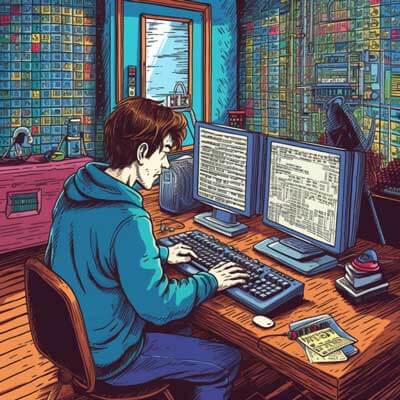
Table of Contents
Overview of CSS Optimization and Its Importance
CSS optimization involves refining and enhancing CSS files to improve load times, reduce file sizes, and ensure smooth rendering of web pages. Optimized CSS can significantly boost website performance by minimizing render-blocking resources, thereby enhancing user experience. A well-optimized CSS file reduces the amount of data transferred over the network, which is especially critical for mobile devices or slower connections.
When CSS manages styles for multiple components, the size of these files can grow, leading to potential performance bottlenecks. Optimization ensures that only the necessary styles are loaded, improving the overall responsiveness of web applications.
Related Article: How to Use the Webpack CLI Option -d
Benefits of Using a Plugin for CSS Asset Management
Using a plugin for CSS asset management streamlines the optimization process. Plugins automate tasks like minification, concatenation, and tree shaking, allowing developers to focus on writing code instead of managing assets manually.
In Webpack, plugins such as MiniCssExtractPlugin
and OptimizeCSSAssetsPlugin
play a crucial role. The MiniCssExtractPlugin
extracts CSS into separate files, which can be loaded asynchronously, while OptimizeCSSAssetsPlugin
optimizes the extracted CSS by minimizing it.
These plugins significantly reduce the complexity of configuring CSS optimization, providing built-in functionalities that enhance development efficiency.
Implementing Code Splitting for CSS Assets
Code splitting allows developers to divide their CSS files into smaller chunks that can be loaded on demand. This technique improves the initial load time of applications by only loading the CSS required for the current view.
To implement code splitting, configure Webpack as follows:
// webpack.config.jsconst MiniCssExtractPlugin = require('mini-css-extract-plugin');module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist', }, module: { rules: [ { test: /\.css$/, use: [MiniCssExtractPlugin.loader, 'css-loader'], }, ], }, plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', }), ],};
In this configuration, the MiniCssExtractPlugin
creates separate CSS files for each entry point. When the application runs, only the CSS needed for the currently active view is loaded.
Exploring Tree Shaking for Unused CSS
Tree shaking is a technique used to eliminate unused CSS, reducing file sizes and improving performance. This process is particularly useful in large applications where styles may be defined but not utilized.
Webpack performs tree shaking by analyzing the code and determining which CSS rules are not being applied. To enable tree shaking for CSS, ensure you are using CSS Modules or a similar approach that allows Webpack to understand which styles are actually being used.
Example of CSS modules:
/* styles.module.css */.button { background-color: blue;}.hidden { display: none;}
// Component.jsimport styles from './styles.module.css';const Button = () => { return <button className={styles.button}>Click Me</button>;};
In this setup, only the styles used in the component will be included in the final bundle, minimizing unused CSS.
Related Article: How to Use Extract Text Webpack Plugin
Minification vs. Compression in CSS Assets
Minification and compression are two distinct processes that help reduce CSS file sizes. Minification involves removing whitespace, comments, and unused code from the CSS file, while compression further reduces the file size by using algorithms to encode the data more efficiently.
Minification can be achieved in Webpack using the OptimizeCSSAssetsPlugin
:
// webpack.config.jsconst OptimizeCSSAssetsPlugin = require('optimize-css-assets-webpack-plugin');module.exports = { optimization: { minimizer: [new OptimizeCSSAssetsPlugin({})], },};
Compression is typically handled at the server level, where files are served using Gzip or Brotli compression. To enable Gzip compression in an Express server, for example, use the compression
middleware:
// server.jsconst express = require('express');const compression = require('compression');const app = express();app.use(compression());app.use(express.static('dist'));
This way, both minification and compression work together to significantly reduce the size of CSS assets.
Configuring Asset Management for CSS Optimization
Proper configuration of asset management in Webpack is essential for optimizing CSS. This involves setting up loaders and plugins to handle CSS files correctly.
Here’s an example configuration that includes the necessary loaders and plugins for optimal CSS asset management:
// webpack.config.jsconst MiniCssExtractPlugin = require('mini-css-extract-plugin');const OptimizeCSSAssetsPlugin = require('optimize-css-assets-webpack-plugin');module.exports = { module: { rules: [ { test: /\.css$/, use: [ MiniCssExtractPlugin.loader, 'css-loader', 'postcss-loader', // Optional: for autoprefixing ], }, ], }, optimization: { minimizer: [new OptimizeCSSAssetsPlugin({})], }, plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', }), ],};
This configuration sets up a smooth pipeline for handling CSS files, ensuring they are extracted, optimized, and ready for production.
Tools for Analyzing CSS Bundle Size
Analyzing the size of CSS bundles is critical for understanding performance. Tools like webpack-bundle-analyzer
provide visual representations of your bundle sizes, helping identify large dependencies or unused styles.
To use webpack-bundle-analyzer
, install it via npm:
npm install --save-dev webpack-bundle-analyzer
Then, update your Webpack configuration:
// webpack.config.jsconst { BundleAnalyzerPlugin } = require('webpack-bundle-analyzer');module.exports = { plugins: [ new BundleAnalyzerPlugin(), ],};
After running the build, a report will be generated that illustrates the size of each module within the bundle. This information helps in making informed decisions about CSS optimization.
Best Practices for CSS Optimization in Projects
Following best practices for CSS optimization can lead to significant performance improvements. Here are key practices to consider:
- Use a CSS preprocessor like SASS or LESS for better organization and modularity.
- Implement CSS Modules to scope styles locally and avoid conflicts.
- Avoid using !important
to maintain specificity and clarity in styles.
- Use shorthand properties to reduce the amount of CSS written.
- Regularly audit your CSS for unused styles and remove them.
Incorporating these practices helps maintain a clean and efficient codebase.
Related Article: How to Set Webpack Target to Node.js
Integrating Critical CSS for Enhanced Performance
Critical CSS is the practice of inlining essential styles that are required for the initial rendering of a web page. This reduces render-blocking resources, leading to faster page loads.
To implement critical CSS in Webpack, consider using the critters
plugin:
npm install --save-dev critters-webpack-plugin
Then, add it to your Webpack configuration:
// webpack.config.jsconst Critters = require('critters-webpack-plugin');module.exports = { plugins: [ new Critters(), ],};
This plugin automatically extracts critical CSS and inlines it in the HTML, ensuring that the essential styles are loaded quickly.
Caching Strategies for Improved CSS Load Times
Caching is crucial for improving load times, as it allows browsers to store CSS files locally, reducing the need for repeated downloads.
To implement caching, use versioning in file names. This ensures that when you update CSS files, the browser fetches the new version:
// webpack.config.jsconst MiniCssExtractPlugin = require('mini-css-extract-plugin');module.exports = { plugins: [ new MiniCssExtractPlugin({ filename: '[name].[contenthash].css', // Use content hash for cache busting }), ],};
Setting cache headers on your server configuration also helps. For example, in an Nginx server configuration:
location ~* \.(css)$ { expires 1y; add_header Cache-Control "public, no-transform";}
This configuration tells the browser to cache CSS files for one year.
Performance Tuning Techniques for CSS Assets
Performance tuning for CSS assets involves various techniques aimed at reducing load times and improving rendering speed. Key methods include:
- Minifying CSS to reduce file size.
- Using a Content Delivery Network (CDN) to deliver CSS files faster.
- Loading CSS asynchronously to prevent render-blocking.
- Using media queries to conditionally load styles based on device characteristics.
Implementing these techniques provides a more responsive user experience.
Post-Processing Methods for CSS Optimization
Post-processing involves applying additional transformations to CSS after it has been written. Tools like PostCSS can be configured to enhance your CSS automatically.
To use PostCSS, install the necessary packages:
npm install --save-dev postcss postcss-loader autoprefixer
Then, set up PostCSS in your Webpack configuration:
// webpack.config.jsmodule.exports = { module: { rules: [ { test: /\.css$/, use: [ MiniCssExtractPlugin.loader, 'css-loader', { loader: 'postcss-loader', options: { postcssOptions: { plugins: [ require('autoprefixer'), // Automatically adds vendor prefixes ], }, }, }, ], }, ], },};
PostCSS improves cross-browser compatibility and can optimize CSS further.
Related Article: How to Use Webpack Manifest Plugin
Hot Module Replacement for CSS Development
Hot Module Replacement (HMR) allows developers to replace modules in a running application without a full reload. This feature is beneficial for CSS, as it enables live updates without losing application state.
To enable HMR for CSS in Webpack, update the configuration as follows:
// webpack.config.jsconst webpack = require('webpack');module.exports = { devServer: { hot: true, // Enable hot module replacement }, plugins: [ new webpack.HotModuleReplacementPlugin(), ],};
In development mode, changes to CSS files will be instantly reflected in the browser, improving the development workflow.
Analyzing Bundle Size with Built-in Tools
Webpack provides built-in tools for analyzing bundle sizes. The --profile
and --json
flags can be used when running the build command:
webpack --profile --json > stats.json
This generates a stats.json
file containing detailed information about the bundle. You can then use tools like webpack-bundle-analyzer
or source-map-explorer
to visualize the data.
Responsive Design Considerations in CSS Optimization
Responsive design is essential for modern web applications. CSS optimization for responsive design involves using media queries effectively and avoiding fixed sizes.
Using relative units like percentages, em
, and rem
ensures that styles adapt to various screen sizes. Additionally, using CSS Grid and Flexbox provides flexibility in layout design, enhancing responsiveness.
Example of a responsive layout using Flexbox:
.container { display: flex; flex-wrap: wrap;}.item { flex: 1 1 200px; /* Flexible item with a base width */}
This layout adapts to screen sizes, ensuring that items reflow appropriately.
Common Mistakes in Optimizing CSS Assets
Several common mistakes can hinder CSS optimization efforts. Avoid these pitfalls:
- Failing to minify CSS files, leading to larger file sizes.
- Not using a CSS preprocessor, which can lead to disorganized code.
- Overusing !important
, which complicates styles and overrides.
- Ignoring browser compatibility, leading to inconsistent experiences across devices.
Related Article: How to Use Environment Variables in Webpack
Additional Resources
- Benefits of Using Webpack Plugins for CSS