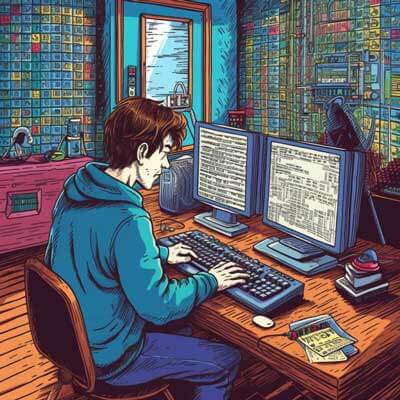
To reverse a string in Java, you can use various approaches. Here are two possible solutions:
1. Using a StringBuilder
One straightforward way to reverse a string in Java is by using the StringBuilder
class, which provides a reverse()
method. Here’s an example:
public String reverseString(String input) { StringBuilder sb = new StringBuilder(input); sb.reverse(); return sb.toString(); }
In this example, we create a new StringBuilder
object with the given input string. Then, we call the reverse()
method, which modifies the string in-place, reversing its characters. Finally, we convert the reversed StringBuilder
object back to a string using the toString()
method and return it.
This approach has a time complexity of O(n), where n is the length of the input string.
Related Article: How To Parse JSON In Java
2. Using a char array
Another approach to reverse a string in Java is by converting the string to a char array and then swapping the characters from both ends of the array. Here’s an example:
public String reverseString(String input) { char[] charArray = input.toCharArray(); int left = 0; int right = charArray.length - 1; while (left < right) { char temp = charArray[left]; charArray[left] = charArray[right]; charArray[right] = temp; left++; right--; } return new String(charArray); }
In this example, we first convert the input string to a char array using the toCharArray()
method. Then, we initialize two pointers: left
pointing to the first character of the array and right
pointing to the last character. We swap the characters at left
and right
positions iteratively while incrementing left
and decrementing right
until they cross each other.
Once we finish swapping all the characters, we create a new string from the reversed char array using the String
constructor and return it.
This approach also has a time complexity of O(n), where n is the length of the input string.
Alternate Approaches
– Using recursion: You can also reverse a string recursively by recursively calling a method to reverse the substring excluding the first character and then appending the first character at the end. However, this approach may not be as efficient as the previous ones for large strings due to the additional overhead of recursive function calls.
– Using Collections.reverse()
: If you are allowed to use the java.util.Collections
class, you can convert the string to a List
, reverse the list using the reverse()
method, and then convert it back to a string.
It’s important to note that strings in Java are immutable, meaning that you cannot modify them directly. Therefore, converting the string to a mutable data structure like StringBuilder
or char[]
is necessary to perform the reversal.
Overall, reversing a string in Java can be achieved using various approaches, and the choice depends on factors such as performance requirements, code readability, and personal preference.
Related Article: How To Convert Array To List In Java