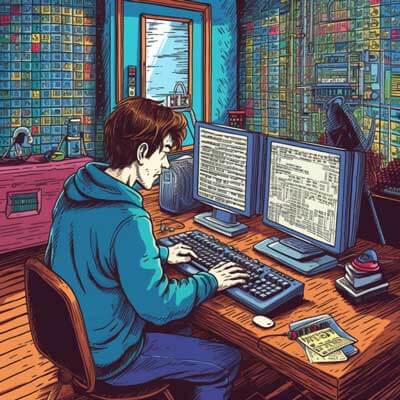
Table of Contents
React Router is a popular library for handling routing in React applications. While React Router provides declarative routing through its components, there are situations where you may need to navigate programmatically. In this guide, we will explore different approaches to navigate using React Router programmatically.
Using the useHistory Hook
React Router provides a useHistory hook that allows you to access the history object. The history object contains various methods that can be used to navigate programmatically. Here's an example of how to use the useHistory hook to navigate to a different route:
import { useHistory } from 'react-router-dom'; function MyComponent() { const history = useHistory(); const navigateToAbout = () => { history.push('/about'); }; return ( <button>Go to About</button> ); }
In the example above, we import the useHistory hook from 'react-router-dom' and use it to access the history object. We then define a function called navigateToAbout that uses the history.push method to navigate to the '/about' route when a button is clicked.
Related Article: How to Reverse a String In-Place in JavaScript
Using the withRouter Higher-Order Component
If you are using a class component or if you prefer to use higher-order components (HOCs), you can use the withRouter HOC provided by React Router. The withRouter HOC injects the history object as a prop to the wrapped component, allowing you to access it and navigate programmatically. Here's an example:
import { withRouter } from 'react-router-dom'; class MyComponent extends React.Component { navigateToAbout = () => { this.props.history.push('/about'); }; render() { return ( <button>Go to About</button> ); } } export default withRouter(MyComponent);
In the example above, we import the withRouter HOC from 'react-router-dom' and wrap our component with it using the export default withRouter(MyComponent) syntax. This injects the history object as a prop to the MyComponent class, allowing us to access it in the navigateToAbout method and navigate programmatically.
Using the Redirect Component
Another approach to navigate programmatically is to use the Redirect component provided by React Router. The Redirect component allows you to conditionally redirect to a different route based on certain conditions. Here's an example:
import { Redirect } from 'react-router-dom'; class MyComponent extends React.Component { constructor(props) { super(props); this.state = { redirectToAbout: false }; } navigateToAbout = () => { this.setState({ redirectToAbout: true }); }; render() { if (this.state.redirectToAbout) { return ; } return ( <button>Go to About</button> ); } }
In the example above, we import the Redirect component from 'react-router-dom' and define a constructor that sets an initial state variable redirectToAbout to false. When the navigateToAbout method is called, we update the state to true, which causes the component to render the Redirect component and navigate to the '/about' route.
Best Practices
When navigating programmatically using React Router, it is important to consider best practices to ensure a smooth user experience. Here are a few best practices to keep in mind:
- Avoid excessive use of programmatic navigation: While programmatic navigation can be useful in certain scenarios, it is generally recommended to rely on declarative routing using React Router's components as much as possible. This helps to maintain a clear and predictable flow in your application.
- Use appropriate lifecycle methods or hooks: When navigating programmatically in class components, it is important to consider the appropriate lifecycle method to perform the navigation. For example, if you need to navigate after a component has mounted, you can use the componentDidMount lifecycle method or the useEffect hook in functional components.
- Handle redirects and errors gracefully: When navigating programmatically, it is important to handle redirects and errors gracefully. React Router provides various methods and components to handle redirects and errors, such as the Redirect component and the useHistory hook's replace method.
- Test your navigation logic: As with any functionality in your application, it is important to thoroughly test your programmatic navigation logic. Use unit tests or integration tests to ensure that your navigation code behaves as expected and does not introduce any bugs or unexpected behavior.