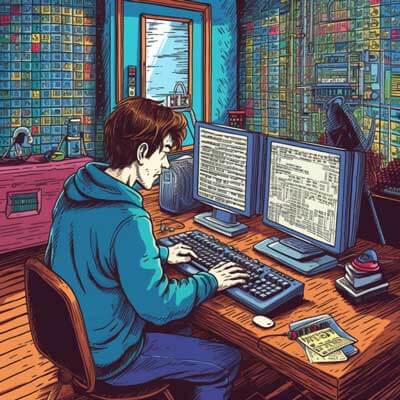
Table of Contents
Overview
Setting up a proxy in a development environment allows developers to route API requests from the frontend to a backend server. This is useful when the frontend and backend are running on different ports or when there are CORS (Cross-Origin Resource Sharing) issues. By using a proxy, requests to the backend can be seamlessly forwarded without needing to change the code in the frontend application.
Related Article: How to Use webpack -d for Development Mode
Configuring devServer
To enable proxy functionality in Webpack, modifications need to be made to the webpack.config.js
file. The devServer
property is where the proxy settings are configured. Below is an example configuration:
// webpack.config.js module.exports = { // other configuration settings... devServer: { port: 3000, proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, }, }, };
In this setup, any request starting with /api
will be proxied to http://localhost:5000
.
Proxy Configuration Options Explained
The proxy configuration can include several options:
- target
: Specifies the URL to proxy the requests to.
- changeOrigin
: Changes the origin of the host header to the target URL.
- pathRewrite
: Allows rewriting of the URL path before sending it to the target.
- secure
: Controls whether the proxy should verify the SSL certificate.
Each option modifies how the proxy behaves and can be tailored to specific needs.
Handling CORS Issues
CORS issues arise when a web application attempts to access resources from a domain other than its own. By setting up a proxy, these issues can be circumvented since the browser sees the requests as coming from the same origin. The proxy forwards the requests to the backend server, making it appear to the browser that the resources are being fetched from the same domain.
Related Article: How to Set Up Webpack SCSS Loader
Making API Requests Through a Proxy
When an API request is made from the frontend, it should match the proxy configuration. For example, if the backend API endpoint is http://localhost:5000/api/users
, the frontend can simply call /api/users
:
// Example API request using fetch fetch('/api/users') .then(response => response.json()) .then(data => console.log(data));
This request will be routed through the proxy to the backend server.
Using Target in Proxy Configuration
The target
option in the proxy configuration defines where the requests will be sent. This is crucial when the backend server is hosted on a different port or domain. For instance, if the backend API is hosted on a cloud service, the target could be set like this:
// webpack.config.js proxy: { '/api': { target: 'https://api.example.com', changeOrigin: true, }, },
This configuration ensures that requests to /api
are forwarded to https://api.example.com
.
Rewriting URLs for Backend Server Calls
Sometimes, the API endpoints on the backend may not match the routes expected by the frontend. The pathRewrite
option allows for modifying the outgoing request URL. For example:
// webpack.config.js proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, pathRewrite: { '^/api': '', // Removes '/api' from the forwarded request }, }, },
In this case, a request to /api/users
will be forwarded to http://localhost:5000/users
.
Setting Up Hot Module Replacement with Proxy
Hot Module Replacement (HMR) allows updates to be applied to the application without a full reload. When using a proxy, HMR can be enabled in the devServer
settings:
// webpack.config.js devServer: { hot: true, proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, }, },
With HMR enabled, changes in the application will be reflected instantly, improving the development experience.
Related Article: How to Choose Between Gulp and Webpack
Implementing a Middleware
In some cases, additional processing may be required for requests passing through the proxy. Middleware can be used to manipulate requests or responses. Here is an example of integrating middleware:
const { createProxyMiddleware } = require('http-proxy-middleware'); // In your webpack config devServer: { before: function(app) { app.use('/api', createProxyMiddleware({ target: 'http://localhost:5000', changeOrigin: true, onProxyReq: (proxyReq, req, res) => { // Custom logic can be added here }, })); }, },
This setup allows for custom processing of API requests before they reach the backend.
Accessing Localhost Through Proxy
In development, accessing a local backend server through a proxy is common. By setting the target to http://localhost:[port]
, the frontend can communicate with the local server without CORS issues:
// webpack.config.js proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, },
Requests made to /api
will be directed to the local server, allowing for testing and development.
Setting Up a Proxy for Multiple Servers
To manage requests to multiple backend servers, the proxy configuration can be expanded. Here is an example of how to set up different paths for different servers:
// webpack.config.js proxy: { '/api': { target: 'http://localhost:5000', changeOrigin: true, }, '/auth': { target: 'http://localhost:6000', changeOrigin: true, }, },
In this scenario, requests to /api
will be sent to one server, while requests to /auth
will go to another.
Troubleshooting Proxy Issues in Development
Common issues when using proxies include:
- Incorrect target URL: Ensure the target is correctly set and the backend server is running.
- CORS errors: Check if the proxy settings match the request paths.
- Network issues: Verify that there are no firewall or network settings blocking the connections.
Using browser developer tools can help diagnose these issues by observing network requests.
Related Article: How to Fix Angular Webpack Plugin Initialization Error
Enabling WebSocket Connections
WebSockets require additional configuration for proxies. The proxy setup must handle WebSocket connections explicitly. Here’s how to enable WebSocket support:
// webpack.config.js proxy: { '/api': { target: 'http://localhost:5000', ws: true, // Enable WebSocket proxying changeOrigin: true, }, },
The ws
option allows WebSocket connections to be proxied, ensuring real-time communication works without issues.
Improving Development Workflow
Using a proxy can greatly enhance the development workflow. It allows for easier testing of API calls without changing the frontend code. This setup can lead to faster iterations and smoother integration between the frontend and backend.
Identifying Limitations
While proxies are useful, they come with limitations. For instance, they may not fully replicate a production environment, leading to discrepancies in behavior. Additionally, performance can be impacted due to the added layer of routing requests.
Using Environment Variables
To make the proxy configuration more flexible, environment variables can be used. This allows developers to switch settings based on the environment (development, testing, production). Here’s an example:
// webpack.config.js const targetUrl = process.env.API_URL || 'http://localhost:5000'; proxy: { '/api': { target: targetUrl, changeOrigin: true, }, },
Related Article: How To Fix Unhandled Exception Cannot Find Module Webpack
Additional Resources
- Setting Up a Proxy in Webpack Dev Server