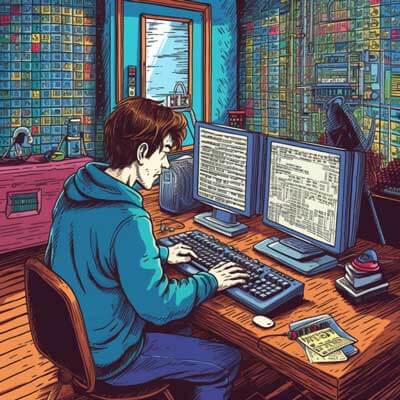
Table of Contents
Sorting a Pandas DataFrame by one column in Python is a common task in data analysis and manipulation. The Pandas library provides several methods to sort a DataFrame based on the values in one or more columns. This answer will guide you through the process step by step.
Method 1: Using the sort_values() method
The most straightforward way to sort a Pandas DataFrame by one column is by using the sort_values()
method. This method allows you to sort the DataFrame based on the values in a specific column.
Here's an example of how to sort a DataFrame named df
by the values in the column named 'column_name' in ascending order:
df_sorted = df.sort_values('column_name')
To sort the DataFrame in descending order, you can pass the argument ascending=False
to the sort_values()
method:
df_sorted = df.sort_values('column_name', ascending=False)
You can also sort the DataFrame by multiple columns by passing a list of column names to the sort_values()
method. The DataFrame will be sorted based on the values in the first column, and if there are any ties, it will be further sorted based on the values in the second column, and so on:
df_sorted = df.sort_values(['column_name1', 'column_name2'])
Related Article: How to Manipulate Strings in Python and Check for Substrings
Method 2: Using the sort_index() method
Another way to sort a Pandas DataFrame by one column is by using the sort_index()
method. This method sorts the DataFrame based on the index values.
To sort the DataFrame by the values in a specific column, you can first use the sort_values()
method to sort the DataFrame by that column, and then use the sort_index()
method to sort it back based on the index:
df_sorted = df.sort_values('column_name').sort_index()
This method is useful when you want to preserve the original order of the DataFrame but still sort it based on a specific column.
Best Practices
Related Article: How to Save and Load Objects Using pickle.load in Python
When sorting a Pandas DataFrame by one column, it's important to keep a few best practices in mind:
1. Make sure the column you want to sort by contains compatible data types. Sorting can be done on numeric, string, or datetime columns, but not on columns with mixed data types.
2. If the column contains missing values, consider how you want to handle them. By default, missing values are placed at the end of the sorted DataFrame. You can change this behavior by using the na_position
parameter in the sort_values()
method.
3. If you want to sort the DataFrame in place, without creating a new sorted DataFrame, you can use the inplace=True
parameter in the sort_values()
or sort_index()
method.
4. To sort the DataFrame based on the index in ascending order, you can use the sort_index()
method without any arguments:
df_sorted = df.sort_index()
To sort the DataFrame based on the index in descending order, you can pass the argument ascending=False
to the sort_index()
method:
df_sorted = df.sort_index(ascending=False)
These best practices will help you sort a Pandas DataFrame by one column effectively and efficiently.