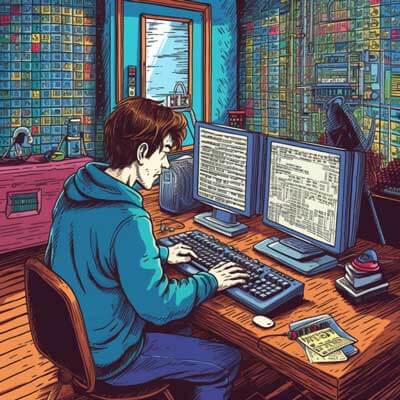
Table of Contents
Option 1: Using Set
One of the simplest and most efficient ways to remove duplicate values from a JavaScript array is by using the Set object. The Set object only allows unique values, so by converting the array to a Set and then back to an array, we can automatically remove any duplicates.
Here's an example of how to remove duplicates using Set:
const array = [1, 2, 3, 3, 4, 5, 5, 6]; const uniqueArray = [...new Set(array)]; console.log(uniqueArray);
In this example, we have an array with duplicate values: [1, 2, 3, 3, 4, 5, 5, 6]. By converting it to a Set using the spread operator ([...new Set(array)]), we automatically remove the duplicates. The resulting unique array is then logged to the console.
This method is particularly useful when dealing with arrays of primitive values like numbers or strings. However, it may not work as expected when working with arrays of objects or arrays containing complex data structures.
Related Article: How to Create Responsive Images in Next.JS
Option 2: Using Filter and indexOf
Another approach to removing duplicate values from a JavaScript array is by using the filter method in combination with the indexOf method. This method works by iterating over each element of the array and filtering out any duplicates based on their index.
Here's an example of how to remove duplicates using filter and indexOf:
const array = [1, 2, 3, 3, 4, 5, 5, 6]; const uniqueArray = array.filter((value, index) => array.indexOf(value) === index); console.log(uniqueArray);
In this example, the filter method is used to iterate over each element of the array. The callback function checks the index of each element using indexOf and keeps only the elements that have the same index as their first occurrence. The resulting unique array is then logged to the console.
This method works well with all types of arrays, including arrays of objects or arrays containing complex data structures. However, it may have a higher time complexity compared to using Set, especially for larger arrays.
Best Practices
Related Article: How To Disable & Enable a Submit Button With jQuery
When removing duplicate values from a JavaScript array, it's important to consider the following best practices:
1. Use the Set object when dealing with arrays of primitive values like numbers or strings. This method is simple, efficient, and ensures uniqueness automatically.
2. For arrays of objects or arrays containing complex data structures, use the filter method in combination with indexOf. This method allows for more flexibility and can handle any type of array.
3. If you need to preserve the original order of the elements, use the filter method in combination with indexOf. This method keeps the first occurrence of each element while removing the duplicates.
4. Be cautious when working with large arrays, as the filter and indexOf method may have a higher time complexity compared to using Set. Consider the performance implications and choose the method that best suits your specific use case.
Overall, removing duplicate values from a JavaScript array can be achieved in a straightforward manner using either the Set object or the filter method in combination with indexOf. Choose the method that best fits your requirements and consider the complexity and performance implications when working with larger arrays or more complex data structures.