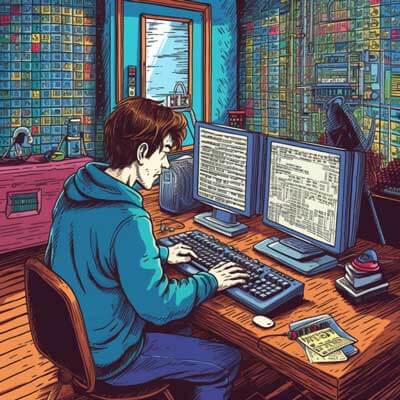
Table of Contents
To remove a key from a Python dictionary, you can use the del
statement or the pop()
method. The del
statement removes the key-value pair from the dictionary, while the pop()
method not only removes the key-value pair but also returns the value associated with the key.
Using the del statement
To remove a key from a dictionary using the del
statement, you need to specify the key that you want to remove. Here's the syntax:
del dictionary_name[key]
Here's an example that demonstrates how to remove a key from a dictionary using the del
statement:
# Create a dictionary my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} # Remove the 'age' key del my_dict['age'] # Print the updated dictionary print(my_dict)
Output:
{'name': 'John', 'city': 'New York'}
In this example, the del
statement is used to remove the 'age' key from the my_dict
dictionary.
Related Article: How to Use Stripchar on a String in Python
Using the pop() method
The pop()
method allows you to remove a key from a dictionary and also retrieve the corresponding value. Here's the syntax:
dictionary_name.pop(key, default_value)
The pop()
method takes two arguments: the key of the item to remove, and an optional default value to return if the key is not found in the dictionary.
Here's an example that demonstrates how to remove a key from a dictionary using the pop()
method:
# Create a dictionary my_dict = {'name': 'John', 'age': 30, 'city': 'New York'} # Remove the 'age' key and get the value age = my_dict.pop('age') # Print the updated dictionary print(my_dict) # Print the value of the 'age' key print(age)
Output:
{'name': 'John', 'city': 'New York'} 30
In this example, the pop()
method is used to remove the 'age' key from the my_dict
dictionary and retrieve its value. The value is then stored in the age
variable.
Handling key errors
Related Article: Python Operators Tutorial & Advanced Examples
Both the del
statement and the pop()
method raise a KeyError
if the specified key is not found in the dictionary. To prevent this error, you can use the in
operator to check if the key exists in the dictionary before attempting to remove it.
Here's an example that demonstrates how to handle key errors:
# Create a dictionary my_dict = {'name': 'John', 'city': 'New York'} # Check if the 'age' key exists before removing it if 'age' in my_dict: del my_dict['age'] # Print the updated dictionary print(my_dict)
Output:
{'name': 'John', 'city': 'New York'}
In this example, the in
operator is used to check if the 'age' key exists in the my_dict
dictionary before attempting to remove it with the del
statement.