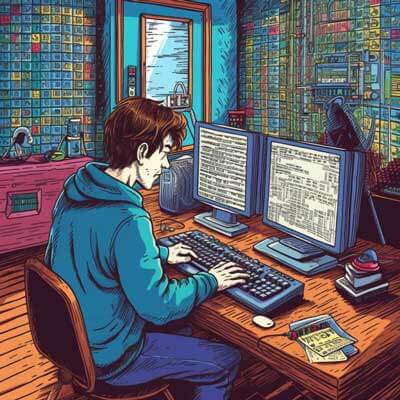
To read an external local JSON file in JavaScript, you can use the XMLHttpRequest object or the fetch() function. Both methods allow you to retrieve the contents of a JSON file and process it within your JavaScript code.
Using XMLHttpRequest
1. Create a new instance of the XMLHttpRequest object:
var xhr = new XMLHttpRequest();
2. Open a GET request to the JSON file:
xhr.open('GET', 'path/to/file.json', true);
3. Set the response type to ‘json’:
xhr.responseType = 'json';
4. Define a callback function to handle the response:
xhr.onload = function() { if (xhr.status === 200) { var data = xhr.response; // Process the JSON data here } }; 5. Send the request: ```javascript xhr.send();
Related Article: nvm (Node Version Manager): Install Guide & Cheat Sheet
Using fetch()
1. Use the fetch() function to make a GET request to the JSON file:
fetch('path/to/file.json') .then(function(response) { return response.json(); }) .then(function(data) { // Process the JSON data here });
Best Practices
– Always handle errors and check the status code of the response to ensure that the request was successful before processing the JSON data.
– Use the response.json() method to parse the JSON data automatically.
– Encapsulate the code for reading the JSON file in a function to promote reusability.
Alternative Ideas
– If you are using a modern JavaScript framework like React or Angular, you can leverage their built-in mechanisms for fetching and processing JSON data. These frameworks often provide utilities or hooks that simplify the process.
– Consider using a library like axios or jQuery.ajax() to handle the AJAX request for you. These libraries provide additional features and can make the code more concise.
Related Article: How to Use the forEach Loop with JavaScript
Example: Fetching and Displaying JSON Data
fetch('path/to/file.json') .then(function(response) { return response.json(); }) .then(function(data) { displayData(data); }) .catch(function(error) { console.log('An error occurred:', error); }); function displayData(data) { // Display the JSON data on the page }
In this example, the fetch() function is used to retrieve the JSON data from the specified file. The response.json() method is then called to parse the data, and the resulting JavaScript object is passed to the displayData() function for further processing.