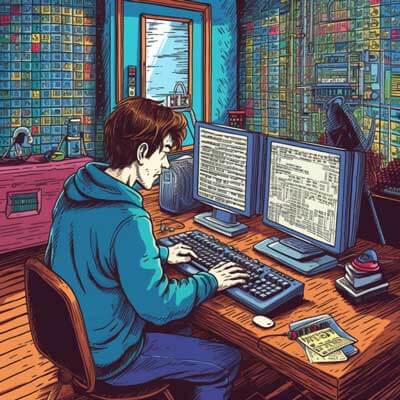
Table of Contents
Introduction
In Node.js, the 'Cannot find module' error occurs when the required module is not found or cannot be located by Node.js. This error commonly happens when there is a mismatch between the module name used in the code and the actual module installed in the system. Fortunately, there are several steps you can take to troubleshoot and resolve this error.
Related Article: How to Switch to an Older Version of Node.js
Possible Solutions
Related Article: AI Implementations in Node.js with TensorFlow.js and NLP
1. Check the Module Name
The first step is to double-check the module name used in your code. Ensure that the module name is spelled correctly and matches the installed module's name exactly. Node.js is case-sensitive, so make sure the capitalization is correct as well.
For example, if you have a line of code like this:
const myModule = require('my-module');
Make sure that 'my-module' matches the name of the installed module exactly. If you're uncertain about the module name, you can check the package.json file or the npm registry for the correct module name.
2. Verify the Module is Installed
If you're certain that the module name is correct, the next step is to verify that the module is installed in your project's dependencies.
You can do this by checking the package.json file in your project's root directory. Look for the 'dependencies' section and ensure that the required module is listed. If it's missing, you can install it using the following command:
npm install module-name
Replace 'module-name' with the actual name of the module you want to install.
3. Check the Module's Installation Location
Sometimes, the 'Cannot find module' error can occur if the module is installed in a different location than expected. This can happen if you have multiple versions of Node.js installed or if you're using a specific version manager like nvm.
To check the installation location of the module, you can use the following command:
npm ls module-name
This command will display the module's installation path. If the path doesn't match the expected location, you may need to update your project's configuration or adjust your environment variables to point to the correct location.
4. Clean the Node.js Cache
Node.js caches modules to improve performance. However, sometimes this cache can become outdated or corrupted, leading to the 'Cannot find module' error. Cleaning the Node.js cache can help resolve this issue.
To clean the cache, use the following command:
npm cache clean --force
This command will clear the entire cache. After cleaning the cache, try running your code again to see if the error persists.
5. Reinstall the Module
If none of the above steps resolve the issue, you can try reinstalling the module. This can help if the module's installation files are corrupted or if the module version is incompatible with your project.
To reinstall the module, use the following command:
npm uninstall module-namenpm install module-name
Replace 'module-name' with the actual name of the module you want to reinstall.
6. Update Node.js and npm
Outdated versions of Node.js and npm can sometimes cause compatibility issues with certain modules. Updating Node.js and npm to the latest versions can help resolve these issues.
To update Node.js, visit the official Node.js website (https://nodejs.org) and download the latest version for your operating system. Follow the installation instructions to update Node.js.
To update npm, you can use the following command:
npm install -g npm@latest
This command will update npm to the latest version globally.
7. Check for Typos or Syntax Errors
Sometimes, the 'Cannot find module' error can be caused by simple typos or syntax errors in your code. Double-check your code for any mistakes, such as missing or extra characters, incorrect file paths, or incorrect import statements.
8. Consult the Module's Documentation or Community
If you're still experiencing the 'Cannot find module' error after trying the above solutions, it can be helpful to consult the module's documentation or community. Many modules have dedicated documentation or community forums where you can find specific troubleshooting steps or ask for assistance.