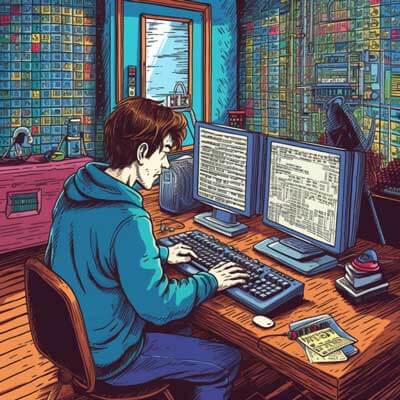
Table of Contents
Running Tests on Specific Files
Testing is a crucial part of software development. It ensures that your code behaves as expected and helps identify bugs before they reach production. Often, you may want to run tests on a specific file rather than executing the entire test suite. This can save time and focus your debugging efforts on particular areas of your application.
To run tests on a specific file using npm, you typically need to leverage a test runner like Jest or Mocha. These tools allow you to specify which files to test, making it easy to isolate issues without running unnecessary tests.
Related Article: How to Fix npm Self Signed Certificate Error
Setting Up Test Scripts
In order to run tests using npm, you first need to set up your test scripts in the package.json
file. This file contains metadata about your project, including scripts that can be executed with npm.
Here is an example of how to set up a test script using Jest:
{ "scripts": { "test": "jest" }}
For Mocha, it might look like this:
{ "scripts": { "test": "mocha" }}
Once you have your test script set up, you can run it by executing npm test
from your terminal.
Using npm Test Command
The npm test command executes the script that you defined in your package.json
file. When you type npm test
in your terminal, npm looks up this script and runs it. If your script is set to run Jest or Mocha, this command will start the testing process.
For example:
npm test
This command will run all the tests defined in your project unless you specify otherwise.
Specifying Test File Paths
To run tests on a specific file, you can specify the file path directly in the test command. With Jest, you can do this by adding the path of the test file as an argument.
For example:
npm test -- path/to/your/testFile.test.js
In this command, --
indicates that the following arguments are meant for the underlying test framework (Jest). For Mocha, the syntax is similar:
npm test -- path/to/your/testFile.spec.js
Related Article: How to Use npm with Next.js
Running Unit Tests
Unit tests are designed to test individual components or functions in isolation. They ensure that each part of your code performs as intended. When you want to run unit tests on a specific file, you can follow the same approach mentioned above, specifying the path to the unit test file.
For instance, if you have a unit test file named math.test.js
, you can run it using:
npm test -- math.test.js
This command will execute just the tests defined in the math.test.js
file, allowing you to quickly check its functionality without running the entire test suite.
Creating a Test Suite
A test suite is a collection of tests that are grouped together. When working with Jest or Mocha, you typically create test suites using describe
blocks. This enables you to organize your tests logically.
Here’s an example of a test suite in Jest:
// math.test.jsdescribe('Math Functions', () => { test('adds 1 + 2 to equal 3', () => { expect(1 + 2).toBe(3); });});
For Mocha, the syntax is similar:
// math.spec.jsconst assert = require('assert');describe('Math Functions', function() { it('adds 1 + 2 to equal 3', function() { assert.strictEqual(1 + 2, 3); });});
Creating test suites helps in organizing tests and making them easier to maintain.
Defining Test Cases
Test cases are the individual tests that check specific functionality within your code. In Jest, you define a test case using the test
function, while in Mocha, you use the it
function. Each test case should focus on a single aspect of function behavior.
For example, in Jest:
// string.test.jsdescribe('String Functions', () => { test('should return the length of a string', () => { expect('hello'.length).toBe(5); });});
In Mocha, the structure is similar:
// string.spec.jsconst assert = require('assert');describe('String Functions', function() { it('should return the length of a string', function() { assert.strictEqual('hello'.length, 5); });});
Defining clear and concise test cases is vital for maintaining code quality and ensuring that each part behaves correctly.
Executing Tests with Jest
Jest is a popular testing framework for JavaScript applications, especially those built with React. To execute tests using Jest, you can run the following command:
npm test
To execute a specific test file, you can specify the file path as mentioned earlier:
npm test -- path/to/testFile.test.js
This will run all the tests within that specific file. Jest provides additional options for running tests, such as --watch
, which enables continuous testing as you make changes to your code.
Related Article: How to Fix npm Warn Ebadengine Unsupported Engine
Testing with Mocha
Mocha is another widely used testing framework that provides flexibility in structuring tests. To run tests in Mocha, the command is similar:
npm test
For a specific test file, you would use:
npm test -- path/to/testFile.spec.js
Mocha's output is typically more verbose, making it easier to see which tests passed or failed. Using the --reporter
option, you can customize the output format.
Passing Arguments to npm Test
When running tests, you might need to pass additional arguments to the underlying test runner. This can be done by appending them after the --
in the command.
For example, if you want to run Jest with coverage reporting, you can use:
npm test -- --coverage
For Mocha, if you want to specify a reporter, you can do something like this:
npm test -- --reporter spec
Passing arguments allows for more control over how tests are executed and reported.
Configuring npm for Specific Files
Configuring npm to run specific test files can streamline your workflow. By adding custom scripts to your package.json
, you can create shortcuts for frequently run tests.
For instance, you can add a script for a specific test file:
{ "scripts": { "test:math": "jest path/to/math.test.js" }}
You can then run this specific test with:
npm run test:math
This method reduces the need to remember long command-line arguments and improves efficiency.
Using npm Test Options
npm test options allow for fine-tuning the testing process. Aside from specifying test files, you can control the behavior of your test runner. For example, Jest provides options like --watch
, --coverage
, and more, while Mocha offers reporters, timeout settings, and other configurations.
To run tests with coverage reporting in Jest, you would execute:
npm test -- --coverage
This command would provide a coverage report along with the test results, helping identify untested parts of your code.
Related Article: How to Use npm for Package Management
Targeting a Single Test File
Targeting a single test file is a common practice when debugging or refining specific features. This approach saves time and focuses your testing efforts on the relevant parts of your application.
To target a single test file, you can use:
npm test -- path/to/your/testFile.test.js
This command runs only the tests contained in that file, allowing you to quickly verify functionality without running every test in your suite.
Syntax for npm Test with Specific Files
The syntax for running npm tests on specific files is consistent across different testing frameworks. The general format is:
npm test -- path/to/your/testFile.extension
Replace extension
with .test.js
for Jest or .spec.js
for Mocha. This syntax allows for flexibility and precision when executing tests, making it easier to manage large projects with numerous test files.
Running tests on specific files is a vital practice for maintaining code quality. By leveraging npm and popular testing frameworks like Jest and Mocha, developers can streamline their testing workflows and ensure that their applications function as expected.