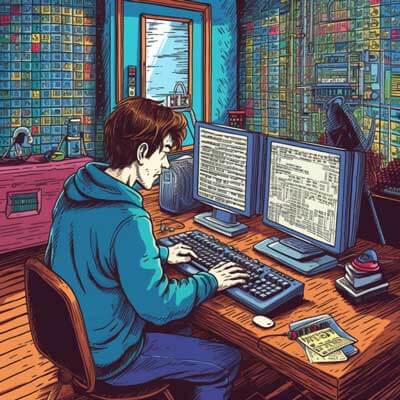
Table of Contents
Overview of Testing with Npm
Testing is an essential part of software development. It ensures that your code behaves as expected and catches errors before they reach production. Npm, the package manager for Node.js, plays a crucial role in managing testing tools and libraries. By integrating testing directly into your workflow, you can maintain high code quality. Npm allows you to install various testing frameworks and libraries that can help you create, run, and manage your tests conveniently.
Related Article: How to Use tough-cookie with npm
Setting Up Testing Frameworks
To run tests in your project, you first need to set up a testing framework. Popular choices include Jest, Mocha, and Jasmine. Each of these frameworks has its own strengths, so the choice depends on your project's requirements.
To install Jest, for example, you can run the following command:
npm install --save-dev jest
For Mocha and Chai, you would use:
npm install --save-dev mocha chai
After installation, configuring the framework is often necessary. For Jest, you may add a configuration section in your package.json
:
{ "scripts": { "test": "jest" }}
Configuring Mocha might involve creating a test directory and specifying a test runner in your package.json:
{ "scripts": { "test": "mocha 'test/**/*.js'" }}
The choice of framework will dictate how you structure your tests and run them, leading to the next topic: creating a test script.
Creating a Test Script
A test script is a small piece of code that validates functionality in your application. This script includes the actual tests written in JavaScript. To create one, you can add a new file in your test directory, such as example.test.js
if you are using Jest, or example.spec.js
for Mocha.
An example of a simple test script using Jest looks like this:
// example.test.jstest('adds 1 + 2 to equal 3', () => { expect(1 + 2).toBe(3);});
For Mocha, a simple test might look like this:
// example.spec.jsconst assert = require('chai').assert;describe('Simple Math Test', function() { it('should return 3 when adding 1 and 2', function() { assert.equal(1 + 2, 3); });});
Creating these scripts allows you to define expected outcomes and validate your code against them. The next step involves running these tests from the command line.
Running Tests from the Command Line
Running tests via the command line is a common practice. It enables you to automate the testing process and integrate it into your development workflow. After you have set up your test script, you can execute your tests using npm.
For Jest, the command would be:
npm test
For Mocha, if you have set it up in your package.json
, the command remains the same:
npm test
This command triggers the test runner, which will look for the defined test scripts and execute them. If any tests fail, the output will include error messages to help you identify issues in your code.
Related Article: How to Track the History of npm Packages
Specifying a Test File Path
When working on a large codebase, you might only want to run tests for a specific file rather than all tests. Npm allows you to specify the path to a test file directly from the command line.
With Jest, you can run a specific test file like this:
npx jest path/to/your/test/example.test.js
For Mocha, you can specify the test file like so:
npx mocha path/to/your/test/example.spec.js
This functionality helps save time during development by allowing you to focus on specific components or features without running the entire test suite.
Using Jest for File-Specific Tests
Jest is particularly suited for running file-specific tests due to its simplicity and robust features. The framework allows for running tests directly from the command line with a designated path. Jest also supports watch mode, which facilitates real-time testing as code changes occur.
To run Jest in watch mode, use:
npx jest --watch
This command keeps Jest running and monitors file changes, automatically rerunning tests for affected files. This is useful for developers focusing on a specific file, as it keeps the workflow smooth and continuous.
Using Mocha and Chai Together
Mocha is a flexible testing framework that pairs well with the Chai assertion library. Chai provides a wide range of assertions to validate your code's behavior. When running a specific test file with Mocha, you can leverage Chai's syntax to write clear and readable tests.
To run Mocha with Chai, install both libraries, and then create a test file, for example, math.spec.js
:
// math.spec.jsconst { expect } = require('chai');describe('Math Functions', function() { it('should return 4 when multiplying 2 and 2', function() { expect(2 * 2).to.equal(4); });});
Executing the tests in this file is as simple as running:
npx mocha path/to/your/test/math.spec.js
This approach allows you to maintain a clear structure for your tests while ensuring each component is tested thoroughly.
Configuring Npm for Targeted Testing
Configuring npm to facilitate targeted testing is beneficial for larger projects. You can define multiple test scripts in your package.json
file, allowing for quick execution of tests based on the context.
For instance, you could have different scripts for unit tests, integration tests, and end-to-end tests:
{ "scripts": { "test": "jest", "test:unit": "jest path/to/unit/tests", "test:integration": "jest path/to/integration/tests" }}
Related Article: How to Handle npm Warn Using –force Protection Disabled
Running Tests in a Specific Directory
When managing a larger codebase, organizing tests into directories can streamline the testing process. You can run all tests within a specific directory by specifying the directory path when executing your test runner.
For Jest, you can run all tests in a directory like this:
npx jest path/to/your/tests/
For Mocha, the command would look similar:
npx mocha path/to/your/tests/
Best Practices for Organizing Test Files
Organizing test files properly is crucial for maintaining a scalable and manageable codebase. Here are some best practices to consider:
- Directory Structure: Keep your tests in a separate directory, often named tests
or spec
, at the root of your project. This helps in distinguishing between production code and test code.
- File Naming Conventions: Use consistent naming conventions for test files, such as filename.test.js
for Jest or filename.spec.js
for Mocha. This makes it easier to identify test files at a glance.
- Grouping Tests: Use descriptive describe
blocks in your test files to group related tests together. This enhances readability and makes it easier to understand what is being tested.
- Keep Tests Small: Each test should verify a small piece of functionality. This makes it easier to isolate failures and understand what part of the code is broken.