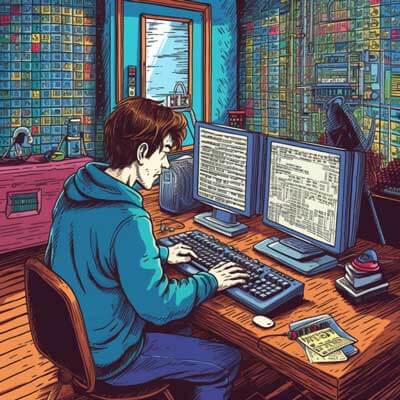
Table of Contents
To replace a substring in a string in a shell script, you can use various built-in string manipulation functions and techniques available in Linux bash. In this answer, I will provide two possible approaches to accomplish this task.
Approach 1: Using the sed Command
The sed command is a useful tool for stream editing in Linux. It can be used to search for a pattern in a file or a string and replace it with a new value. Here's how you can use sed to replace a substring in a string:
1. Make sure you have the sed command installed on your system. You can check if it's available by running the following command in your terminal:
which sed
2. Use the following syntax to replace a substring in a string using sed:
new_string=$(echo "$original_string" | sed 's/old_substring/new_substring/')
Here, original_string
is the string that contains the substring you want to replace, old_substring
is the substring you want to replace, and new_substring
is the new substring you want to replace it with.
3. Replace the variables original_string
, old_substring
, and new_substring
with the actual values in your script.
Here's an example that demonstrates the usage of sed to replace a substring in a string:
original_string="Hello, World!"new_string=$(echo "$original_string" | sed 's/World/Universe/')echo "$new_string" # Output: Hello, Universe!
This example replaces the substring "World" with "Universe" in the original string "Hello, World!".
Related Article: How to Extract Substrings in Bash
Approach 2: Using Parameter Expansion
Another way to replace a substring in a string is by using parameter expansion, a feature provided by the bash shell. Here's how you can do it:
1. Use the following syntax to replace a substring in a string using parameter expansion:
new_string=${original_string//old_substring/new_substring}
Here, original_string
is the string that contains the substring you want to replace, old_substring
is the substring you want to replace, and new_substring
is the new substring you want to replace it with.
2. Replace the variables original_string
, old_substring
, and new_substring
with the actual values in your script.
Here's an example that demonstrates the usage of parameter expansion to replace a substring in a string:
original_string="Hello, World!"new_string=${original_string//World/Universe}echo "$new_string" # Output: Hello, Universe!
This example replaces the substring "World" with "Universe" in the original string "Hello, World!".
Alternative Ideas and Best Practices
Related Article: Should You Use Numbers in Bash Script Names?
- If you only want to replace the first occurrence of the substring in the string, you can modify the sed command or parameter expansion syntax accordingly.
- The sed command supports regular expressions, so you can use more complex patterns for searching and replacing substrings.
- When using parameter expansion, be cautious about the use of the double slash (//
) to replace all occurrences of the substring. If you only want to replace the first occurrence, use a single slash (/
) instead.