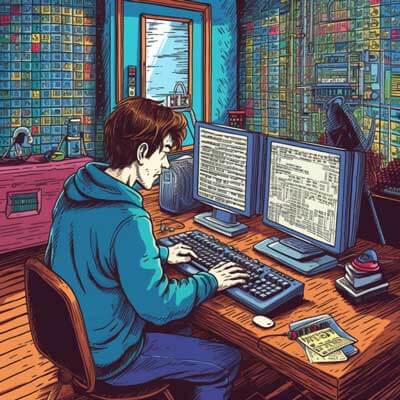
Table of Contents
To reset the index in a Pandas DataFrame, you can use the reset_index()
method. The index of a DataFrame is a unique identifier for each row, and sometimes it may be necessary to reset the index to a default integer index.
Here are two possible ways to reset the index in a Pandas DataFrame:
Method 1: Using the reset_index()
method
The reset_index()
method in Pandas allows you to reset the index of a DataFrame to a default integer index. This method returns a new DataFrame with the index reset.
Here is an example of how to use the reset_index()
method:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emma', 'Sophia', 'Michael'], 'Age': [28, 32, 25, 35], 'City': ['New York', 'Paris', 'London', 'Sydney']} df = pd.DataFrame(data) # Reset the index df_reset = df.reset_index() print(df_reset)
Output:
index Name Age City 0 0 John 28 New York 1 1 Emma 32 Paris 2 2 Sophia 25 London 3 3 Michael 35 Sydney
In the above example, the reset_index()
method resets the index of the DataFrame df
to a default integer index. The resulting DataFrame df_reset
has a new column named "index" which contains the original index values.
Related Article: How To Access Index In Python For Loops
Method 2: Using the set_index()
method followed by reset_index()
Another way to reset the index of a DataFrame is by using the set_index()
method followed by the reset_index()
method. The set_index()
method sets a column as the new index of the DataFrame, and the reset_index()
method resets the index to a default integer index.
Here is an example of how to use the set_index()
and reset_index()
methods:
import pandas as pd # Create a DataFrame data = {'Name': ['John', 'Emma', 'Sophia', 'Michael'], 'Age': [28, 32, 25, 35], 'City': ['New York', 'Paris', 'London', 'Sydney']} df = pd.DataFrame(data) # Set the 'Name' column as the index df_set_index = df.set_index('Name') # Reset the index df_reset = df_set_index.reset_index() print(df_reset)
Output:
Name Age City 0 John 28 New York 1 Emma 32 Paris 2 Sophia 25 London 3 Michael 35 Sydney
In the above example, the set_index()
method is used to set the 'Name' column as the new index of the DataFrame df
. The resulting DataFrame df_set_index
has the 'Name' column as the index. Then, the reset_index()
method is used to reset the index to a default integer index, resulting in the DataFrame df_reset
.
Alternative ideas:
- If you want to reset the index in place, without creating a new DataFrame, you can pass the parameter inplace=True
to the reset_index()
method. This will modify the existing DataFrame instead of creating a new one. Example:
df.reset_index(inplace=True)
- If you want to reset the index to a specific column, you can pass the column name to the set_index()
method. This will set the specified column as the new index. Example:
df.set_index('Column_Name', inplace=True)
- If you want to reset the index to a range of values starting from 1, you can use the range()
function in combination with the len()
function. Example:
df.index = range(1, len(df)+1)
Best practices:
- It is a good practice to reset the index of a DataFrame after performing operations that may have modified the index or when the current index is no longer meaningful or useful.
- When resetting the index, it is important to consider whether you want to create a new DataFrame with the reset index or modify the existing DataFrame in place. The reset_index()
method provides the flexibility to choose between these options.
- If you want to reset the index to a specific column, make sure that the column contains unique values. Otherwise, you may end up with duplicate index values, which can cause unexpected behavior in subsequent operations.
- After resetting the index, it is recommended to check the resulting DataFrame to ensure that the index has been reset correctly and meets your expectations.