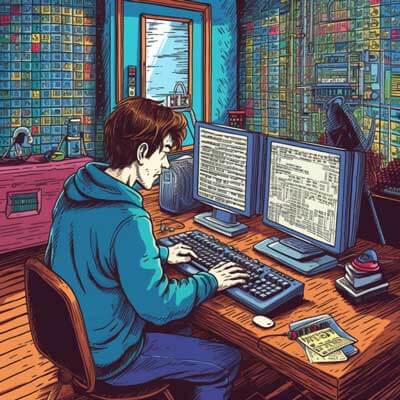
Table of Contents
How does NPM relates to React.js?
npm stands for Node Package Manager. It is a package manager for JavaScript that allows developers to easily download, install, and manage JavaScript packages and libraries. npm is included with Node.js, which is a JavaScript runtime environment that allows developers to run JavaScript on the server-side.
React.js can be installed and managed using npm. By using npm, developers can easily add React.js as a dependency in their project and ensure that the correct version of React.js is installed. npm also provides a way to manage other dependencies and libraries used in a React.js project, making it easier to maintain and update the project over time.
In addition to managing dependencies, npm also provides a command-line interface that allows developers to run scripts, build projects, and perform other tasks related to their React.js project. This makes it a useful tool for managing the development workflow and automating repetitive tasks.
Related Article: Adding a Newline in the JSON Property Render with ReactJS
What role does Node.js play in rendering React.js code with npm?
Node.js plays a crucial role in rendering React.js code with npm. Node.js is a JavaScript runtime environment that allows developers to run JavaScript on the server-side. It provides a server-side execution environment for JavaScript code, which is a key requirement for rendering React.js code on the server.
One of the main benefits of rendering React.js code on the server is improved performance and search engine optimization (SEO). When a user requests a web page, the server can pre-render the React components on the server and send the pre-rendered HTML to the client. This allows the client to receive a fully rendered page without waiting for the JavaScript code to execute on the client-side. It also allows search engines to crawl and index the content of the web page, improving its visibility in search results.
Node.js, together with npm, provides the necessary tools and libraries to enable server-side rendering of React.js code. npm allows developers to install and manage the dependencies of the server-side rendering process, such as libraries for rendering React components on the server and managing the application state.
Node.js also provides the infrastructure for running the server-side rendering process. Developers can write JavaScript code that runs on the server using Node.js, and they can use npm to install and manage the necessary libraries and tools.
Overall, Node.js and npm are essential tools in rendering React.js code on the server, enabling improved performance and SEO for React.js applications.
Setting Up Your Development Environment
Before you can start rendering React.js code with npm, you need to set up your development environment. Here are the steps to set up your development environment for React.js:
1. Install Node.js: Node.js is required to run npm and execute JavaScript code on the server-side. You can download and install Node.js from the official website (https://nodejs.org). Choose the version that matches your operating system and follow the installation instructions.
2. Verify Node.js installation: Once Node.js is installed, open a terminal or command prompt and run the following command to verify that Node.js is installed correctly:
node -v
This command should display the version number of Node.js. If you see the version number, Node.js is installed correctly.
3. Create a new project: Create a new directory for your React.js project and navigate to it in the terminal or command prompt. Run the following command to initialize a new npm project:
npm init
This command will prompt you to enter some information about your project, such as the project name and version. You can press Enter to accept the default values or enter your own values.
4. Install React.js and dependencies: Once the npm project is initialized, you can install React.js and its dependencies. Run the following command to install React.js:
npm install react react-dom
This command will download and install React.js and react-dom packages from the npm registry.
5. Verify React.js installation: After the installation is complete, create a new file named index.js in your project directory and add the following code:
import React from 'react'; import ReactDOM from 'react-dom'; ReactDOM.render( <h1>Hello, world!</h1>, document.getElementById('root') );
This code imports React and ReactDOM from the installed packages and uses ReactDOM.render() to render a simple "Hello, world!" message to the DOM.
6. Create an HTML file: Create a new file named index.html in your project directory and add the following code:
<!DOCTYPE html> <html> <head> <title>React App</title> </head> <body> <div id="root"></div> <script src="index.js"></script> </body> </html>
This code creates a basic HTML file with a div element with the id "root", which will be used as the container for the React.js application. It also includes the index.js file using a script tag.
7. Start the development server: To view your React.js application in the browser, you need to start a development server. Run the following command to start the development server:
npx webpack serve --mode development
This command will start the webpack development server and automatically open your React.js application in the browser. Any changes you make to the code will be automatically reloaded in the browser.
Congratulations! You have successfully set up your development environment for rendering React.js code with npm. You can now start building and rendering React components.
Installing React.js with npm
To install React.js with npm, follow these steps:
1. Create a new directory: Create a new directory for your React.js project.
2. Navigate to the project directory: Open a terminal or command prompt and navigate to the project directory.
3. Initialize a new npm project: Run the following command to initialize a new npm project:
npm init
This command will prompt you to enter some information about your project, such as the project name and version. You can press Enter to accept the default values or enter your own values.
4. Install React.js: Run the following command to install React.js:
npm install react react-dom
This command will download and install the latest stable versions of React.js and react-dom from the npm registry.
5. Verify React.js installation: After the installation is complete, you can verify that React.js is installed correctly by importing it in your JavaScript code. Create a new JavaScript file in your project directory and add the following code:
import React from 'react'; import ReactDOM from 'react-dom'; console.log(React); console.log(ReactDOM);
Save the file and run it using Node.js:
node your-file.js
If there are no errors, React.js is installed correctly and you can start using it in your project.
Congratulations! You have successfully installed React.js with npm. You can now start building React components and rendering them in your application.
Related Article: How to Implement Hover State in ReactJS with Inline Styles
Creating a Basic React Component
In React.js, a component is a reusable piece of UI that can be rendered to the DOM. Components are the building blocks of React applications and can be composed together to create complex UIs.
To create a basic React component, follow these steps:
1. Create a new JavaScript file: Create a new JavaScript file in your project directory and give it a descriptive name, such as "MyComponent.js".
2. Import React: In the JavaScript file, import the React library:
import React from 'react';
3. Create a functional component: In React, a functional component is a simple JavaScript function that returns JSX code. Define a new function with a name that represents your component:
function MyComponent() { return ( <div> <h1>Hello, World!</h1> </div> ); }
4. Export the component: To make the component available for other parts of your application, export it:
export default MyComponent;
5. Use the component: In another JavaScript file, import the component and use it in your application:
import React from 'react'; import ReactDOM from 'react-dom'; import MyComponent from './MyComponent'; ReactDOM.render( <MyComponent />, document.getElementById('root') );
In this example, we import the MyComponent component and render it to the DOM using ReactDOM.render().
Congratulations! You have created a basic React component. You can now use this component in your React application and render it to the DOM.
Rendering React Components
In React.js, rendering components to the DOM is a fundamental part of building React applications. To render a React component, follow these steps:
1. Import React and ReactDOM: In your JavaScript file, import the React and ReactDOM libraries:
import React from 'react'; import ReactDOM from 'react-dom';
2. Create a component: Define a new component using a functional or class component. For example, we will create a functional component that displays a simple message:
function MyComponent() { return ( <div> <h1>Hello, World!</h1> </div> ); }
3. Render the component: Use the ReactDOM.render() function to render the component to the DOM. Pass the component as the first argument and the DOM element where you want to render the component as the second argument:
ReactDOM.render( <MyComponent />, document.getElementById('root') );
In this example, we render the MyComponent component to the DOM. The component will be rendered inside the element with the id "root".
Congratulations! You have successfully rendered a React component to the DOM. You can now build more complex components and render them in your React application.
Using JSX in React.js
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. It is used in React.js to describe the structure and appearance of the user interface.
To use JSX in React.js, follow these steps:
1. Create a new JavaScript file: Create a new JavaScript file in your project directory and give it a descriptive name, such as "App.js".
2. Import React: In the JavaScript file, import the React library:
import React from 'react';
3. Write JSX code: In React, you can write JSX code to describe the structure and appearance of the UI. JSX looks similar to HTML, but it is not actually HTML. It is a combination of JavaScript and XML-like syntax. Here's an example of JSX code:
function App() { return ( <div> <h1>Hello, World!</h1> <p>This is a React application.</p> </div> ); }
4. Export the component: To make the component available for other parts of your application, export it:
export default App;
5. Use the component: In another JavaScript file, import the component and use it in your application:
import React from 'react'; import ReactDOM from 'react-dom'; import App from './App'; ReactDOM.render( <App />, document.getElementById('root') );
In this example, we import the App component and render it to the DOM using ReactDOM.render().
Congratulations! You have successfully used JSX in React.js. You can now write HTML-like code within your JavaScript files to describe the structure and appearance of your React components.
Transpiling JSX with Babel
JSX is a syntax extension for JavaScript that allows you to write HTML-like code within JavaScript. However, JSX is not supported by all browsers out of the box. To ensure that JSX code can be executed in all browsers, it needs to be transpiled into regular JavaScript code.
Babel is a popular JavaScript compiler that can transpile JSX code into regular JavaScript code. To transpile JSX with Babel, follow these steps:
1. Install Babel: Install Babel and the necessary presets and plugins. Run the following command in your project directory:
npm install --save-dev @babel/core @babel/preset-react
2. Create a Babel configuration file: Create a file named ".babelrc" in your project directory and add the following code:
{ "presets": ["@babel/preset-react"] }
This configuration file tells Babel to use the "@babel/preset-react" preset, which includes the necessary plugins to transpile JSX code.
3. Transpile JSX code: Use Babel to transpile your JSX code. For example, if you have a file named "App.js" that contains JSX code, you can transpile it using the following command:
npx babel App.js --out-file transpiled.js
This command will transpile the "App.js" file and save the transpiled code to "transpiled.js".
Congratulations! You have successfully transpiled JSX code with Babel. You can now use JSX in your React.js applications and ensure that it is compatible with all browsers.
Related Article: Handling Routing in React Apps with React Router
Bundling React.js Code with Webpack
Webpack is a popular module bundler for JavaScript applications. It is commonly used in React.js development to bundle and optimize the JavaScript code, CSS, and other assets of a React.js application.
To bundle React.js code with Webpack, follow these steps:
1. Install Webpack: Install Webpack and the necessary loaders. Run the following command in your project directory:
npm install --save-dev webpack webpack-cli webpack-dev-server babel-loader css-loader style-loader
2. Create a Webpack configuration file: Create a file named "webpack.config.js" in your project directory and add the following code:
const path = require('path'); module.exports = { entry: './src/index.js', output: { path: path.resolve(__dirname, 'dist'), filename: 'bundle.js', }, module: { rules: [ { test: /\.(js|jsx)$/, exclude: /node_modules/, use: 'babel-loader', }, { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, devServer: { contentBase: path.resolve(__dirname, 'dist'), port: 3000, }, };
This configuration file tells Webpack how to bundle your React.js code. It specifies the entry point of your application, the output path and file name of the bundle, and the loaders to use for different file types.
3. Create an index.html file: Create an HTML file named "index.html" in your project directory and add the following code:
<!DOCTYPE html> <html> <head> <title>React App</title> </head> <body> <div id="root"></div> <script src="bundle.js"></script> </body> </html>
This code creates a basic HTML file with a div element with the id "root", which will be used as the container for the React.js application. It also includes the "bundle.js" file using a script tag.
4. Create an index.js file: Create a JavaScript file named "index.js" in your project directory and add the following code:
import React from 'react'; import ReactDOM from 'react-dom'; import App from './App'; ReactDOM.render( <App />, document.getElementById('root') );
In this example, we import the App component and render it to the DOM using ReactDOM.render().
5. Build the bundle: Use Webpack to build the bundle. Run the following command in your project directory:
npx webpack
This command will bundle your React.js code and its dependencies into a single file named "bundle.js" in the "dist" directory.
6. Start the development server: To view your React.js application in the browser, you need to start a development server. Run the following command:
npx webpack serve --mode development
This command will start the webpack development server and automatically open your React.js application in the browser. Any changes you make to the code will be automatically reloaded in the browser.
Congratulations! You have successfully bundled your React.js code with Webpack. You can now build and optimize your React.js application using Webpack.
Working with Node.js in React.js
Node.js plays a crucial role in React.js development, especially when it comes to server-side rendering, API integration, and setting up a development environment.
Here are some common use cases for working with Node.js in React.js:
1. Server-side rendering: React.js can be rendered on the server using Node.js. This allows the server to send pre-rendered HTML to the client, improving performance and search engine optimization. Node.js provides the server-side execution environment for running React.js code and generating the pre-rendered HTML.
2. API integration: React.js applications often need to communicate with APIs to fetch data and update the user interface. Node.js can be used to build server-side APIs that the React.js application can interact with. Node.js provides a robust and scalable runtime for building server-side APIs, and it can easily integrate with popular frameworks and libraries for handling API requests and responses.
3. Development environment: Node.js is commonly used in the development environment for React.js applications. It provides tools and libraries for running a development server, automating build processes, and managing dependencies. Node.js can be used with npm, the package manager for JavaScript, to install and manage the dependencies of a React.js project.
4. Tooling and build processes: Node.js is often used in the tooling and build processes of React.js applications. It provides a runtime for running build scripts, transpiling code, bundling assets, and optimizing the application for production. Node.js can be used with popular build tools like Webpack, Babel, and ESLint to enhance the development workflow and automate repetitive tasks.
What are the benefits of using React.js for frontend development?
React.js offers several benefits for frontend development:
1. Component-Based Architecture: React.js follows a component-based architecture, where each UI component is a self-contained unit that can be composed together to create complex UIs. This modular approach makes it easier to build and maintain large-scale applications, as components can be reused and tested independently.
2. Virtual DOM: React.js uses a virtual DOM to efficiently update the UI. Instead of directly manipulating the browser's DOM, React.js updates a virtual representation of the DOM and then efficiently applies the necessary changes to the actual DOM. This approach minimizes the number of DOM manipulations, resulting in better performance and a smoother user experience.
3. Reusability: React.js promotes reusability by encouraging the creation of reusable UI components. These components can be easily shared and reused across different projects, reducing development time and effort.
4. Performance Optimization: React.js includes built-in performance optimization features, such as the ability to memoize components and implement shouldComponentUpdate lifecycle method. These features help improve the performance of React.js applications by minimizing unnecessary re-renders and updates.
5. Large and Active Community: React.js has a large and active community of developers, which means there are plenty of resources, tutorials, and libraries available for developers to leverage. This community also provides support and contributes to the continuous improvement of React.js.
What are UI components in React.js?
In React.js, UI components are the building blocks of the user interface. A UI component represents a specific part of the UI and can be composed together to create complex UIs. UI components can be as simple as a button or as complex as a form with multiple fields.
React.js promotes the reusability of UI components, which means that components can be easily shared and reused across different parts of the application. This reusability is achieved through a combination of encapsulation, composition, and props.
Encapsulation refers to the practice of encapsulating the implementation details of a UI component within the component itself. This allows the component to be self-contained and independent of other components.
Composition refers to the practice of combining multiple UI components together to create more complex UIs. This is achieved by nesting components within each other, creating a tree-like structure of components.
Props, short for properties, are a way to pass data from a parent component to its child components. Props are immutable, meaning they cannot be modified by the child components. This ensures that the child components are predictable and easier to reason about.
Related Article: How to Add If Statements in a ReactJS Render Method
What is the virtual DOM in React.js?
The virtual DOM in React.js is an in-memory representation of the actual Document Object Model (DOM) of a web page. It is a lightweight copy of the DOM that React.js uses to efficiently update the UI.
When a React.js component's state or props change, React.js generates a new virtual DOM tree by calling the component's render() method. The virtual DOM tree is then compared with the previous virtual DOM tree to determine the minimal set of changes needed to update the actual DOM.
The virtual DOM also allows React.js to optimize the rendering process by performing a diffing algorithm. This algorithm efficiently compares the new virtual DOM tree with the previous one and identifies the differences between them. It then updates only the parts of the actual DOM that have changed, instead of updating the entire DOM tree.
Overall, the virtual DOM in React.js enables efficient and optimized updates to the UI, resulting in better performance and a more responsive user interface.
What is JSX in React.js?
JSX is a syntax extension for JavaScript that allows developers to write HTML-like code within JavaScript. It is used in React.js to describe the structure and appearance of the user interface.
JSX looks similar to HTML, but it is not actually HTML. It is a combination of JavaScript and XML-like syntax. JSX allows developers to write familiar HTML-like code, which makes it easier to understand and maintain the UI code.
Here is an example of JSX code:
const element = <h1>Hello, world!</h1>;
In this example, the JSX code <h1>Hello, world!</h1>
represents a React element. React elements are the building blocks of React applications and describe what should be rendered to the screen.
Under the hood, JSX code is transpiled to regular JavaScript code that can be understood and executed by the browser. This transpilation process is usually done using a tool called Babel.
JSX provides several benefits in React.js development:
1. Readability: JSX code looks similar to HTML, which makes it more readable and easier to understand, especially for developers who are familiar with HTML.
2. Expressiveness: JSX allows developers to use JavaScript expressions within the code, which makes it more expressive and useful. This allows for dynamic rendering and conditional rendering based on the state or props of a component.
3. Type Safety: JSX code can be statically analyzed by tools like TypeScript or Flow, which provide type checking and help catch errors before they happen.
Overall, JSX is a useful and expressive syntax that enhances the development experience in React.js.
What is Babel and why is it used with React.js?
Babel is a popular JavaScript compiler that is used to transform modern JavaScript code into a compatible version that can be understood and executed by older browsers. It is commonly used in React.js development to transpile JSX code into regular JavaScript code.
React.js uses JSX to describe the structure and appearance of the user interface. However, JSX is not supported by all browsers out of the box. To ensure that JSX code can be executed in all browsers, it needs to be transpiled into regular JavaScript code.
Babel provides a plugin called "babel-preset-react" that includes the necessary presets and plugins to transpile JSX code. By configuring Babel to use this plugin, developers can write JSX code in their React.js applications and have it automatically transpiled to JavaScript code that is compatible with all browsers.
In addition to transpiling JSX code, Babel can also be used to transform other modern JavaScript features, such as arrow functions, template literals, and object destructuring. This allows developers to write modern JavaScript code and have it transformed into a compatible version that can be executed in older browsers.
Babel is a useful tool that enhances the development experience in React.js by enabling the use of modern JavaScript features and ensuring compatibility with a wide range of browsers.
What is Webpack and why is it used with React.js?
Webpack is a popular module bundler for JavaScript applications. It is commonly used in React.js development to bundle and optimize the JavaScript code, CSS, and other assets of a React.js application.
React.js applications are usually composed of multiple JavaScript modules that are imported and exported. Each module can have its own dependencies and can be written in a separate file. While this modular approach promotes code reusability and maintainability, it can also result in a large number of separate JavaScript files that need to be loaded by the browser.
Webpack solves this problem by bundling all the JavaScript modules and their dependencies into a single file, called a bundle. This bundle contains all the necessary code for the React.js application to run, and it can be efficiently loaded by the browser.
In addition to bundling, Webpack also provides other features that are useful in React.js development, such as:
1. Code Splitting: Webpack allows developers to split the code into multiple bundles, which can be loaded on-demand. This can improve the initial loading time of the application and reduce the amount of code that needs to be downloaded.
2. Asset Optimization: Webpack can optimize the assets of a React.js application, such as images and CSS files, by compressing them or transforming them into a more efficient format.
3. Development Server: Webpack includes a development server that can be used to serve the React.js application during development. This server automatically rebuilds the application whenever a file is changed, and it provides features like hot module replacement, which allows for faster development and debugging.
4. Loaders: Webpack supports loaders, which are plugins that can be used to preprocess files before they are bundled. For example, there are loaders available for transpiling TypeScript code, compiling Sass or Less stylesheets, and optimizing images.
Overall, Webpack is a useful tool that enhances the development experience in React.js by bundling and optimizing the code, and providing additional features that improve productivity and performance.
Related Article: Implementing HTML Templates in ReactJS
Additional Resources
- Server-Side Rendering (SSR) with Create React App