" }
Ensure that the version numbers match the latest versions available. This setup allows npm to manage the installation and updates of the packages.
Related Article: How to Use npm with Next.js
Installing PrimeVue Components via npm
With the configuration complete, the next step is to install PrimeVue components using npm. Run the following command in the terminal to install PrimeVue, along with the PrimeIcons library, which is often used for icons in PrimeVue components:
npm install primevue primeicons
This command will download the necessary packages and update your node_modules
directory, where npm stores installed packages. It will also update your package.json
file with the new dependencies.
After the installation, you can verify that PrimeVue has been added to your project by checking the node_modules
directory or looking at the package.json
file.
Importing PrimeVue Components
Importing components into your Vue application is essential for using them. PrimeVue components can be imported in the main entry file of your Vue application, typically main.js
.
To import a component, use the following syntax:
import { createApp } from 'vue'; import App from './App.vue'; import PrimeVue from 'primevue/config'; import 'primeicons/primeicons.css'; import 'primevue/resources/themes/saga-blue/theme.css'; // Choose a theme import 'primevue/resources/primevue.min.css'; // Core css const app = createApp(App); app.use(PrimeVue); app.mount('#app');
In this example, PrimeVue is imported and passed to the Vue application using app.use(PrimeVue)
. Additionally, the necessary CSS files for PrimeVue and PrimeIcons are included to ensure that the components are styled correctly.
Registering PrimeVue Components
After importing PrimeVue into your application, individual components need to be registered before they can be used in your templates. This can be done globally or locally.
For global registration, you can register components in the main.js
file. Here is how to register a Button component:
import Button from 'primevue/button'; app.component('Button', Button);
Now, the Button component can be used in any template within the application.
For local registration, you can register components directly within a Vue component. This is beneficial when the component is only used in a specific file. Here is an example:
<template> <Button label="Click Me" /> </template> <script> import Button from 'primevue/button'; export default { components: { Button } } </script>
In this case, the Button component is imported and registered within the scope of the component, allowing its use only in that specific context.
Troubleshooting Component Visibility
If components do not appear as expected in your application, several common issues could be the cause.
First, ensure that the component is correctly registered, either globally or locally. Check for any typographical errors in the component name used in the template. The name used in the template must match the name used during registration.
Next, verify that the necessary CSS files are included in your project. Missing CSS can lead to components not rendering properly. If styles are missing, check that the paths to the CSS files in main.js
are correct.
Additionally, check your console for any errors. If there are issues with the component or its dependencies, the console may provide hints on what is wrong. Addressing errors listed in the console can often resolve visibility issues.
Related Article: How to Fix npm err cb never called
Manual Installation Without CLI
If there is a need to install PrimeVue components without using the command line interface (CLI), it can be done manually by downloading the necessary files. Navigate to the PrimeVue GitHub repository or its official website to download the required components.
Once downloaded, place the files in your project directory, typically within a libs
or vendor
folder. You will then need to import these files in your main.js
file similar to how you would if they were installed via npm:
import Button from './libs/primevue/button'; import './libs/primevue/resources/primevue.min.css';
This approach may be less common but can be useful in scenarios where a network connection is limited or when dealing with specific build processes.