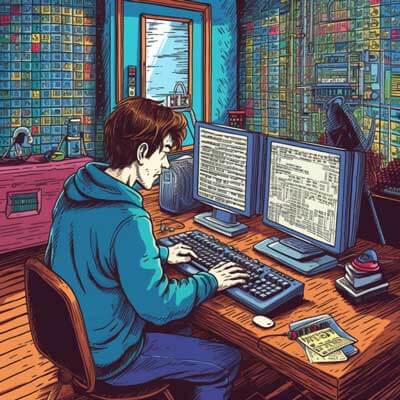
Table of Contents
Overview of Setting Up SCSS Loader
Setting up the SCSS loader in Webpack allows developers to utilize SCSS (Sassy CSS), a popular CSS preprocessor that adds features like variables, nested rules, and mixins. This setup integrates SCSS into the Webpack build process, enabling developers to write more maintainable and scalable stylesheets. It involves installing necessary packages, configuring Webpack, and writing SCSS files that can be processed and bundled for deployment.
Related Article: How to Use the Webpack CLI Option -d
Module Bundlers and Their Importance
Module bundlers serve the purpose of taking various assets and combining them into a single output file or a few files. This is crucial for improving load times, managing dependencies, and optimizing resource usage in web applications. Webpack is a widely used module bundler that handles not only JavaScript but also CSS, images, and other assets, allowing for a modular development approach.
JavaScript Transpilation Explained
Transpilation refers to the process of converting code written in one programming language into another language. In the context of JavaScript, developers often use transpilers like Babel to convert modern JavaScript (ES6+) into a version compatible with older browsers. This ensures that all users can access the application regardless of their browser capabilities. For example, a simple arrow function can be transpiled into a traditional function syntax that older browsers can understand.
Example command to install Babel:
npm install --save-dev @babel/core @babel/preset-env babel-loader
CSS Preprocessing with SCSS
SCSS is a syntax of Sass (Syntactically Awesome Style Sheets) that extends CSS with additional features. It allows nesting of CSS rules, use of variables, and functions, making stylesheets more modular and easier to maintain. For instance, using variables for colors can reduce duplication and help standardize styles across an application.
Example SCSS file:
$primary-color: #3498db;.button { background-color: $primary-color; border: none; color: white; padding: 10px 20px; &:hover { background-color: darken($primary-color, 10%); }}
Related Article: How To Exclude Test Files In Webpack Builds
Enabling Source Maps for Better Debugging
Source maps provide a way to map the compiled code back to the original source code. This is particularly useful for debugging styles, as it allows developers to see the original SCSS code in browser developer tools instead of the compiled CSS. To enable source maps in Webpack, you can set the devtool
property in your Webpack configuration.
Example Webpack configuration:
module.exports = { devtool: 'source-map', // other configurations...};
Hot Module Replacement for SCSS
Hot Module Replacement (HMR) enables live reloading of styles without a full page refresh. When a SCSS file is modified, only the updated styles are injected into the browser. This speeds up development and provides immediate feedback. To set up HMR, you generally need to include the webpack-dev-server
and configure it properly.
Example command to install webpack-dev-server:
npm install --save-dev webpack-dev-server
Example Webpack configuration for HMR:
const webpack = require('webpack');module.exports = { // other configurations... plugins: [ new webpack.HotModuleReplacementPlugin(), ],};
Tree Shaking for Efficient Code Management
Tree shaking is a technique used to eliminate dead code from the final bundle. By analyzing the code during the build process, Webpack can identify parts that are not being used and exclude them from the output. This results in smaller bundle sizes and faster load times. To take advantage of tree shaking, ensure that your code uses ES6 module syntax (import/export).
Example of tree shaking:
// utils.jsexport const unusedFunction = () => { /*...*/ };export const usedFunction = () => { /*...*/ };// main.jsimport { usedFunction } from './utils'; // unusedFunction will be excluded
Code Splitting to Improve Load Times
Code splitting allows developers to break down the application into smaller bundles that can be loaded on demand. This can significantly improve initial load times, as users only download the code they need for the current view. Webpack supports code splitting through dynamic imports.
Example of dynamic import:
function loadComponent() { import('./Component.js').then(module => { const Component = module.default; // Use the component... });}
Related Article: How to Configure Webpack for Expo Projects
Managing Assets in Web Applications
Managing assets such as images, fonts, and stylesheets is crucial for web applications. Webpack provides a systematic way to handle these assets through loaders. Loaders transform files into modules that can be included in your JavaScript. For example, the file-loader
can be used to manage image files.
Example command to install file-loader:
npm install --save-dev file-loader
Example Webpack configuration for file-loader:
module.exports = { module: { rules: [ { test: /\.(png|jpg|gif)$/, use: { loader: 'file-loader', options: { name: '[path][name].[ext]', }, }, }, ], },};
Using npm Scripts for Task Automation
NPM scripts allow developers to automate tasks such as building, testing, and running Webpack. They can be defined in the package.json
file, enabling quick access to common commands. This simplifies the workflow, making it easier to manage different environments.
Example of npm scripts in package.json:
"scripts": { "build": "webpack --mode production", "start": "webpack serve --mode development"}
Essential Configuration for SCSS Loader
To use the SCSS loader with Webpack, specific loaders must be installed and configured in the Webpack setup. The necessary loaders typically include sass-loader
, css-loader
, and style-loader
. The sass-loader
compiles SCSS to CSS, while the css-loader
interprets @import
and url()
statements in CSS, and the style-loader
injects CSS into the DOM.
Example command to install SCSS loaders:
npm install --save-dev sass-loader css-loader style-loader sass
Example Webpack configuration for SCSS loader:
module.exports = { module: { rules: [ { test: /\.scss$/, use: [ 'style-loader', 'css-loader', 'sass-loader' ], }, ], },};
PostCSS Integration with SCSS
PostCSS is a tool that allows developers to transform CSS with JavaScript plugins. Integrating PostCSS with SCSS enables additional processing such as autoprefixing, which automatically adds vendor prefixes to CSS properties. This improves browser compatibility.
Example command to install PostCSS and autoprefixer:
npm install --save-dev postcss-loader autoprefixer
Example Webpack configuration with PostCSS:
module.exports = { module: { rules: [ { test: /\.scss$/, use: [ 'style-loader', 'css-loader', { loader: 'postcss-loader', options: { postcssOptions: { plugins: [ require('autoprefixer') ], }, }, }, 'sass-loader', ], }, ], },};
Related Article: How to Use ESLint Webpack Plugin for Code Quality
Using Babel Loader for JavaScript
Babel loader is essential for transpiling modern JavaScript into a format that is compatible with older browsers. This allows developers to use the latest JavaScript features without worrying about browser support. The Babel loader processes JavaScript files according to the configuration specified in Babel.
Example command to install Babel loader:
npm install --save-dev babel-loader @babel/preset-env
Example Webpack configuration for Babel loader:
module.exports = { module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'], }, }, }, ], },};
Configuring SCSS Loader in Detail
Configuring the SCSS loader involves specifying how Webpack should handle SCSS files. This includes the order of loaders, ensuring styles are injected into the DOM, and processing any dependencies properly. The loaders are defined in a specific order: style-loader
first, followed by css-loader
, and then sass-loader
.
Detailed Webpack configuration:
const path = require('path');module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.scss$/, use: [ 'style-loader', // Injects styles into the DOM 'css-loader', // Translates CSS into CommonJS 'sass-loader', // Compiles Sass to CSS ], }, ], }, devtool: 'source-map', // Enable source maps for debugging};
Differences Between SCSS and CSS
SCSS differs from CSS in several ways. SCSS supports features such as variables, nesting, mixins, and functions, which enhance the capability of writing styles. In contrast, CSS is limited to basic styling without these enhancements. For instance, SCSS allows you to define a color variable and use it throughout the stylesheet, while CSS requires repeating the color code.
Example SCSS with variables:
$base-padding: 10px;.container { padding: $base-padding; .child { padding: $base-padding / 2; }}
Optimizing SCSS Files for Performance
Optimizing SCSS files can reduce file size and improve load times. Techniques include minimizing the use of deep nesting, cleaning up unused styles, and using mixins. Additionally, tools like sass
can be configured to output compressed CSS.
Example command to compile SCSS to compressed CSS:
sass --style=compressed styles.scss styles.css
Related Article: How to Use Extract Text Webpack Plugin
Common Issues with SCSS Loader
Common issues when using the SCSS loader include not installing the required loaders, incorrect loader order, and syntax errors in SCSS files. Developers should ensure all loaders are correctly installed and configured, and check for errors in the SCSS code that may prevent compilation.
Using Multiple Loaders for SCSS
Using multiple loaders for SCSS is common when additional processing is needed. For example, combining sass-loader
, css-loader
, postcss-loader
, and style-loader
allows for comprehensive handling of SCSS files, including autoprefixing and modular loading.
Example Webpack configuration with multiple loaders:
module.exports = { module: { rules: [ { test: /\.scss$/, use: [ 'style-loader', 'css-loader', 'postcss-loader', 'sass-loader', ], }, ], },};
Purpose of the Style Loader in Asset Management
The style loader injects CSS into the DOM by adding a <style>
tag. This is crucial for dynamic styles where the application needs to reflect changes without a full page refresh. The style loader works in conjunction with other loaders to ensure styles are processed and applied correctly.
Importing SCSS Files into JavaScript Modules
Importing SCSS files into JavaScript modules allows developers to encapsulate styles with components. By importing SCSS directly in a JavaScript file, Webpack processes the styles and injects them into the page as part of the build process.
Example of importing SCSS in a JavaScript file:
import './styles.scss';
Related Article: How to Set Webpack Target to Node.js
Recommended Plugins for SCSS Management
Several plugins can enhance SCSS management in Webpack. For example, MiniCssExtractPlugin
can extract CSS into separate files instead of injecting them into the DOM, improving load times for larger applications.
Example command to install MiniCssExtractPlugin:
npm install --save-dev mini-css-extract-plugin
Example Webpack configuration with MiniCssExtractPlugin:
const MiniCssExtractPlugin = require('mini-css-extract-plugin');module.exports = { module: { rules: [ { test: /\.scss$/, use: [ MiniCssExtractPlugin.loader, 'css-loader', 'sass-loader', ], }, ], }, plugins: [ new MiniCssExtractPlugin({ filename: '[name].css', }), ],};
Setting Up a Development Environment with SCSS
Setting up a development environment with SCSS involves configuring Webpack to watch for changes in SCSS files and automatically recompile them. This setup typically includes installing necessary dependencies, configuring loaders, and setting up a local server for testing.
Example command to start the development server:
npm start
Example Webpack configuration for a development environment:
const path = require('path');module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist'), }, module: { rules: [ { test: /\.scss$/, use: [ 'style-loader', 'css-loader', 'sass-loader', ], }, ], }, devServer: { contentBase: path.join(__dirname, 'dist'), compress: true, port: 9000, },};
Additional Resources
- Configuring SCSS Loader in Webpack