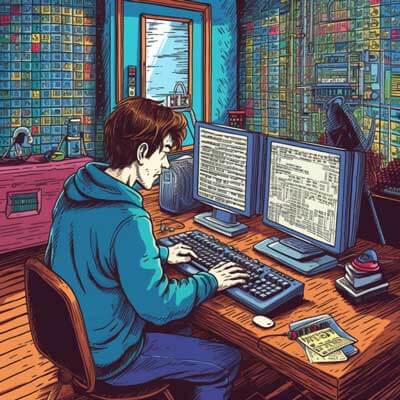
Table of Contents
Overview of Library Target in Configuration
Webpack is a popular module bundler that allows developers to bundle JavaScript files for usage in a browser. One of the key features of Webpack is its ability to configure how libraries are output. This is controlled through the libraryTarget
option in the configuration file. The libraryTarget
defines how the library will be exposed and used in different environments.
Related Article: How To Exclude Test Files In Webpack Builds
What is libraryTarget in configuration
The libraryTarget
setting in Webpack defines the format in which a library will be exported. This can affect how the library can be imported and used in different environments, such as CommonJS, AMD, or as a global variable. Each format has its own use cases and benefits, allowing developers to choose the best one for their needs.
How to configure libraryTarget in configuration
Configuring the libraryTarget
in Webpack is done in the main configuration file, typically named webpack.config.js
. You would specify the library
and libraryTarget
options under the output
property. Here is an example of how to do this:
// webpack.config.js module.exports = { output: { filename: 'my-library.js', path: __dirname + '/dist', library: 'MyLibrary', libraryTarget: 'umd' // Set the desired library target here } };
This example defines a library named MyLibrary
and specifies that it should be output using the UMD (Universal Module Definition) format.
Different libraryTarget options available
Webpack offers several options for the libraryTarget
, each suited for different environments:
- var: Exposes the library as a variable. Useful for simple scripts.
- module: Exposes the library as an ES module.
- this: Exposes the library to the global this
context.
- commonjs: Exposes the library as a CommonJS module for Node.js.
- amd: Exposes the library as an AMD module for RequireJS.
- umd: A universal format that works as both AMD and CommonJS, and also exposes a global variable.
Choosing the right option depends on the target environment where the library will be used.
Related Article: How to Configure Webpack for Expo Projects
Default libraryTarget in configuration
The default libraryTarget
in Webpack is var
. This means if no libraryTarget
is specified, Webpack will output the library as a variable in the global scope. This is sufficient for many use cases, especially when creating simple scripts that do not require module support.
Creating a library with configuration settings
To create a library, you need to define the output settings in your Webpack configuration. Here’s an example of a library that could be used in both Node.js and browser environments:
// webpack.config.js module.exports = { entry: './src/index.js', output: { filename: 'my-library.js', path: __dirname + '/dist', library: 'MyLibrary', libraryTarget: 'umd' } };
This configuration compiles the code in src/index.js
and outputs it as my-library.js
in the dist
directory, making it available as MyLibrary
in various module systems.
Comparison of umd and commonjs library targets
The umd
(Universal Module Definition) target is designed to work in multiple environments, while the commonjs
target is specific to Node.js.
With umd
, the library can be imported in both Node.js and the browser. It checks for CommonJS, AMD, or a global variable in that order. Here’s a simple comparison:
- CommonJS:
// Using in Node.js const myLibrary = require('my-library');
- UMD:
// Using in Node.js or AMD define(['my-library'], function(myLibrary) { // Use myLibrary });
The umd
format is more flexible and is commonly used for libraries intended for broader use.
Impact of libraryTarget on output
The libraryTarget
directly affects how the output library can be used in various environments. Depending on the selected target, the structure and accessibility of the library will change.
For example, when using var
, the library will be accessible globally:
// After building with libraryTarget: 'var' console.log(MyLibrary); // Accessed globally
In contrast, with commonjs
, you will need to import the library using require
, affecting how other modules can access it and how they integrate with build systems.
Related Article: How to Use Environment Variables in Webpack
Specifying multiple libraryTargets in configuration
Webpack does not allow specifying multiple libraryTarget
options directly within a single configuration. However, you can create multiple configurations in your webpack.config.js
to achieve this. Here’s an example:
// webpack.config.js module.exports = [ { entry: './src/index.js', output: { filename: 'my-library-commonjs.js', path: __dirname + '/dist', library: 'MyLibrary', libraryTarget: 'commonjs' } }, { entry: './src/index.js', output: { filename: 'my-library-umd.js', path: __dirname + '/dist', library: 'MyLibrary', libraryTarget: 'umd' } } ];
This creates two outputs: one for CommonJS and one for UMD, allowing users to choose the appropriate version based on their needs.
Effects of libraryTarget on module compatibility
The selected libraryTarget
influences how compatible the library is with other module systems. For example, using commonjs
makes the library suitable for Node.js environments but may limit browser compatibility unless combined with a bundler.
On the other hand, using umd
or amd
provides broader compatibility, allowing the library to be easily integrated into various projects regardless of the module system used.
Best practices for using libraryTarget in configuration
When configuring libraryTarget
, consider the following best practices:
1. Know Your Audience: Understand where your library will be used. If it’s for both Node.js and the browser, favor umd
.
2. Keep It Simple: If you’re only targeting one environment, choose the most suitable libraryTarget
for that environment.
3. Documentation: Clearly document the output formats available and how to use them.
4. Testing: Test the library in different environments to ensure compatibility.
Following these practices will help ensure that your library is usable and effective for its intended audience.
Using externals with libraryTarget settings
When creating a library, you might want to exclude certain dependencies from the bundle, allowing users to provide them externally. This is done using the externals
configuration in Webpack. Here’s how to set this up:
// webpack.config.js module.exports = { entry: './src/index.js', output: { filename: 'my-library.js', path: __dirname + '/dist', library: 'MyLibrary', libraryTarget: 'umd' }, externals: { react: 'React', // Exclude React from the bundle lodash: '_' } };
In this configuration, React and Lodash are treated as external libraries, meaning they must be included by the user when using your library, reducing bundle size and potential version conflicts.
Related Article: How to Choose Between Gulp and Webpack
Implementing hot module replacement with libraryTarget
Hot Module Replacement (HMR) allows modules to be updated in a running application without a full refresh. To use HMR with a library, ensure you set up the HMR plugin and configuration correctly. Here’s a basic setup:
// webpack.config.js const webpack = require('webpack'); module.exports = { entry: './src/index.js', output: { filename: 'my-library.js', path: __dirname + '/dist', library: 'MyLibrary', libraryTarget: 'umd' }, plugins: [ new webpack.HotModuleReplacementPlugin() ], devServer: { hot: true, contentBase: './dist' } };
In this configuration, the library is set up for HMR, allowing developers to see changes in real-time without refreshing the entire application, speeding up the development process.
Async loading with libraryTarget configuration
Async loading of libraries can be achieved using dynamic imports or the import()
syntax. Depending on the libraryTarget
, you can optimize how libraries are loaded. Here’s an example:
// src/index.js export const myFunction = () => { console.log('Hello from my library!'); }; // Async import in another file import('path/to/my-library') .then(module => { module.myFunction(); });
This method allows the library to be loaded only when necessary, improving performance in larger applications by reducing the initial load time.
Additional Resources
- Understanding libraryTarget in Webpack