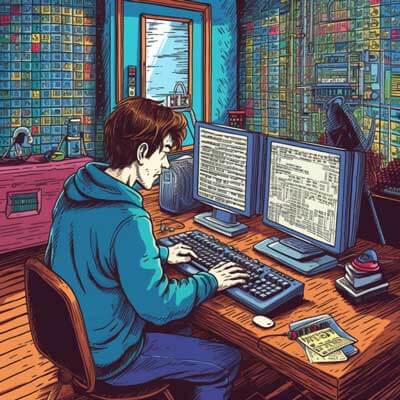
Table of Contents
To set the selected option of a select element in jQuery, you can use the val()
method combined with the :selected
selector. Here are two possible ways to achieve this:
Method 1: Using Attribute Selector
// Assuming you have a select element with id "mySelect" $("#mySelect option[value='optionValue']").attr("selected", "selected");
This method uses the attribute selector to target the option element with the desired value. The attr()
method is then used to set the selected
attribute to "selected", which makes the option selected.
Related Article: How to Create a Two-Dimensional Array in JavaScript
Method 2: Using val() Method
// Assuming you have a select element with id "mySelect" $("#mySelect").val("optionValue");
This method uses the val()
method to set the value of the select element directly. When the value matches one of the available options, that option will automatically be selected.
It is worth mentioning that if you have multiple select elements with the same value, both methods will select the first matching option. If you want to select multiple options, you can pass an array of values to the val()
method.
Best Practices and Suggestions
Related Article: Optimal Practices for Every JavaScript Component
- It is recommended to use the prop()
method instead of attr()
to set the selected option. The prop()
method sets or returns properties and is generally preferred over attr()
when dealing with boolean attributes like selected
. Here is an example:
$("#mySelect option[value='optionValue']").prop("selected", true);
- If you are working with a dynamic select element that gets populated after the page loads, make sure to run your code after the element is populated. You can use the ready()
method or place your code at the end of the document to ensure the DOM is ready before manipulating the select element.
- If you are setting the selected option based on user input or data from an API, make sure to validate and sanitize the input to prevent any potential security vulnerabilities. Cross-site scripting (XSS) attacks can occur if user input is not properly sanitized.
- If you want to clear the selected option, you can either set the value to an empty string or use the prop()
method to remove the selected
attribute:
$("#mySelect").val(""); // Clear the selected option by setting the value to an empty string $("#mySelect option").prop("selected", false); // Clear the selected option using prop() method
These suggestions and best practices will help you set the selected option by value in jQuery in a safe and efficient manner.