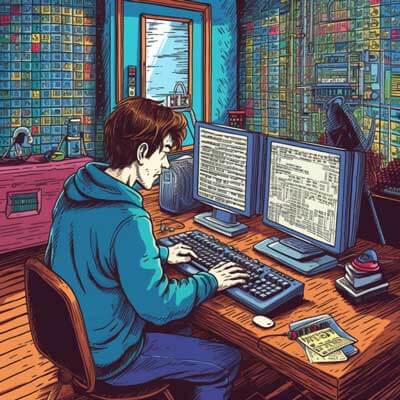
Table of Contents
To sort object properties by their values in JavaScript, you can follow these steps:
Method 1: Using the Object.entries() method and the Array.sort() method
1. Convert the object into an array of key-value pairs using the Object.entries() method.
2. Use the Array.sort() method to sort the array based on the values.
3. Convert the sorted array back into an object using the Object.fromEntries() method.
Here's an example:
const obj = { name: "John", age: 30, city: "New York" }; const sortedObj = Object.fromEntries( Object.entries(obj).sort((a, b) => a[1] - b[1]) ); console.log(sortedObj);
In this example, the obj
variable represents the object that we want to sort. The Object.entries()
method is used to convert the object into an array of key-value pairs. Then, the Array.sort()
method is used to sort the array based on the values. Finally, the Object.fromEntries()
method is used to convert the sorted array back into an object. The sortedObj
variable contains the sorted object.
Related Article: How To Disable & Enable a Submit Button With jQuery
Method 2: Using the Object.keys() method, the Array.sort() method, and the Array.reduce() method
1. Get the keys of the object using the Object.keys() method.
2. Sort the keys array based on the values using the Array.sort() method and a custom comparison function.
3. Use the Array.reduce() method to construct a new object with the sorted keys and their corresponding values.
Here's an example:
const obj = { name: "John", age: 30, city: "New York" }; const sortedObj = Object.keys(obj) .sort((a, b) => obj[a] - obj[b]) .reduce((acc, key) => { acc[key] = obj[key]; return acc; }, {}); console.log(sortedObj);
In this example, the obj
variable represents the object that we want to sort. The Object.keys()
method is used to get the keys of the object. Then, the Array.sort()
method is used to sort the keys based on the values, using a custom comparison function. Finally, the Array.reduce()
method is used to construct a new object with the sorted keys and their corresponding values. The sortedObj
variable contains the sorted object.
Alternative Ideas:
1. If you want to sort the object in descending order, you can modify the comparison function to b[1] - a[1]
or obj[b] - obj[a]
in the first and second methods respectively.
2. If you have an array of objects instead of a single object, you can apply the same sorting techniques by iterating over the array and applying the sorting methods to each object individually.
Best Practices:
1. When using the Array.sort()
method, it's important to provide a custom comparison function that compares the values correctly. The default comparison function converts the values to strings and performs a lexicographic comparison, which may not give the desired results when sorting numbers.
2. If the object contains non-numeric values or complex data types like arrays or objects, the sorting methods mentioned above may not work as expected. In such cases, you may need to provide a more specific and customized comparison function to handle the sorting logic for those data types.
3. It's a good practice to store the sorted object in a new variable to avoid modifying the original object if it needs to be preserved.
4. If you are working with large objects or performance is a concern, consider using more optimized sorting algorithms, such as quicksort or mergesort, instead of the default sorting algorithm provided by Array.sort()
. These algorithms have better average-case time complexity and can improve the sorting performance.
Now you know how to sort object properties by their values in JavaScript using different methods. Apply these techniques based on your specific use case and requirements to achieve the desired sorting behavior.