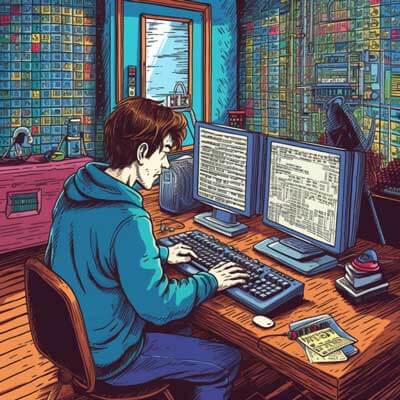
Table of Contents
To reverse an array in Javascript without using any libraries, you can follow these steps:
Method 1: Using a Loop
One way to reverse an array is by using a loop. Here's how you can do it:
function reverseArray(arr) { var reversedArray = []; for (var i = arr.length - 1; i >= 0; i--) { reversedArray.push(arr[i]); } return reversedArray; } var originalArray = [1, 2, 3, 4, 5]; var reversedArray = reverseArray(originalArray); console.log(reversedArray);
In this method, we initialize an empty array called reversedArray
. Then, using a loop, we iterate over the original array from the last element to the first element. Inside the loop, we push each element into the reversedArray
. Finally, we return the reversedArray
.
Related Article: JavaScript Objects & Managing Complex Data Structures
Method 2: Using the Array.prototype.reverse() Method
Another way to reverse an array is by using the built-in reverse()
method provided by the Array
object in JavaScript. Here's an example:
var originalArray = [1, 2, 3, 4, 5]; var reversedArray = originalArray.reverse(); console.log(reversedArray);
In this method, we directly call the reverse()
method on the original array. This method reverses the elements in place, meaning it modifies the original array itself. Therefore, in this example, the originalArray
is also reversed.
Best Practices
When reversing an array without libraries in JavaScript, there are a few best practices to keep in mind:
1. Be mindful of the original array: Depending on your use case, you may want to preserve the original array or reverse it in place. Choose the appropriate method accordingly.
2. Consider the array's mutability: If you need to preserve the original array and avoid mutation, use the loop method to create a new reversed array. If mutability is not a concern, using the reverse()
method can be more concise.
3. Performance considerations: For small arrays, the performance difference between the two methods is negligible. However, for large arrays, the loop method can be more efficient as it avoids modifying the original array in place.
Alternative Ideas
While the two methods above are the most common ways to reverse an array in JavaScript, there are alternative approaches you can explore:
1. Using the spread operator: You can leverage the spread operator (...
) to reverse an array. Here's an example:
var originalArray = [1, 2, 3, 4, 5]; var reversedArray = [...originalArray].reverse(); console.log(reversedArray);
In this approach, the spread operator creates a new array with the same elements as the original array. Then, the reverse()
method is applied to the new array, reversing the order of its elements.
2. Using Array destructuring: Another alternative is to use array destructuring to reverse an array. Here's an example:
var originalArray = [1, 2, 3, 4, 5]; var reversedArray = [...originalArray].reverse(); console.log(reversedArray);
In this approach, the array destructuring syntax ([...originalArray]
) creates a new array with the same elements as the original array. Then, the reverse()
method is applied to the new array, reversing the order of its elements.
These alternative ideas provide different ways to achieve the same result, allowing you to choose the approach that best fits your coding style and requirements.