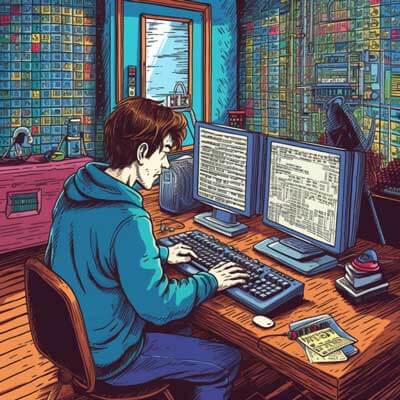
Table of Contents
To retrieve the current date and time in Java, you can use the built-in classes provided by the Java API. There are several ways to achieve this, and in this answer, I will explain two common approaches.
Approach 1: Using the java.util.Date Class
The java.util.Date class provides methods to get the current date and time. Here's an example of how you can use this class:
import java.util.Date; public class GetCurrentDateTime { public static void main(String[] args) { // Create a new Date object Date currentDate = new Date(); // Print the current date and time System.out.println("Current Date and Time: " + currentDate); } }
In this example, we import the java.util.Date
class and create a new Date
object called currentDate
. The Date
object is initialized with the current date and time. Finally, we print the current date and time using the System.out.println
method.
Related Article: How to Read a JSON File in Java Using the Simple JSON Lib
Approach 2: Using the java.time.LocalDateTime Class
Starting from Java 8, the java.time
package was introduced, which provides more comprehensive support for date and time manipulation. One of the classes in this package is LocalDateTime
, which represents a date and time without a time zone. Here's an example of how you can use this class to retrieve the current date and time:
import java.time.LocalDateTime; public class GetCurrentDateTime { public static void main(String[] args) { // Create a new LocalDateTime object LocalDateTime currentDateTime = LocalDateTime.now(); // Print the current date and time System.out.println("Current Date and Time: " + currentDateTime); } }
In this example, we import the java.time.LocalDateTime
class and create a new LocalDateTime
object called currentDateTime
. The LocalDateTime.now()
method is used to get the current date and time. Finally, we print the current date and time using the System.out.println
method.
Best Practices:
- It is recommended to use the java.time
package introduced in Java 8, as it provides a more modern and comprehensive set of classes for date and time manipulation.
- When working with dates and times, consider the time zone and daylight saving time issues. The java.time
package provides classes such as ZonedDateTime
to handle time zone-specific date and time operations.
- Always use the appropriate date and time class based on your requirement. For example, if you only need to work with dates, consider using the java.time.LocalDate
class.
Alternative Approach:
Apart from the approaches mentioned above, you can also use third-party libraries such as Joda-Time or Apache Commons Lang to retrieve the current date and time in Java. These libraries provide additional features and flexibility for date and time manipulation.
Here's an example of how you can use Joda-Time to retrieve the current date and time:
import org.joda.time.DateTime; public class GetCurrentDateTime { public static void main(String[] args) { // Create a new DateTime object DateTime currentDateTime = new DateTime(); // Print the current date and time System.out.println("Current Date and Time: " + currentDateTime); } }
In this example, we import the org.joda.time.DateTime
class from the Joda-Time library and create a new DateTime
object called currentDateTime
. The DateTime
object is initialized with the current date and time. Finally, we print the current date and time using the System.out.println
method.