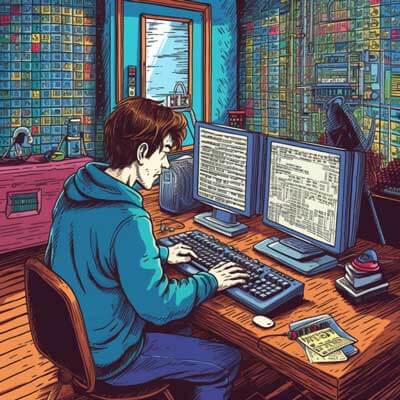
Table of Contents
There are several ways to pretty print nested dictionaries in Python. In this answer, we will explore two popular methods: using the pprint
module and using the json
module.
Using the pprint module
The pprint
module in Python provides a way to pretty print data structures, including nested dictionaries. It formats the output in a more readable and organized way.
To use the pprint
module, you first need to import it:
import pprint
Once imported, you can use the pprint
function to pretty print a nested dictionary:
data = { 'name': 'John', 'age': 30, 'address': { 'street': '123 Main St', 'city': 'New York', 'state': 'NY' } } pprint.pprint(data)
This will produce the following output:
{'address': {'city': 'New York', 'state': 'NY', 'street': '123 Main St'}, 'age': 30, 'name': 'John'}
As you can see, the output is nicely formatted with indentation and line breaks, making it easier to read and understand the structure of the nested dictionary.
Related Article: How to Use Redis with Django Applications
Using the json module
Another way to pretty print nested dictionaries in Python is by using the json
module. The json
module provides a dump
function that can be used to serialize a dictionary into a JSON formatted string.
To pretty print a nested dictionary using the json
module, you can follow these steps:
1. Import the json
module:
import json
2. Serialize the dictionary into a JSON formatted string using the json.dumps
function with the indent
parameter set to the desired number of spaces for indentation:
data = { 'name': 'John', 'age': 30, 'address': { 'street': '123 Main St', 'city': 'New York', 'state': 'NY' } } pretty_json = json.dumps(data, indent=4)
3. Print the pretty JSON string:
print(pretty_json)
This will produce the following output:
{ "name": "John", "age": 30, "address": { "street": "123 Main St", "city": "New York", "state": "NY" } }
As you can see, the output is formatted with indentation and newlines, making it easier to read and understand the structure of the nested dictionary.
Best Practices
Related Article: Python Super Keyword Tutorial
When pretty printing nested dictionaries in Python, it's important to keep the following best practices in mind:
1. Use the pprint
module if you need a quick and easy way to pretty print nested dictionaries. It provides a simple interface and automatically handles the formatting for you.
2. If you need more control over the formatting, such as custom indentation or sorting of keys, you can use the json
module. This allows you to serialize the dictionary into a JSON formatted string and gives you more flexibility in how the output is displayed.
3. Consider using the indent
parameter in the json.dumps
function to control the number of spaces used for indentation. This can help make the output more readable and organized.
4. If you are working with large or complex nested dictionaries, consider using a combination of both the pprint
and json
modules. Use pprint
for a quick overview of the structure, and use json
for more detailed control over the formatting.