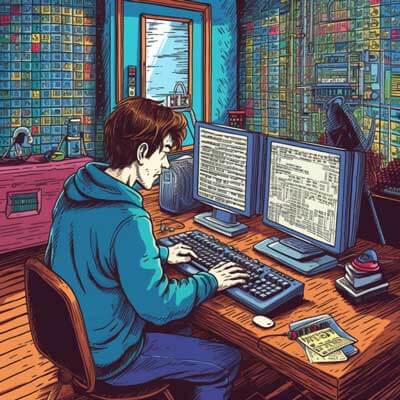
Table of Contents
Overview
Setting the target to Node in Webpack allows developers to create bundles intended to run in a Node.js environment. This is essential for server-side applications, as it ensures that the generated code is compatible with Node.js features and APIs, rather than those of a browser. By default, Webpack targets the browser, which may lead to issues when running code on the server.
Related Article: How to Use Extract Text Webpack Plugin
Configuring Environment for Node Applications
Configuring the environment for Node applications involves ensuring that the necessary dependencies and settings are in place. Developers usually start by creating a package.json
file in the project directory if it doesn't already exist. This file contains metadata about the project and its dependencies.
To initialize a new Node project, run:
npm init -y
Next, install Webpack and its CLI as development dependencies:
npm install --save-dev webpack webpack-cli
The Webpack configuration file, typically named webpack.config.js
, is where the target will be set to Node.
Example of a simple configuration file:
// webpack.config.js module.exports = { target: 'node', entry: './src/index.js', output: { path: __dirname + '/dist', filename: 'bundle.js', }, };
This configuration specifies that the entry point of the application is src/index.js
and the output will be saved in a folder named dist
.
Defining Bundle Size for Node Targets
Bundle size is crucial for performance, especially in Node applications, where larger bundles can lead to longer load times. Developers can analyze the output bundle size using Webpack's built-in tools or plugins.
To define bundle size limits, use the performance
property in the Webpack configuration:
// webpack.config.js module.exports = { // ... other settings performance: { maxAssetSize: 100000, // 100 kb maxEntrypointSize: 300000, // 300 kb }, };
This setting warns you if your assets exceed the specified sizes, helping maintain optimal performance.
Transpilation Settings for Node Code
Transpilation is necessary when using modern JavaScript syntax that may not be supported by all Node versions. Babel is often used for this purpose. First, install Babel and the necessary presets:
npm install --save-dev babel-loader @babel/core @babel/preset-env
After installing, configure Babel in a file named .babelrc
:
{ "presets": ["@babel/preset-env"] }
Then, update the Webpack configuration to use Babel:
// webpack.config.js module.exports = { // ... other settings module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', }, }, ], }, };
This tells Webpack to transpile JavaScript files using Babel, enabling the use of the latest syntax.
Related Article: How to Use ESLint Webpack Plugin for Code Quality
Using CommonJS and ESM in Node
Node.js supports both CommonJS and ECMAScript Modules (ESM). CommonJS uses require
and module.exports
, whereas ESM uses import
and export
.
To use CommonJS:
// CommonJS Module const express = require('express'); module.exports = express();
For ESM, ensure your package.json
has "type": "module"
:
{ "type": "module" }
Then use the ESM syntax:
// ESM Module import express from 'express'; export default express();
Webpack can handle both module types, so choose based on your project requirements.
Implementing Hot Module Replacement in Node
Hot Module Replacement (HMR) allows modules to be updated in real-time without a full reload. For Node applications, HMR can be set up using the webpack-node-externals
package and adding the HMR plugin.
First, install the package:
npm install --save-dev webpack-node-externals
Then, configure Webpack for HMR:
// webpack.config.js const webpack = require('webpack'); const nodeExternals = require('webpack-node-externals'); module.exports = { target: 'node', entry: [ 'webpack/hot/poll?1000', // Poll every second for changes './src/index.js', ], externals: [nodeExternals()], plugins: [ new webpack.HotModuleReplacementPlugin(), ], };
This setup allows for HMR support, making development more dynamic.
Applying Tree Shaking for Node Projects
Tree shaking is a technique to eliminate dead code from the final bundle, reducing its size. To enable it in Node projects, use ES modules and ensure the mode
is set to production
in the Webpack configuration.
Example configuration:
// webpack.config.js module.exports = { mode: 'production', target: 'node', // ... other settings };
Setting Output Directory for Node Builds
The output directory is where the bundled files will be saved after the build process. This can be configured in the output
property of the Webpack configuration.
Example:
// webpack.config.js module.exports = { target: 'node', output: { path: __dirname + '/dist', filename: 'app.js', }, };
In this case, the output will be stored in the dist
folder, and the bundle will be named app.js
.
Related Article: How to Use Django Webpack Loader with Webpack
Choosing Devtool Options for Node Applications
Devtool options affect how source maps are generated, which helps with debugging. For Node applications, consider using cheap-module-source-map
for a balance of speed and usability.
Example configuration:
// webpack.config.js module.exports = { target: 'node', devtool: 'cheap-module-source-map', // ... other settings };
This setting provides a good compromise between build speed and the quality of source maps.
Selecting Loaders for Node Compatibility
Loaders are essential for processing files before they are bundled. Common loaders for Node applications include Babel for JavaScript and CSS loaders for styles.
Example configuration for handling JavaScript and CSS:
// webpack.config.js module.exports = { target: 'node', module: { rules: [ { test: /\.js$/, exclude: /node_modules/, use: 'babel-loader', }, { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, };
This ensures that both JavaScript and CSS files are correctly processed during the build.
Using Plugins for Node Targets
Plugins enhance Webpack's functionality, allowing for additional features like minification, environment variable injection, and more. Common plugins for Node applications include HtmlWebpackPlugin
and WebpackBundleAnalyzer
.
Example of using HtmlWebpackPlugin
:
// webpack.config.js const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { target: 'node', plugins: [ new HtmlWebpackPlugin({ template: './src/index.html', }), ], };
This plugin generates an HTML file that includes the bundled JavaScript, facilitating easier testing and deployment.
Differences Between Target: 'Node' and Target: 'Web'
The key differences between the target: 'node'
and target: 'web'
settings lie in the environment for which the code is optimized.
When targeting Node:
- The output is optimized for Node.js APIs.
- Built-in Node modules are not bundled.
- Code can use Node-specific features like the fs
module.
In contrast, when targeting the web:
- The output is suited for browser APIs.
- The bundle is optimized for performance in a web environment.
- It includes polyfills for browser compatibility.
Choose the target based on where the code will run.
Related Article: How to Configure SVGR with Webpack
Handling Node Modules in Bundles
Node modules can be handled differently based on whether they should be included in the bundle or excluded. Using webpack-node-externals
, developers can exclude Node modules from the bundle, allowing them to be resolved at runtime.
Example configuration:
// webpack.config.js const nodeExternals = require('webpack-node-externals'); module.exports = { target: 'node', externals: [nodeExternals()], };
This setup excludes all node modules, reducing bundle size and allowing for faster startup times.
Using ES6 Syntax with Node Target
Node supports ES6 syntax, but transpilation might be necessary for compatibility with older Node versions. Use Babel to enable ES6 features such as let
, const
, and arrow functions.
The Babel configuration should already be set up as shown previously, allowing the use of modern JavaScript syntax.
Example of using ES6 syntax:
// src/index.js const greet = (name) => `Hello, ${name}!`; console.log(greet('World'));
This code utilizes ES6 features while remaining compatible with the Node environment through Babel transpilation.
Best Practices for Node Configuration
Best practices for configuring Webpack for Node applications include:
- Use target: 'node'
to ensure compatibility with Node.js.
- Keep the bundle size small by excluding unnecessary modules.
- Implement Babel for transpilation to modern syntax.
- Use plugins and loaders appropriately to enhance functionality.
- Regularly analyze bundle size and performance.
Following these practices will lead to a more maintainable and efficient Node application.
Optimizing Bundles for Node Applications
Optimizing bundles involves minimizing size and improving performance. Techniques include:
- Using TerserPlugin
for minification.
- Enabling tree shaking by using ES modules.
- Excluding unnecessary dependencies with webpack-node-externals
.
Example of using TerserPlugin
:
// webpack.config.js const TerserPlugin = require('terser-webpack-plugin'); module.exports = { target: 'node', optimization: { minimize: true, minimizer: [new TerserPlugin()], }, };
This configuration activates minification, reducing the bundle size for production.
Related Article: How to Fix Webpack Command Not Found Error
Commonly Used Plugins for Node Projects
Common plugins for Node projects include:
- HtmlWebpackPlugin
: Simplifies the creation of HTML files.
- TerserPlugin
: Minifies JavaScript files.
- webpack-node-externals
: Excludes Node modules from the bundle.
Each plugin serves a specific purpose, enhancing the development experience and final output.
Debugging Node Applications Built with Bundles
Debugging bundled Node applications can be challenging but using source maps simplifies the process. Ensure that the devtool
option is set to generate source maps.
Example:
// webpack.config.js module.exports = { target: 'node', devtool: 'source-map', // ... other settings };
This allows developers to trace errors back to the original source code instead of the bundled output.
Performance Impact of Target Settings
The chosen target setting impacts performance significantly. Using target: 'node'
optimizes the build for server-side execution, reducing startup times and improving resource management.
Performance can be further enhanced by minimizing the bundle, excluding unused dependencies, and ensuring that the code is transpiled only when necessary.
Including External Libraries in Node Projects
Including external libraries in Node projects can be done by installing the libraries via npm and ensuring they are correctly configured in the Webpack settings.
To install a library, run:
npm install express
Then, require or import the library in the code:
// src/index.js const express = require('express'); const app = express(); app.get('/', (req, res) => res.send('Hello World!')); app.listen(3000, () => console.log('Server running on port 3000'));
This enables the use of external libraries in the Node application, enhancing functionality and capabilities.
Related Article: How to Use Webpack Node Externals
Additional Resources
- Webpack Target: Node vs Web