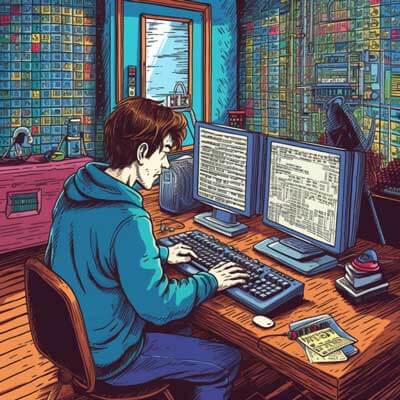
Table of Contents
Removing spaces from a string is a common task in Javascript programming. There are several ways to accomplish this. In this answer, we will explore two different approaches.
Approach 1: Using the replace() method with a regular expression
One straightforward way to remove spaces from a string in Javascript is by using the replace()
method with a regular expression. The regular expression / /g
matches all occurrences of a space in a string.
Here's an example code snippet that demonstrates this approach:
let str = "Hello, world! This is a string with spaces."; let result = str.replace(/ /g, ""); console.log(result);
In this example, the replace()
method is called on the str
variable with the regular expression / /g
as the first argument and an empty string ""
as the second argument. The g
flag in the regular expression ensures that all occurrences of spaces are replaced, not just the first one.
The output of the above code will be:
Hello,world!Thisisastringwithspaces.
This approach is simple and works well when you want to remove all spaces from a string. However, it will also remove spaces within words if present.
Related Article: How To Add A Class To A Given Element In Javascript
Approach 2: Using the split() and join() methods
Another way to remove spaces from a string in Javascript is by using the split()
and join()
methods. The split()
method splits a string into an array of substrings based on a specified separator, and the join()
method joins the elements of an array into a string using a specified separator.
Here's an example code snippet that demonstrates this approach:
let str = "Hello, world! This is a string with spaces."; let result = str.split(" ").join(""); console.log(result);
In this example, the split(" ")
method is called on the str
variable with a space as the separator. This splits the string into an array of substrings at each occurrence of a space. Then, the join("")
method is called on the resulting array with an empty string as the separator. This joins the elements of the array into a string, effectively removing the spaces.
The output of the above code will be:
Hello,world!Thisisastringwithspaces.
This approach is useful when you want to remove only spaces and preserve other characters in the string.
Alternative Ideas
- If you want to remove leading and trailing spaces but preserve the spaces within the string, you can use the trim()
method in combination with either of the above approaches. The trim()
method removes whitespace from both ends of a string.
let str = " Hello, world! "; let result = str.trim().replace(/ /g, ""); console.log(result);
The output of the above code will be:
Hello,world!
- If you want to remove all whitespace characters (including spaces, tabs, and newlines) from a string, you can modify the regular expression in the first approach. For example, the regular expression /s/g
matches all whitespace characters.
let str = "Hello,\tworld!\nThis is a string with spaces."; let result = str.replace(/\s/g, ""); console.log(result);
The output of the above code will be:
Hello,world!Thisisastringwithspaces.
Best Practices
- When removing spaces from a string, it is important to consider the context and requirements of your specific use case. Depending on the situation, you may need to preserve certain spaces or whitespace characters. Choose the approach that best suits your needs.
- If you are working with user input or external data, always validate and sanitize the input before removing spaces. This helps prevent potential security vulnerabilities and unexpected behavior.
- When using the replace()
method with a regular expression, be mindful of the pattern you use. Regular expressions can be useful but also complex. Test and verify your regular expressions to ensure they match the desired spaces accurately.
- Use meaningful variable names to enhance code readability and maintainability. Instead of generic names like str
, consider using more descriptive names that reflect the purpose of the string.