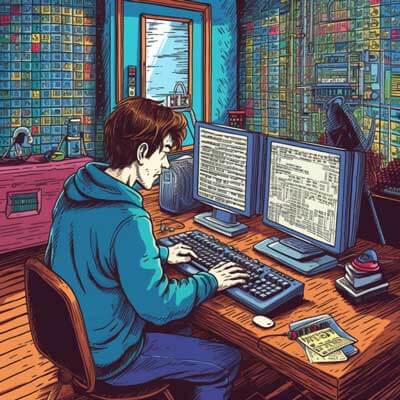
Table of Contents
To round up a number in Python, you can make use of the math module's ceil() function, the decimal module, or the built-in round() function in combination with some basic arithmetic. In this answer, we will explore these different approaches and provide examples of how to round up a number in Python.
Using the math.ceil() function
One way to round up a number in Python is by using the math.ceil() function. This function returns the smallest integer greater than or equal to a given number. Here's how you can use it:
import math number = 4.3 rounded_up = math.ceil(number) print(rounded_up) # Output: 5
In this example, we import the math module and use the ceil() function to round up the number 4.3 to the nearest integer, which is 5.
Related Article: How to Remove a Virtualenv in Python
Using the decimal module
Python's decimal module provides a Decimal class that allows for precise decimal arithmetic. This module also provides a method called quantize(), which can be used to round up a number. Here's an example:
from decimal import Decimal, ROUND_UP number = Decimal('4.3') rounded_up = number.quantize(Decimal('1.'), rounding=ROUND_UP) print(rounded_up) # Output: 5
In this example, we import the Decimal class and the ROUND_UP constant from the decimal module. We then create a Decimal object with the number 4.3 and use the quantize() method to round it up to the nearest integer. The rounding parameter is set to ROUND_UP to ensure that the number is always rounded up.
Using the round() function and basic arithmetic
Another way to round up a number in Python is by using the built-in round() function in combination with basic arithmetic. Here's an example:
number = 4.3 rounded_up = -int(-number // 1) print(rounded_up) # Output: 5
In this example, we use the integer division operator (//) to divide the number by 1. This effectively truncates the decimal part of the number. We then use the negation operator (-) twice to round up the number. By negating the result of the division and then negating it again, we effectively round up the number to the nearest integer.
Alternative approaches
While the above methods are commonly used to round up a number in Python, there are alternative approaches that you can explore:
- Using the math.ceil() function in combination with the int() function:
import math number = 4.3 rounded_up = int(math.ceil(number)) print(rounded_up) # Output: 5
- Using the numpy module:
import numpy as np number = 4.3 rounded_up = np.ceil(number) print(int(rounded_up)) # Output: 5
- Using the NumPy ceil() function in combination with the int() function.
Related Article: How to Find a Value in a Python List
Best practices
When rounding up a number in Python, consider the following best practices:
- Choose the method that best suits your specific use case. If you need precise decimal arithmetic, consider using the decimal module. If you are working with simple floating-point numbers, the math.ceil() function or the round() function with basic arithmetic may be sufficient.
- Be aware of the potential floating-point precision issues that can arise when working with floating-point numbers. These issues can affect the accuracy of the rounded result.
- When using the decimal module, ensure that you use the appropriate rounding mode, such as ROUND_UP, to achieve the desired rounding behavior.
- Consider the performance implications of the different rounding methods. In general, the math.ceil() function and the round() function with basic arithmetic tend to be faster than the decimal module for simple rounding operations.